Leetcode 2073: Time Needed to Buy Tickets
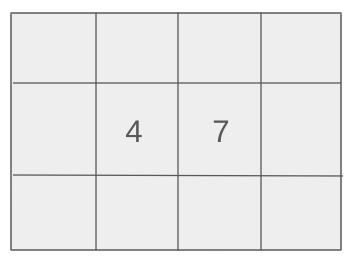
You are given a queue of
n
people, where each person wants to buy a specific number of tickets. The task is to determine the time taken for the person at index k
to finish buying all their tickets.Problem
Approach
Steps
Complexity
Input: You are given an array `tickets` of length `n` where each element represents the number of tickets the i-th person wants to buy, and an integer `k` representing the position of the person for whom we need to calculate the time.
Example: tickets = [3, 2, 5], k = 1
Constraints:
• 1 <= n <= 100
• 1 <= tickets[i] <= 100
• 0 <= k < n
Output: Return an integer representing the time taken for the person at position `k` to finish buying all their tickets.
Example: Output: 8
Constraints:
Goal: To calculate the total time it takes for the k-th person to finish buying their tickets based on the ticket queue process.
Steps:
• Start by simulating the process of people buying tickets.
• For each person, allow them to buy 1 ticket at a time and move to the back of the queue until they finish all their tickets.
• Keep track of the time it takes for the person at position k to complete the process.
Goal: The constraints specify the maximum values for the input sizes and the number of tickets each person wants.
Steps:
• n == tickets.length
• 1 <= n <= 100
• 1 <= tickets[i] <= 100
• 0 <= k < n
Assumptions:
• The people in the queue take 1 second to buy 1 ticket.
• Once a person finishes buying all their tickets, they leave the queue.
• Input: Example 1
• Explanation: In this example, the queue initially has the values [3, 2, 5], with the person at index 1 needing to buy tickets. After several rounds of buying tickets, the total time taken for the k-th person to finish is 8 seconds.
Approach: The problem is solved by simulating the process of people buying tickets. The k-th person’s time is tracked as the queue progresses.
Observations:
• We need to process each person in the queue until the person at index k has finished buying tickets.
• We can iterate over the queue repeatedly, subtracting 1 ticket from each person in each round, until the person at k finishes.
Steps:
• Initialize the time variable to 0.
• Loop through the queue, deducting tickets from each person until the person at index k completes their purchases.
• For each iteration, check if the person at index k is done, and return the time.
Empty Inputs:
• The queue must not be empty (n >= 1).
Large Inputs:
• For larger inputs, the algorithm should still work efficiently within the given constraints.
Special Values:
• When k is 0, the first person in the queue, they will finish earlier as they are at the front.
Constraints:
int timeRequiredToBuy(vector<int>& nums, int k) {
int res = 0, key = nums[k];
for(int i = 0; i < nums.size(); i++) {
res += min(key - (i > k), nums[i]);
}
return res;
}
1 : Function Declaration
int timeRequiredToBuy(vector<int>& nums, int k) {
This is the function signature for `timeRequiredToBuy`, which takes two inputs: `nums`, a vector representing the time required to buy each item, and `k`, the index of the item for which the time to purchase is calculated. It returns an integer representing the total time.
2 : Variable Declaration
int res = 0, key = nums[k];
This line declares two variables: `res` (the variable to accumulate the total time required) and `key` (the time required to buy the item at index `k`).
3 : Loop
for(int i = 0; i < nums.size(); i++) {
This loop iterates over the `nums` vector, processing each item to calculate the total time.
4 : Calculation
res += min(key - (i > k), nums[i]);
This line updates the total time `res`. It adds the smaller of two values: the time required for the item at index `i`, considering whether `i` is greater than `k`, and the actual time of the item.
5 : Return
return res;
This line returns the total accumulated time, which is the result of the calculation done in the loop.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The worst case occurs when each person buys at least 1 ticket, resulting in a time complexity of O(n).
Best Case: O(n)
Worst Case: O(n)
Description: We use O(n) space to store the tickets array and track the time.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|