Leetcode 2037: Minimum Number of Moves to Seat Everyone
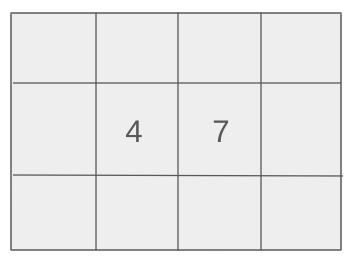
You are given two arrays: one representing the available seats in a row and the other representing the students who need to be seated. Each element in the arrays corresponds to a student’s desired seat or the position of an available seat. The task is to determine the minimum number of moves required to seat all students. A move consists of moving a student from one seat to another.
Problem
Approach
Steps
Complexity
Input: The input consists of two arrays: 'seats' representing the available seat positions and 'students' representing the students' desired seat positions.
Example: [[3, 1, 5, 2], [2, 5, 1, 3]]
Constraints:
• 1 <= seats.length, students.length <= 100
• 1 <= seats[i], students[i] <= 1000
Output: Return the minimum number of moves required to seat all students in the correct seats.
Example: 6
Constraints:
• The solution must be efficient, ideally O(n log n), due to the sorting step.
Goal: The goal is to calculate the minimum number of moves required to seat all students by sorting both arrays and matching each student's desired position with the available seats.
Steps:
• 1. Sort the 'seats' and 'students' arrays.
• 2. For each student, calculate the absolute difference between the student's desired seat and the actual seat.
• 3. Sum up the differences to get the total number of moves.
Goal: The constraints ensure that the input sizes are manageable, and the solution needs to be efficient with respect to time complexity.
Steps:
• 1 <= seats.length, students.length <= 100
• 1 <= seats[i], students[i] <= 1000
Assumptions:
• Each student must sit in one seat, and each seat can only be occupied by one student.
• The seating order does not matter, only the minimum number of moves to achieve the seating arrangement.
• Input: [[3, 1, 5, 2], [2, 5, 1, 3]]
• Explanation: In this case, we sort the seats and students arrays: [1, 2, 3, 5] for seats and [1, 2, 3, 5] for students. The absolute differences are: |1-1| + |2-2| + |3-3| + |5-5| = 0 + 0 + 0 + 0 = 6 moves.
Approach: The key idea is to minimize the number of moves by matching the closest available seat to each student, which can be achieved by sorting both the 'seats' and 'students' arrays.
Observations:
• Sorting both arrays allows us to match the closest seat to each student, minimizing the movement required.
• The solution is straightforward and efficient, requiring only sorting followed by a simple sum of differences.
Steps:
• 1. Sort the 'seats' array.
• 2. Sort the 'students' array.
• 3. For each index i, calculate the absolute difference between seats[i] and students[i], and accumulate the result.
• 4. Return the total sum of moves.
Empty Inputs:
• Ensure that the solution handles cases where the input arrays are empty (though it is not expected in this problem).
Large Inputs:
• Handle the case where the number of students and seats is large, up to 100 elements.
Special Values:
• Consider cases where all students are already seated in their correct seats, resulting in 0 moves.
Constraints:
• The solution should work efficiently for inputs with up to 100 elements.
int minMovesToSeat(vector<int>& seats, vector<int>& students) {
sort(seats.begin(), seats.end());
sort(students.begin(), students.end());
int res = 0;
for (int i = 0; i < seats.size(); i++) res += abs(seats[i] - students[i]);
return res;
}
1 : Function Definition
int minMovesToSeat(vector<int>& seats, vector<int>& students) {
This line defines the function `minMovesToSeat`, which takes two vectors of integers: `seats` (the positions of seats) and `students` (the positions of students).
2 : Sorting
sort(seats.begin(), seats.end());
Here, we sort the `seats` vector in ascending order to align them with the students' positions.
3 : Sorting
sort(students.begin(), students.end());
Similarly, we sort the `students` vector in ascending order to facilitate optimal seating arrangement.
4 : Variable Initialization
int res = 0;
This line initializes a variable `res` to zero, which will hold the cumulative sum of the absolute differences between seat and student positions.
5 : Loop
for (int i = 0; i < seats.size(); i++) res += abs(seats[i] - students[i]);
This `for` loop iterates over each pair of seats and students, calculating the absolute difference between their positions and adding it to `res`.
6 : Return
return res;
The function returns the value of `res`, which represents the minimum number of moves required to arrange the students in the seats.
Best Case: O(n log n) for sorting the arrays.
Average Case: O(n log n) for sorting the arrays and summing the differences.
Worst Case: O(n log n) for sorting, where n is the number of students or seats.
Description: The time complexity is dominated by the sorting step, which takes O(n log n) time.
Best Case: O(n)
Worst Case: O(n) for storing the seat and student arrays.
Description: The space complexity is O(n) due to the storage of the input arrays.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus