Leetcode 2134: Minimum Swaps to Group All 1's Together II
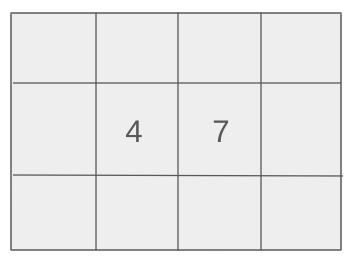
You are given a binary circular array, where each element is either 0 or 1. The goal is to group all the 1’s together in the array using the minimum number of swaps. A swap consists of exchanging the values at two distinct positions in the array. The array is circular, meaning the first and last elements are adjacent. Your task is to find the minimum number of swaps required to group all the 1’s together.
Problem
Approach
Steps
Complexity
Input: The input is a binary array, which is circular in nature, represented as a list of integers where each element is either 0 or 1.
Example: nums = [1, 0, 1, 1, 0, 1, 0]
Constraints:
• 1 <= nums.length <= 10^5
• nums[i] is either 0 or 1
Output: Return an integer representing the minimum number of swaps required to group all the 1's together in the array.
Example: Input: nums = [1, 0, 1, 1, 0, 1, 0] Output: 1
Constraints:
• The solution must be optimal and efficient for large arrays.
Goal: The goal is to minimize the number of swaps required to group all the 1's together in a circular array.
Steps:
• 1. Count the total number of 1's in the array.
• 2. Create a doubled array to account for the circular nature of the array.
• 3. Use a sliding window of size equal to the number of 1's to count how many 1's are within each window.
• 4. Find the window with the maximum number of 1's and calculate the number of swaps needed to move the remaining 1's into that window.
Goal: The problem requires finding the minimum number of swaps efficiently, especially for large input sizes.
Steps:
• The input array length is between 1 and 10^5.
• Each element in the array is either 0 or 1.
Assumptions:
• The input array will always contain at least one element.
• We assume that the input array can be quite large (up to 10^5 elements).
• Input: Input: nums = [1, 0, 1, 1, 0, 1, 0]
• Explanation: In this case, the number of 1's is 4. The window of size 4 can be placed in several positions. The window containing the last 4 elements, [1, 1, 0, 1], has 3 ones. We can move one more 1 into this window with just 1 swap, thus the minimum number of swaps is 1.
• Input: Input: nums = [0, 1, 1, 1, 0, 0, 1, 1, 0]
• Explanation: Here, there are 5 ones in the array. By applying the sliding window technique, we can find that the minimum swaps required to group all 1's together is 2.
• Input: Input: nums = [1, 1, 0, 0, 1]
• Explanation: All the 1's are already grouped together due to the circular property of the array. Thus, no swaps are needed.
Approach: The approach involves using a sliding window to efficiently group the 1's together while accounting for the circular nature of the array.
Observations:
• A direct approach would involve trying all possible combinations of 1's, but that would be inefficient for large arrays.
• A sliding window is a more optimal way to find the smallest number of swaps needed.
• The key idea is to find the largest window containing the most 1's, then count how many swaps are needed to bring the remaining 1's into this window.
Steps:
• 1. Count the total number of 1's in the array.
• 2. Create a doubled array to handle the circular structure.
• 3. Use a sliding window of size equal to the number of 1's.
• 4. For each window, count how many 1's are inside it and calculate the number of swaps needed.
• 5. Return the minimum number of swaps from all the windows.
Empty Inputs:
• An empty array is not a valid input as per the problem constraints.
Large Inputs:
• The solution must handle input arrays of size up to 100,000 efficiently.
Special Values:
• Arrays with all 1's or all 0's should be handled as special cases, as no swaps are needed in these cases.
Constraints:
• The solution should be optimized to run in linear time, O(n), given the constraint that n can be as large as 100,000.
int minSwaps(vector<int>& nums) {
int ones = 0, x = 0, onesInWindows = 0, i = 0, n = nums.size();
ones = count(nums.begin(), nums.end(), 1);
vector<int> nums2(2 *n);
for(int i = 0; i < 2 * n; i++)
nums2[i] = nums[i%n];
for(int i = 0; i < 2 *n ; i++) {
if(i >= ones && nums2[i - ones] == 1) x--;
if(nums2[i] == 1) x++;
onesInWindows = max(x, onesInWindows);
}
return ones - onesInWindows;
}
1 : Function Declaration
int minSwaps(vector<int>& nums) {
The function `minSwaps` takes a reference to a vector of integers `nums`, representing a binary array, and returns the minimum number of swaps needed to group all the 1's together.
2 : Variable Declaration
int ones = 0, x = 0, onesInWindows = 0, i = 0, n = nums.size();
This line declares and initializes the variables: `ones` to count the number of 1's, `x` for the current count of 1's in the window, `onesInWindows` for the maximum number of 1's in the window, `i` as the loop counter, and `n` to store the size of the input array `nums`.
3 : Operation
ones = count(nums.begin(), nums.end(), 1);
This line counts the total number of 1's in the array `nums` using the `count` function and stores it in `ones`.
4 : Vector Initialization
vector<int> nums2(2 *n);
A new vector `nums2` of size `2*n` is created to simulate a circular array by repeating the original array `nums`.
5 : Loop
for(int i = 0; i < 2 * n; i++)
A loop to populate the `nums2` vector with the elements of `nums` repeated twice. This helps to simulate the circular nature of the array.
6 : Operation
nums2[i] = nums[i%n];
Each element of `nums2` is assigned the corresponding element from `nums`, using the modulus operation to ensure it wraps around when `i` exceeds the length of `nums`.
7 : Loop
for(int i = 0; i < 2 *n ; i++) {
This loop iterates through the `nums2` array to calculate the number of 1's in the sliding window of size `ones`.
8 : Condition
if(i >= ones && nums2[i - ones] == 1) x--;
If the current index `i` is greater than or equal to the window size `ones`, and the element at position `i - ones` is a 1, we decrement `x` as it falls out of the window.
9 : Condition
if(nums2[i] == 1) x++;
If the current element `nums2[i]` is 1, we increment `x`, as it is part of the current window.
10 : Operation
onesInWindows = max(x, onesInWindows);
This updates `onesInWindows` to the maximum value between `x` (the current count of 1's in the window) and the previous value of `onesInWindows`.
11 : Return
return ones - onesInWindows;
The function returns the difference between `ones` (total number of 1's) and `onesInWindows` (the maximum number of 1's found in the sliding window), which is the minimum number of swaps needed.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n) due to the sliding window approach and the linear traversal of the array.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) due to the need for a doubled array to handle the circular nature of the input.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus