Leetcode 2148: Count Elements With Strictly Smaller and Greater Elements
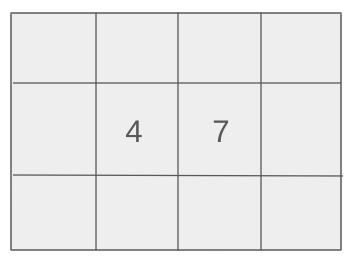
You are given an integer array ’nums’. Your task is to return the count of elements in the array that have both a strictly smaller and a strictly greater number in the array. Each element should have at least one number strictly smaller and one number strictly greater.
Problem
Approach
Steps
Complexity
Input: The input consists of a single integer array 'nums'.
Example: nums = [5, 8, 1, 10]
Constraints:
• 1 <= nums.length <= 100
• -105 <= nums[i] <= 105
Output: The output is a single integer representing the count of elements in the array that satisfy the given condition.
Example: Output: 2
Constraints:
• The result should be a non-negative integer.
Goal: We aim to count the number of elements in 'nums' that have both a strictly smaller and a strictly greater element.
Steps:
• Sort the array to easily compare neighboring elements.
• Identify the range where there are both smaller and larger elements than the current element.
• Count elements that satisfy the condition.
Goal: The length of the input array must be between 1 and 100, and each element of the array must be between -105 and 105.
Steps:
• 1 <= nums.length <= 100
• -105 <= nums[i] <= 105
Assumptions:
• The input array contains at least one element.
• The array may contain duplicate elements.
• Input: Example 1: nums = [5, 8, 1, 10]
• Explanation: The element '8' has both a smaller element '1' and a greater element '10'. Similarly, '5' has a smaller element '1' and a greater element '8'. Thus, two elements satisfy the condition.
Approach: We can solve this problem by sorting the array and then finding elements that have both smaller and greater elements in the sorted array.
Observations:
• Sorting the array helps in easily finding elements that have both smaller and larger neighbors.
• We need to traverse the sorted array and count the elements that meet the criteria.
Steps:
• Sort the array in non-decreasing order.
• Iterate through the array to check for elements that have both a smaller and a greater element.
• Count the number of such elements and return the result.
Empty Inputs:
• An empty array is not valid per the constraints.
Large Inputs:
• The solution should handle the maximum size of the input array efficiently.
Special Values:
• Arrays with all identical elements should return 0.
Constraints:
• Ensure that the array is sorted before making comparisons.
int countElements(vector<int>& nums) {
sort(nums.begin(), nums.end());
int res = 0, l = -1, r = -1, n = nums.size();
for(int i = 1; i < n; i++) {
if(nums[i] > nums[i - 1]) {
l = i;
break;
}
}
for(int i = n - 1; i > 0; i--) {
if(nums[i] > nums[i - 1]) {
r = i;
break;
}
}
if(l < r) return r - l;
return 0;
}
1 : Function Start
int countElements(vector<int>& nums) {
The function begins. The input vector `nums` is passed by reference.
2 : Sort Array
sort(nums.begin(), nums.end());
Sort the input array to prepare for identifying the unsorted segment.
3 : Variable Initialization
int res = 0, l = -1, r = -1, n = nums.size();
Initialize variables: `res` (result), `l` (left index), `r` (right index), and `n` (size of the array).
4 : Forward Iteration
for(int i = 1; i < n; i++) {
Start a loop to iterate from the second element to the end of the sorted array.
5 : Check Left Bound
if(nums[i] > nums[i - 1]) {
Check if the current element is greater than the previous one, identifying the first unordered pair.
6 : Set Left Index
l = i;
Set the left index `l` to the current position where the first unordered element is found.
7 : Break Loop
break;
Break out of the loop once the left boundary is identified.
8 : Backward Iteration
for(int i = n - 1; i > 0; i--) {
Start a loop to iterate from the last element to the first in reverse order.
9 : Check Right Bound
if(nums[i] > nums[i - 1]) {
Check if the current element is greater than the previous one, identifying the second unordered pair.
10 : Set Right Index
r = i;
Set the right index `r` to the current position where the second unordered element is found.
11 : Break Loop
break;
Break out of the loop once the right boundary is identified.
12 : Check Unsorted Segment
if(l < r) return r - l;
If the left index `l` is less than the right index `r`, return the difference between them as the length of the unsorted segment.
13 : Return Result
return 0;
Return 0 if no unsorted segment was found.
Best Case: O(n log n)
Average Case: O(n log n)
Worst Case: O(n log n)
Description: The time complexity is determined by the sorting step, which takes O(n log n).
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is constant since we only use a few variables for counting and comparisons.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus