Leetcode 2180: Count Integers With Even Digit Sum
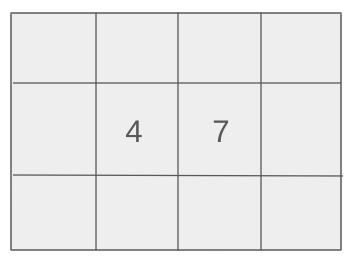
Given a positive integer
num
, return the number of positive integers less than or equal to num
whose digit sum is even.Problem
Approach
Steps
Complexity
Input: The input consists of a single integer `num`.
Example: 7
Constraints:
• 1 <= num <= 1000
Output: The output is the number of positive integers less than or equal to `num` whose digit sum is even.
Example: 3
Constraints:
• The digit sum is calculated by summing all the digits of a number.
Goal: The goal is to count how many numbers from 1 to `num` have an even digit sum.
Steps:
• For each number from 1 to `num`, calculate the sum of its digits.
• If the sum of the digits is even, increment the count.
Goal: The input `num` should be within the given bounds.
Steps:
• 1 <= num <= 1000
Assumptions:
• The number `num` is positive and within the given range.
• Input: 7
• Explanation: The integers less than or equal to 7 whose digit sum is even are 2, 4, and 6.
Approach: The approach involves checking the digit sum of each integer from 1 to `num` and counting how many have an even digit sum.
Observations:
• We can easily calculate the sum of digits by iterating over the digits of a number.
• Since we are iterating over the numbers up to `num`, the time complexity will be proportional to the size of `num`.
Steps:
• Iterate over all integers from 1 to `num`.
• For each number, calculate the sum of its digits.
• Check if the sum is even and keep a count of such numbers.
Empty Inputs:
• If `num` is 1, the result is 0 since there are no numbers with even digit sums less than 1.
Large Inputs:
• For large values of `num`, the solution should handle them efficiently within the given constraints.
Special Values:
• The edge case where `num` is the smallest value, 1, should be handled separately.
Constraints:
• Ensure the solution works efficiently for `num` values up to 1000.
int countEven(int num) {
int temp = num, sum = 0;
while (num > 0) {
sum += num % 10;
num /= 10;
}
return sum % 2 == 0 ? temp / 2 : (temp - 1) / 2;
}
1 : Function Definition
int countEven(int num) {
Define the function `countEven` that takes an integer `num` and returns an integer result based on the sum of the digits.
2 : Variable Initialization
int temp = num, sum = 0;
Initialize two variables: `temp` stores the original value of `num`, and `sum` accumulates the sum of the digits of `num`.
3 : Loop
while (num > 0) {
Start a while loop that continues until `num` becomes 0.
4 : Sum Calculation
sum += num % 10;
Add the last digit of `num` (obtained using `num % 10`) to `sum`.
5 : Update Number
num /= 10;
Remove the last digit from `num` by dividing it by 10.
6 : Return Statement
return sum % 2 == 0 ? temp / 2 : (temp - 1) / 2;
Check if the sum of digits (`sum`) is even. If true, return `temp / 2`; otherwise, return `(temp - 1) / 2`.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is linear in terms of the input size (`num`).
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is constant since the solution only requires a fixed amount of extra space.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus