Leetcode 2160: Minimum Sum of Four Digit Number After Splitting Digits
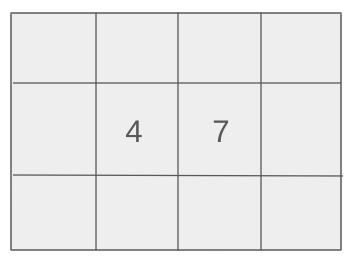
You are given a four-digit integer ’num’. Your task is to split this number into two new integers, ’new1’ and ’new2’, by rearranging its digits. The digits in ’num’ must be used in their entirety, and leading zeros are allowed in ’new1’ and ’new2’. Your goal is to return the minimum possible sum of ’new1’ and ’new2'.
Problem
Approach
Steps
Complexity
Input: The input consists of a single integer 'num', which is a four-digit number.
Example: num = 4312
Constraints:
• 1000 <= num <= 9999
Output: The output should be the minimum possible sum of the two integers formed by rearranging the digits of 'num'.
Example: 45
Constraints:
• The sum of the two integers formed should be the minimum possible sum.
Goal: Rearrange the digits of 'num' to form two integers, and calculate their sum to minimize the result.
Steps:
• Convert the integer 'num' to a string to access its digits.
• Sort the digits in ascending order.
• Form two integers by taking alternate digits from the sorted list and summing them.
• Return the sum of the two integers.
Goal: The input integer 'num' is a four-digit integer.
Steps:
• 1000 <= num <= 9999
Assumptions:
• The integer 'num' is a positive four-digit number.
• Input: Example 1: num = 4312
• Explanation: By rearranging the digits of '4312', we can get pairs like [13, 42], [14, 32], [12, 43]. The smallest sum is obtained by the pair [13, 32], and their sum is 45.
• Input: Example 2: num = 9503
• Explanation: By rearranging the digits of '9503', we can get pairs such as [03, 95], [05, 93], [9, 503]. The pair [03, 95] yields the smallest sum of 38.
Approach: The approach is to sort the digits of 'num' in ascending order, then form two integers by taking alternate digits. This ensures that the sum of the two integers is minimized.
Observations:
• Sorting the digits ensures that we use the smallest available digits first, minimizing the sum.
• After sorting, alternating the digits between the two integers minimizes the sum of the two numbers.
Steps:
• Convert 'num' to a string to access the digits.
• Sort the digits in ascending order.
• Use the first two digits for one number and the last two digits for the other.
• Sum the two numbers and return the result.
Empty Inputs:
• The input will always be a four-digit number, so no special handling for empty inputs is required.
Large Inputs:
• As the input is always four digits, there is no need to handle extremely large inputs.
Special Values:
• The input will never have more than four digits, and the result will always be a valid sum.
Constraints:
• Ensure that the solution handles inputs within the range of 1000 to 9999 correctly.
int minimumSum(int num){
string s = to_string(num);
sort(s.begin(), s.end());
int res = (s[0] - '0' + s[1] - '0') * 10 + s[2] - '0' + s[3] - '0';
return res;
}
1 : Function Definition
int minimumSum(int num){
Define a function `minimumSum` that accepts a single integer `num` as an argument.
2 : String Conversion
string s = to_string(num);
Convert the integer `num` to a string `s` for easy manipulation of individual digits.
3 : Sorting
sort(s.begin(), s.end());
Sort the string `s` in ascending order to arrange the digits in increasing order.
4 : Computation
int res = (s[0] - '0' + s[1] - '0') * 10 + s[2] - '0' + s[3] - '0';
Compute the minimum sum by forming two numbers from the sorted digits and summing them.
5 : Return
return res;
Return the computed result, which is the minimum sum of the two numbers.
Best Case: O(1)
Average Case: O(1)
Worst Case: O(1)
Description: The time complexity is constant because the input size is always fixed at four digits.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is constant since we only need space to store the digits of the four-digit number.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus