Leetcode 2059: Minimum Operations to Convert Number
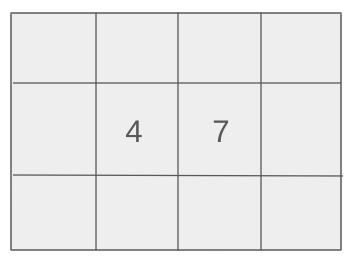
You are given a 0-indexed integer array nums, containing distinct numbers, an integer start, and an integer goal. There is an integer x, initially set to start, and you need to transform x into goal by repeatedly performing operations. You can use any number from the array nums in the following ways: x + nums[i], x - nums[i], or x ^ nums[i] (bitwise-XOR). You can perform each operation any number of times in any order. If the resulting number goes outside the range 0 <= x <= 1000, no further operations can be performed. The task is to determine the minimum number of operations required to convert x from start to goal, or return -1 if it is not possible.
Problem
Approach
Steps
Complexity
Input: You are provided with the following input parameters:
Example: Input: nums = [4, 5, 6], start = 3, goal = 9
Constraints:
• 1 <= nums.length <= 1000
• -10^9 <= nums[i], goal <= 10^9
• 0 <= start <= 1000
• start != goal
• All elements in nums are distinct.
Output: Return the minimum number of operations required to transform start to goal using the operations described, or -1 if it is not possible.
Example: Output: 2
Constraints:
• The result must be a non-negative integer or -1.
Goal: The goal is to determine the minimum number of operations to transform the number start into goal using the operations provided. The strategy involves exploring all possible transformations using a breadth-first search (BFS) approach.
Steps:
• 1. Initialize a queue and a set to track seen numbers.
• 2. Perform a breadth-first search, starting with the value 'start'.
• 3. For each number x, generate new numbers by performing the operations x + nums[i], x - nums[i], and x ^ nums[i], ensuring they are within the allowed range (0 <= x <= 1000).
• 4. Check if any of the new numbers equal goal, and return the current number of operations.
• 5. If no solution is found, return -1.
Goal: The algorithm should handle edge cases efficiently, especially those involving large inputs or impossible transformations.
Steps:
• Ensure the algorithm performs efficiently for up to 1000 elements in nums.
• Handle cases where it is impossible to transform start into goal.
Assumptions:
• The numbers in the nums array are distinct.
• Both start and goal are integers within the range [0, 1000].
• If no valid transformation sequence exists, return -1.
• Input: Input: nums = [2,4,12], start = 2, goal = 12
• Explanation: We can go from 2 → 14 → 12 with the following operations: 2 + 12 = 14, then 14 - 2 = 12. This requires 2 operations.
• Input: Input: nums = [3,5,7], start = 0, goal = -4
• Explanation: We can go from 0 → 3 → -4 with the following operations: 0 + 3 = 3, then 3 - 7 = -4. This requires 2 operations.
• Input: Input: nums = [2,8,16], start = 0, goal = 1
• Explanation: It is impossible to reach 1 starting from 0 with the given nums, so the output is -1.
Approach: The problem is best solved using a breadth-first search (BFS) strategy. BFS is ideal here as it explores all possible transformations level by level, ensuring the minimum number of operations is found.
Observations:
• We need to explore all possible transformations of x from start to goal.
• BFS will allow us to find the shortest path of transformations.
• Since BFS explores level by level, it ensures we find the minimum number of operations to reach the goal.
Steps:
• Step 1: Initialize a queue and a set to track visited numbers.
• Step 2: Start BFS from the 'start' value.
• Step 3: For each number in the queue, generate new numbers by performing the allowed operations (+, -, ^).
• Step 4: If a new number equals the goal, return the number of operations.
• Step 5: If no solution is found, return -1.
Empty Inputs:
• There will always be at least one number in nums, so no need to handle empty input.
Large Inputs:
• Handle cases where nums contains a large number of elements efficiently (up to 1000).
Special Values:
• Ensure the algorithm handles edge cases like unreachable goals or cases where transformations take the number out of the allowed range.
Constraints:
• The BFS should be optimized to avoid unnecessary computations and to handle the constraints efficiently.
int minimumOperations(vector<int>& nums, int start, int goal) {
queue<int> q;
set<int> seen;
q.push(start);
seen.insert(start);
int op = 0;
while(!q.empty()) {
int sz = q.size();
op++;
while(sz--) {
int x = q.front();
q.pop();
for(auto it: nums) {
if((x + it) == goal || (x - it) == goal || (x ^ it) == goal)
return op;
if(!seen.count(x + it) && x + it <= 1000 && x + it >= 0) {
seen.insert(x + it);
q.push(x + it);
}
if(!seen.count(x - it) && x - it <= 1000 && x - it >= 0) {
seen.insert(x - it);
q.push(x - it);
}
if(!seen.count(x ^ it) && (x ^ it) <= 1000 && (x ^ it) >= 0) {
seen.insert(x ^ it);
q.push(x ^ it);
}
}
}
}
return -1;
}
1 : Variable Initialization
int minimumOperations(vector<int>& nums, int start, int goal) {
Initialize the function 'minimumOperations' which takes in a vector 'nums' and two integers 'start' and 'goal' to calculate the minimum operations required.
2 : Queue Initialization
queue<int> q;
Initialize a queue 'q' for BFS traversal to explore the different possible values.
3 : Set Initialization
set<int> seen;
Create a set 'seen' to store the values that have already been processed, ensuring no value is revisited.
4 : Queue Operations
q.push(start);
Push the initial 'start' value into the queue to begin the BFS search.
5 : Set Operations
seen.insert(start);
Insert the 'start' value into the 'seen' set to mark it as processed.
6 : Variable Initialization
int op = 0;
Initialize a variable 'op' to track the number of operations (steps) taken to reach the goal.
7 : Looping
while(!q.empty()) {
Start a while loop that continues until all possible values are explored.
8 : Queue Size
int sz = q.size();
Store the current size of the queue, which represents the number of elements to be processed in this iteration.
9 : Counting
op++;
Increment the operation count as a new level of BFS is processed.
10 : Looping
while(sz--) {
Loop through each element at the current level in the BFS.
11 : Queue Operations
int x = q.front();
Get the front element of the queue to process.
12 : Queue Operations
q.pop();
Remove the front element from the queue after processing.
13 : Looping
for(auto it: nums) {
Loop through each element in the 'nums' vector to apply the operations.
14 : Conditional Logic
if((x + it) == goal || (x - it) == goal || (x ^ it) == goal)
Check if any operation (+, -, or ^) applied to the current value 'x' results in the 'goal'.
15 : Return Statement
return op;
If the 'goal' is reached, return the number of operations (steps) taken.
16 : Set Operations
if(!seen.count(x + it) && x + it <= 1000 && x + it >= 0) {
Check if the result of adding 'it' to 'x' has not been processed yet and falls within the valid range.
17 : Set Operations
seen.insert(x + it);
Insert the new value into the 'seen' set to mark it as processed.
18 : Queue Operations
q.push(x + it);
Push the new value into the queue for further processing.
19 : Set Operations
if(!seen.count(x - it) && x - it <= 1000 && x - it >= 0) {
Check if the result of subtracting 'it' from 'x' has not been processed yet and falls within the valid range.
20 : Set Operations
seen.insert(x - it);
Insert the new value into the 'seen' set to mark it as processed.
21 : Queue Operations
q.push(x - it);
Push the new value into the queue for further processing.
22 : Set Operations
if(!seen.count(x ^ it) && (x ^ it) <= 1000 && (x ^ it) >= 0) {
Check if the result of XOR'ing 'it' with 'x' has not been processed yet and falls within the valid range.
23 : Set Operations
seen.insert(x ^ it);
Insert the new XOR'd value into the 'seen' set.
24 : Queue Operations
q.push(x ^ it);
Push the new XOR'd value into the queue for further processing.
25 : Return Statement
return -1;
Return -1 if the goal cannot be reached with the available operations.
Best Case: O(1)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n) where n is the number of elements in nums, as we explore all possible transformations in BFS.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) due to the queue and the set used to track visited numbers.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus