Leetcode 2169: Count Operations to Obtain Zero
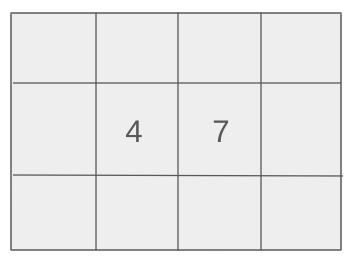
You are given two non-negative integers, num1 and num2. In each operation, subtract the smaller number from the larger one. Continue until one of the numbers becomes zero and return the number of operations performed.
Problem
Approach
Steps
Complexity
Input: The input consists of two integers, num1 and num2, which represent the two numbers for which the operations are to be performed.
Example: [4, 5]
Constraints:
• 0 <= num1, num2 <= 10^5
Output: The output should be a single integer, representing the total number of operations needed to make either num1 or num2 equal to zero.
Example: 4
Constraints:
• At least one of num1 or num2 will become zero after performing the operations.
Goal: The goal is to keep performing the subtraction operation on the two numbers until one of them becomes zero.
Steps:
• Perform subtraction on the two numbers until either num1 or num2 becomes zero.
• Count the number of operations required.
Goal: The problem has constraints on the values of num1 and num2.
Steps:
• 0 <= num1, num2 <= 10^5
Assumptions:
• Both num1 and num2 are non-negative integers.
• Input: [4, 5]
• Explanation: In this example, the subtraction process is performed step by step until num1 becomes zero. The total number of steps is 4.
Approach: The approach is to repeatedly subtract the smaller number from the larger one and keep track of the number of operations. The process continues until one number becomes zero.
Observations:
• The subtraction operation is straightforward, and the number of operations depends on the difference between the two numbers.
• We need to keep track of how many times the subtraction operation is performed until one of the numbers reaches zero.
Steps:
• Initialize a counter for the number of operations.
• Perform the subtraction operation repeatedly until one of the numbers becomes zero.
• Return the counter as the result.
Empty Inputs:
• The input will not be empty as both num1 and num2 must be non-negative integers.
Large Inputs:
• The solution must handle cases where num1 and num2 are large, up to 100,000.
Special Values:
• If num1 or num2 is zero at the start, the number of operations is zero.
Constraints:
• Ensure the solution works efficiently for large inputs.
int countOperations(int num1, int num2) {
int ans = 0;
while(num1 > 0 && num2 > 0) {
if(num1 > num2) {
num1 -= num2;
} else {
num2 -= num1;
}
ans++;
}
return ans;
}
1 : Method Definition
int countOperations(int num1, int num2) {
This is the method definition for `countOperations`, which takes two integer arguments `num1` and `num2`.
2 : Variable Initialization
int ans = 0;
The variable `ans` is initialized to 0. It will store the number of operations performed.
3 : While Loop
while(num1 > 0 && num2 > 0) {
The `while` loop continues as long as both `num1` and `num2` are greater than 0.
4 : If Condition
if(num1 > num2) {
If `num1` is greater than `num2`, the following operation is executed.
5 : Subtraction Operation
num1 -= num2;
This operation subtracts `num2` from `num1`.
6 : Else Condition
} else {
If the `if` condition is not met, the following `else` block is executed.
7 : Subtraction Operation
num2 -= num1;
This operation subtracts `num1` from `num2`.
8 : Increment Operation
ans++;
The `ans` variable is incremented by 1, representing one operation completed.
9 : Return Statement
return ans;
The method returns the value of `ans`, which is the number of operations performed.
Best Case: O(1)
Average Case: O(log(max(num1, num2)))
Worst Case: O(max(num1, num2))
Description: Each operation reduces one of the numbers, and the time complexity is logarithmic in the best case, linear in the worst case.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is constant as we are only storing a few variables.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus