Leetcode 2033: Minimum Operations to Make a Uni-Value Grid
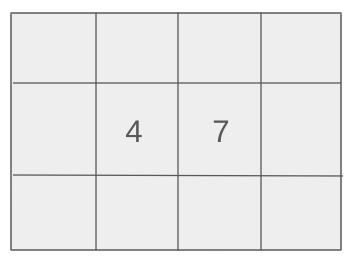
You are given a 2D grid of integers, and an integer
x
. In each operation, you can either add x
or subtract x
from any element in the grid. Your task is to transform the grid into a uni-value grid, where all elements are equal. Return the minimum number of operations needed to achieve this, or return -1 if it is not possible.Problem
Approach
Steps
Complexity
Input: The input consists of a 2D integer grid and an integer `x`.
Example: grid = [[2,4],[6,8]], x = 2
Constraints:
• 1 <= m, n <= 10^5
• 1 <= m * n <= 10^5
• 1 <= x, grid[i][j] <= 10^4
Output: The output is an integer representing the minimum number of operations needed to make the grid uni-value. If it is not possible, return -1.
Example: 4
Constraints:
• Return -1 if it's impossible to make all values equal.
Goal: Transform the grid to have all elements equal with the minimum number of operations.
Steps:
• 1. Check if all differences between grid elements and the first element are divisible by `x`. If not, return -1.
• 2. Sort the grid elements and find the median element.
• 3. Calculate the number of operations required to make all elements equal to the median element.
Goal: Ensure that the input grid has valid dimensions and values as per the given constraints.
Steps:
• 1 <= m, n <= 10^5
• 1 <= x, grid[i][j] <= 10^4
Assumptions:
• All values in the grid are integers.
• The grid can contain up to 100,000 elements.
• Input: grid = [[2,4],[6,8]], x = 2
• Explanation: We can make every element equal to 4 by performing the following operations:
- Add x to 2 once.
- Subtract x from 6 once.
- Subtract x from 8 twice.
Total operations = 4.
• Input: grid = [[1,5],[2,3]], x = 1
• Explanation: We can make all elements equal to 3 by performing the following operations:
- Add x to 1 twice.
- Subtract x from 5 twice.
- Subtract x from 2 once.
Total operations = 5.
• Input: grid = [[1,2],[3,4]], x = 2
• Explanation: It is impossible to make all elements equal because the differences between the elements are not divisible by 2. Therefore, return -1.
Approach: The problem can be solved by checking the divisibility of the differences between the elements and finding the median element in the grid.
Observations:
• We need to check if all differences between grid elements and the first element are divisible by `x`.
• Sorting the grid elements will allow us to easily find the median element.
• We can use the median element as the target value to minimize the number of operations needed.
Steps:
• 1. Traverse the grid and check if the difference between each element and the first element is divisible by `x`. If not, return -1.
• 2. Sort the elements of the grid.
• 3. Find the median element and calculate the number of operations needed to make all elements equal to the median.
Empty Inputs:
• If the grid is empty, return -1.
Large Inputs:
• For large grids, ensure efficient traversal and sorting steps.
Special Values:
• Handle grids where all elements are already equal (0 operations needed).
Constraints:
• Ensure all grid values are within the specified range.
int minOperations(vector<vector<int>>& grid, int x) {
int m = grid.size(), n = grid[0].size();
int num = grid[0][0];
vector<int> arr;
for(int i = 0; i < m; i++)
for(int j = 0; j < n; j++) {
arr.push_back(grid[i][j]);
if(abs(num - grid[i][j]) % x != 0) return -1;
}
sort(arr.begin(), arr.end());
int pos = arr.size() / 2;
int res = 0;
for(int i = 0; i < arr.size(); i++)
res += (abs(arr[pos] - arr[i]) / x);
return res;
}
1 : Function Definition
int minOperations(vector<vector<int>>& grid, int x) {
This defines the 'minOperations' function, which calculates the minimum operations needed to make all elements in the grid divisible by 'x'.
2 : Grid Size Calculation
int m = grid.size(), n = grid[0].size();
This line determines the number of rows 'm' and columns 'n' in the 2D grid.
3 : Initial Number Assignment
int num = grid[0][0];
Here, the first element of the grid is assigned to the variable 'num', which is used to check divisibility conditions for all elements.
4 : Array Initialization
vector<int> arr;
An empty vector 'arr' is created to store all the elements of the grid for further processing.
5 : Grid Traversal
for(int i = 0; i < m; i++)
This loop iterates through all the rows of the grid.
6 : Nested Loop Start
for(int j = 0; j < n; j++) {
This nested loop iterates through all the columns of the grid.
7 : Array Population
arr.push_back(grid[i][j]);
Each element of the grid is pushed into the 'arr' vector.
8 : Divisibility Check
if(abs(num - grid[i][j]) % x != 0) return -1;
This checks if the absolute difference between 'num' and the current grid element is divisible by 'x'. If not, the function returns -1, indicating that the transformation is impossible.
9 : Array Sorting
sort(arr.begin(), arr.end());
The vector 'arr' is sorted in ascending order to prepare for the median calculation.
10 : Median Calculation
int pos = arr.size() / 2;
The position of the median element is calculated by dividing the size of the array by 2.
11 : Result Initialization
int res = 0;
The variable 'res' is initialized to store the total number of operations.
12 : Operation Calculation Loop
for(int i = 0; i < arr.size(); i++)
This loop iterates over all the elements of the sorted array to compute the number of operations required for each element.
13 : Operation Calculation
res += (abs(arr[pos] - arr[i]) / x);
For each element, the absolute difference between the element and the median is calculated and divided by 'x' to get the number of operations. This value is added to 'res'.
14 : Return Result
return res;
The total number of operations 'res' is returned.
Best Case: O(m * n * log(m * n))
Average Case: O(m * n * log(m * n))
Worst Case: O(m * n * log(m * n))
Description: The time complexity is dominated by the sorting step which takes O(m * n * log(m * n)) for a grid of size m x n.
Best Case: O(m * n)
Worst Case: O(m * n)
Description: The space complexity is O(m * n) due to storing the grid elements in an array.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus