Leetcode 2099: Find Subsequence of Length K With the Largest Sum
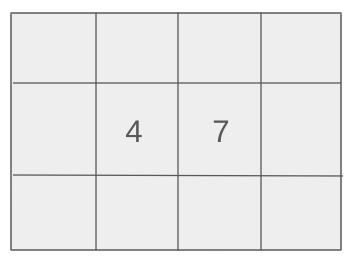
You are given an integer array ’nums’ and an integer ‘k’. You need to find a subsequence of ’nums’ of length ‘k’ such that the sum of its elements is maximized. A subsequence is a sequence that can be derived from another array by deleting some or no elements without changing the order of the remaining elements. Return any subsequence with the largest sum.
Problem
Approach
Steps
Complexity
Input: The input consists of the array 'nums' and an integer 'k' representing the desired subsequence length.
Example: nums = [4, 1, 3, 2], k = 2
Constraints:
• 1 <= nums.length <= 1000
• -10^5 <= nums[i] <= 10^5
• 1 <= k <= nums.length
Output: Return a subsequence of length 'k' with the largest sum.
Example: Output: [3, 4]
Constraints:
Goal: The goal is to find a subsequence of length 'k' that has the largest possible sum of elements.
Steps:
• Identify the 'k' largest elements in 'nums'.
• Keep their relative order from the original array.
• Return the subsequence formed by these elements.
Goal: The constraints ensure the solution handles up to 1000 elements efficiently and handles large or small integer values.
Steps:
• 1 <= nums.length <= 1000
• -10^5 <= nums[i] <= 10^5
• 1 <= k <= nums.length
Assumptions:
• The input list is not empty.
• The value of 'k' is valid (1 <= k <= nums.length).
• Input: Example 1: nums = [4, 1, 3, 2], k = 2
• Explanation: The subsequence of length 2 with the largest sum is [3, 4], as it has the sum of 7.
• Input: Example 2: nums = [2, -1, 5, 3], k = 2
• Explanation: The subsequence with the largest sum is [5, 3], as it gives the highest sum of 8.
Approach: The approach to solve the problem involves selecting the largest 'k' elements and maintaining their order in the original array.
Observations:
• We need to ensure that we select the largest elements while maintaining the order of the original array.
• A simple solution would involve sorting, but we need to track the indices of the selected elements to maintain their original order.
Steps:
• Sort the array to identify the largest 'k' elements.
• Track the indices of these elements in the original array.
• Construct the subsequence by selecting the elements in the order of their indices.
Empty Inputs:
• There are no edge cases for empty inputs as the problem guarantees that nums has at least 'k' elements.
Large Inputs:
• The algorithm should handle arrays of length up to 1000 efficiently.
Special Values:
• Handle cases where all elements are negative or where the largest values are repeated.
Constraints:
• The values of 'k' and the elements in 'nums' are guaranteed to be within the specified constraints.
vector<int> maxSubsequence(vector<int>& nums, int k) {
vector<int> v(nums.begin(), nums.end());
nth_element(v.begin(), v.begin() + k - 1, v.end(), greater<int>());
int cnt = count(v.begin(), v.begin() + k, v[k - 1]);
vector<int> res;
for(int i = 0; i < nums.size(); i++)
if((nums[i] > v[k - 1]) ||
(nums[i] == v[k - 1] && (cnt-- > 0)))
res.push_back(nums[i]);
return res;
}
1 : Function Definition
vector<int> maxSubsequence(vector<int>& nums, int k) {
This is the function signature for the maxSubsequence function, which takes an array 'nums' and an integer 'k' as parameters and returns a vector of integers.
2 : Initialization
vector<int> v(nums.begin(), nums.end());
Creates a copy of the 'nums' vector to allow manipulation without affecting the original array.
3 : Sorting
nth_element(v.begin(), v.begin() + k - 1, v.end(), greater<int>());
Rearranges the elements in 'v' such that the element at position 'k-1' is the largest element, ensuring the top k elements are correctly ordered.
4 : Counting
int cnt = count(v.begin(), v.begin() + k, v[k - 1]);
Counts how many times the k-th largest element appears in the first k elements of the vector 'v'. This count helps in handling ties during subsequence selection.
5 : Subsequence Construction
vector<int> res;
Initializes an empty vector 'res' to store the final subsequence.
6 : Iterating Over Elements
for(int i = 0; i < nums.size(); i++)
Starts a loop to iterate through all elements in the original 'nums' array to determine which elements belong in the subsequence.
7 : Condition Check 1
if((nums[i] > v[k - 1]) ||
Checks if the current element in 'nums' is greater than the k-th largest element from 'v', ensuring the largest elements are selected.
8 : Condition Check 2
(nums[i] == v[k - 1] && (cnt-- > 0)))
Handles cases where the current element equals the k-th largest element, ensuring that only the necessary number of such elements are selected.
9 : Subsequence Update
res.push_back(nums[i]);
Adds the current element to the subsequence if it meets the selection criteria.
10 : Return Statement
return res;
Returns the resulting subsequence as the output of the function.
Best Case: O(n log n)
Average Case: O(n log n)
Worst Case: O(n log n)
Description: The time complexity is dominated by the sorting step, which takes O(n log n).
Best Case: O(k)
Worst Case: O(n)
Description: The space complexity is O(n) due to storing the indices of the elements and the subsequence.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus