Leetcode 2171: Removing Minimum Number of Magic Beans
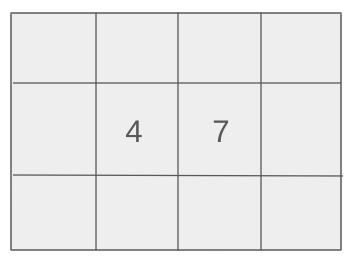
You are given an array of positive integers representing the number of magic beans in each bag. Your task is to remove some beans (possibly none) from each bag so that the number of beans in each remaining non-empty bag is equal. You need to find the minimum number of beans to remove.
Problem
Approach
Steps
Complexity
Input: The input consists of an array of integers, where each integer represents the number of beans in a particular magic bag.
Example: [8, 3, 7, 5]
Constraints:
• 1 <= beans.length <= 10^5
• 1 <= beans[i] <= 10^5
Output: The output should be a single integer representing the minimum number of beans that need to be removed.
Example: 7
Constraints:
• The output must be an integer value representing the minimum beans to be removed.
Goal: The goal is to remove the minimum number of beans while ensuring that all remaining non-empty bags have the same number of beans.
Steps:
• Calculate the sum of all beans in the array.
• Sort the array to facilitate efficient calculation of removals.
• For each possible number of beans to keep in each bag, compute the total removals required, and track the minimum removals.
Goal: The input has constraints on the length of the array and the size of the integers in the array.
Steps:
• 1 <= beans.length <= 10^5
• 1 <= beans[i] <= 10^5
Assumptions:
• The number of bags (beans.length) is at least 1.
• The integers representing the beans in each bag are positive.
• Input: [8, 3, 7, 5]
• Explanation: In this example, the optimal solution is to remove 7 beans in total by making the bags have 3 beans each.
Approach: The approach is to calculate the total sum of beans and explore the different possible configurations of bags with equal numbers of beans.
Observations:
• Sorting the array allows efficient calculation of the total number of beans to be removed.
• The problem involves iterating over the bags to find the most efficient way of removing beans.
Steps:
• Calculate the sum of all the beans in the array.
• Sort the array to identify the optimal target number of beans per bag.
• For each possible number of beans to keep, calculate the total number of removals needed.
Empty Inputs:
• The array will not be empty as per the constraints.
Large Inputs:
• Ensure the solution handles large arrays efficiently with lengths up to 10^5.
Special Values:
• Consider cases where all the bags contain the same number of beans.
Constraints:
• Ensure the solution works within the provided input constraints.
long long minimumRemoval(vector<int>& beans) {
int n = beans.size();
long long sum = accumulate(begin(beans), end(beans), 0L);
sort(beans.begin(), beans.end());
long long res = LLONG_MAX;
for (int i = 0; i < n; i++)
res = min(res, sum - (long long) (n - i) * beans[i]);
return res;
}
1 : Function Definition
long long minimumRemoval(vector<int>& beans) {
Define the function `minimumRemoval`, which takes a vector of integers `beans` and returns a long long integer. This function aims to calculate the minimum beans that need to be removed.
2 : Variable Initialization
int n = beans.size();
Initialize the variable `n` to the size of the `beans` vector, representing the total number of elements in the vector.
3 : Sum Calculation
long long sum = accumulate(begin(beans), end(beans), 0L);
Use the `accumulate` function to calculate the total sum of the `beans` vector. The initial value of the sum is 0L, and the result is stored in `sum`.
4 : Sorting
sort(beans.begin(), beans.end());
Sort the `beans` vector in ascending order. Sorting helps in calculating the minimum removals efficiently.
5 : Variable Initialization
long long res = LLONG_MAX;
Initialize the variable `res` to `LLONG_MAX`, representing the minimum removal result, which will be updated during the iteration.
6 : Loop Start
for (int i = 0; i < n; i++)
Start a loop that iterates through each element in the `beans` vector. The variable `i` tracks the current index.
7 : Update Minimum Removal
res = min(res, sum - (long long) (n - i) * beans[i]);
For each iteration, update `res` with the minimum value between the current `res` and the difference between `sum` and the calculated removal cost for the current index `i`.
8 : Return Result
return res;
Return the final result, which is the minimum number of beans that need to be removed.
Best Case: O(n log n)
Average Case: O(n log n)
Worst Case: O(n log n)
Description: The time complexity is dominated by the sorting step, which takes O(n log n).
Best Case: O(1)
Worst Case: O(n)
Description: The space complexity is O(n) due to the space required for sorting the array.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus