Leetcode 2104: Sum of Subarray Ranges
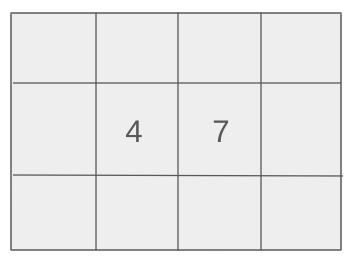
You are given an integer array nums
. A subarray of nums
is a contiguous sequence of elements. The range of a subarray is defined as the difference between the maximum and minimum elements within that subarray.
Your task is to calculate the sum of the ranges for all possible subarrays in the given array nums
.
Problem
Approach
Steps
Complexity
Input: The input is a 1D array of integers `nums` of length n, where each element nums[i] represents an integer in the array.
Example: nums = [1, 2, 3]
Constraints:
• 1 <= nums.length <= 1000
• -10^9 <= nums[i] <= 10^9
Output: Return the sum of all subarray ranges of `nums`.
Example: Output: 4
Constraints:
Goal: The goal is to calculate the sum of all subarray ranges efficiently.
Steps:
• Iterate over each subarray of nums.
• For each subarray, find the minimum and maximum values.
• Calculate the range of the subarray as the difference between the maximum and minimum values.
• Sum up the ranges for all subarrays.
Goal: The input array nums has a length of at most 1000 and each element is an integer in the range [-10^9, 10^9].
Steps:
• 1 <= nums.length <= 1000
• -10^9 <= nums[i] <= 10^9
Assumptions:
• You are given a valid array nums.
• The input is non-empty.
• Input: Input: nums = [1, 2, 3]
• Explanation: The subarrays are [1], [2], [3], [1, 2], [2, 3], and [1, 2, 3]. The ranges of these subarrays are 0, 0, 0, 1, 1, and 2, respectively. The sum of these ranges is 0 + 0 + 0 + 1 + 1 + 2 = 4.
Approach: To solve the problem efficiently, we will calculate the range for each subarray and sum them up. An optimal approach may involve iterating over each subarray in a nested loop and calculating its range.
Observations:
• The brute-force solution is to find the range for every subarray by iterating through the entire array for each subarray, which is O(n^2).
• We should try to optimize this solution, possibly using a sliding window or other techniques to calculate the range more efficiently.
Steps:
• Iterate over each element of the array to consider it as the starting point of a subarray.
• For each subarray, track the minimum and maximum values as you extend the subarray.
• Add the difference (max - min) to the result for each subarray.
Empty Inputs:
• The input will always have at least one element.
Large Inputs:
• The approach should be efficient enough to handle arrays up to the maximum length of 1000.
Special Values:
• Arrays with all identical elements will have zero range for all subarrays.
Constraints:
• The algorithm should work within the time limit for n up to 1000.
long long subArrayRanges(vector<int>& nums) {
long long res = 0, n = nums.size();
for(int i = 0; i < n - 1; i++) {
int mini = nums[i], maxi = nums[i];
for(int j = i + 1; j < n; j++) {
if(nums[j] < mini) mini = nums[j];
if(nums[j] > maxi) maxi = nums[j];
res += maxi - mini;
}
}
return res;
}
1 : Function Declaration
long long subArrayRanges(vector<int>& nums) {
Declares the function that computes the sum of the ranges (max - min) of all subarrays.
2 : Variable Initialization
long long res = 0, n = nums.size();
Initializes `res` to store the result and `n` to store the size of the array.
3 : Outer Loop
for(int i = 0; i < n - 1; i++) {
Starts a loop that iterates over each element of the array, except the last one.
4 : Variable Initialization
int mini = nums[i], maxi = nums[i];
Sets the initial values of mini and maxi to the current element in the outer loop.
5 : Inner Loop
for(int j = i + 1; j < n; j++) {
Starts the inner loop that iterates over all elements after the current outer loop element.
6 : Condition for Mini Update
if(nums[j] < mini) mini = nums[j];
If the current element is smaller than mini, it updates mini.
7 : Condition for Maxi Update
if(nums[j] > maxi) maxi = nums[j];
If the current element is larger than maxi, it updates maxi.
8 : Result Calculation
res += maxi - mini;
Calculates the range (maxi - mini) for the current subarray and adds it to the result.
9 : Return Statement
return res;
Returns the accumulated result from the sum of ranges.
Best Case: O(n^2)
Average Case: O(n^2)
Worst Case: O(n^2)
Description: The worst case time complexity occurs when the array length is at its maximum.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is constant, as we are only storing a few variables for calculation.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus