Leetcode 2208: Minimum Operations to Halve Array Sum
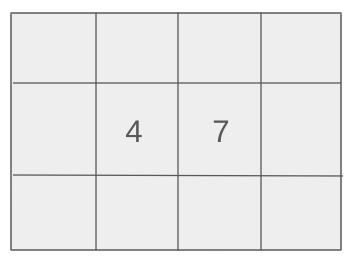
You are given an array nums of positive integers. In each operation, you can reduce any number in the array by half. Your goal is to return the minimum number of operations required to reduce the sum of the array by at least half.
Problem
Approach
Steps
Complexity
Input: The input consists of an array nums containing positive integers.
Example: nums = [5, 19, 8, 1]
Constraints:
• 1 <= nums.length <= 10^5
• 1 <= nums[i] <= 10^7
Output: Return the minimum number of operations required to reduce the sum of nums by at least half.
Example: For input nums = [5, 19, 8, 1], the output is 3.
Constraints:
• The output should be an integer.
Goal: Minimize the number of operations to reduce the sum of nums by at least half.
Steps:
• Calculate the initial sum of the array.
• Use a max-heap to repeatedly reduce the largest number in the array by half.
• Keep track of the number of operations until the sum is reduced by at least half.
Goal: The length of the array and the values of the elements are constrained by the given limits.
Steps:
• 1 <= nums.length <= 10^5
• 1 <= nums[i] <= 10^7
Assumptions:
• The input array nums is not empty.
• All elements in nums are positive integers.
• Input: nums = [5, 19, 8, 1]
• Explanation: By reducing 19 to 9, then 9 to 4, and 8 to 4, we reduce the sum by at least half, using 3 operations.
• Input: nums = [3, 8, 20]
• Explanation: By reducing 20 to 10, then 10 to 5, and 3 to 1.5, we reduce the sum by at least half, using 3 operations.
Approach: To solve this problem, we need to repeatedly reduce the largest elements in the array until the sum is reduced by at least half. A greedy approach using a max-heap allows us to efficiently pick the largest number to halve.
Observations:
• The larger the number we reduce, the more significant the reduction in the total sum.
• We can use a max-heap to always halve the largest element and track the number of operations.
Steps:
• Calculate the sum of the array.
• Insert all elements into a max-heap (using negative values to simulate max behavior in a min-heap).
• Repeat halving the largest element until the sum is reduced by at least half, counting the number of operations.
Empty Inputs:
• The array cannot be empty, as the input is constrained to contain at least one number.
Large Inputs:
• For large arrays, efficient use of heaps and careful handling of large values is required.
Special Values:
• If all elements are very small compared to others, fewer operations will be needed.
Constraints:
• The algorithm should handle input sizes up to 10^5 efficiently.
int halveArray(vector<int>& nums) {
int n = nums.size();
double sum = 0;
priority_queue<double, vector<double>, less<double>> pq;
for(int i = 0; i < n; i++){
pq.push(nums[i]);
sum += nums[i];
}
sum = sum / 2;
double tmp = 0;
int cnt = 0;
while(tmp < sum && !pq.empty()) {
double x = (pq.top() / 2);
tmp += x;
pq.pop();
pq.push(x);
cnt++;
}
return cnt;
}
1 : Variable Initialization
int halveArray(vector<int>& nums) {
Function declaration with the input parameter 'nums', a vector of integers.
2 : Size Calculation
int n = nums.size();
Calculate the size of the input array to iterate over all elements.
3 : Sum Initialization
double sum = 0;
Initialize the sum variable to accumulate the total sum of the array elements.
4 : Priority Queue
priority_queue<double, vector<double>, less<double>> pq;
A max-heap priority queue is initialized to store the elements of the array for efficient access to the largest element.
5 : Iteration Start
for(int i = 0; i < n; i++){
Loop over all the elements of the array to populate the priority queue and calculate the total sum.
6 : Push to Queue
pq.push(nums[i]);
Push each element of the array into the priority queue.
7 : Sum Update
sum += nums[i];
Accumulate the sum of the array elements.
8 : Calculate Half
sum = sum / 2;
Set the target sum to half of the total sum of the array.
9 : Temporary Sum Initialization
double tmp = 0;
Initialize the temporary sum to track the running total of the halved elements.
10 : Counter Initialization
int cnt = 0;
Initialize a counter variable to count how many operations (halving steps) are performed.
11 : Loop Start
while(tmp < sum && !pq.empty()) {
Start a loop that continues until the temporary sum exceeds the target or the priority queue is empty.
12 : Find Largest Element
double x = (pq.top() / 2);
Retrieve the largest element from the priority queue and halve it.
13 : Update Temporary Sum
tmp += x;
Add the halved value to the temporary sum.
14 : Pop and Push
pq.pop();
Remove the top element (largest element) from the priority queue.
15 : Reinsert Halved Value
pq.push(x);
Push the halved value back into the priority queue for further processing.
16 : Increment Counter
cnt++;
Increment the counter to track the number of operations performed.
17 : Return Result
return cnt;
Return the count of operations performed to halve the array to the target sum.
Best Case: O(n log n)
Average Case: O(n log n)
Worst Case: O(n log n)
Description: The time complexity is O(n log n) due to the heap operations for each element in the array.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) due to the storage of the elements in the heap.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus