Leetcode 2011: Final Value of Variable After Performing Operations
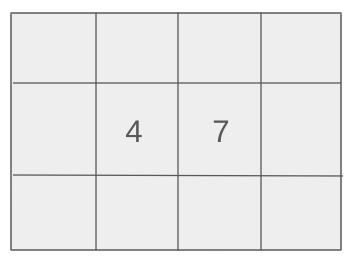
In a simplified programming language, there are only four operations: incrementing or decrementing a variable X by 1. You are given a list of operations that can either increment or decrement the value of X. Your task is to determine the final value of X after applying all the operations.
Problem
Approach
Steps
Complexity
Input: You are given an array of strings, where each string represents an operation on the variable X. The operations can be any of the following: '++X', 'X++', '--X', or 'X--'. Initially, X is set to 0.
Example: operations = ["X++", "--X", "++X"]
Constraints:
• 1 <= operations.length <= 100
• operations[i] will be either '++X', 'X++', '--X', or 'X--'.
Output: Return the final value of X after performing all operations.
Example: Output: 1
Constraints:
• The output will be an integer.
Goal: The goal is to calculate the final value of X after applying all the operations sequentially.
Steps:
• Start with X initialized to 0.
• Iterate through each operation in the list.
• For each operation, update the value of X accordingly: increment if the operation is '++X' or 'X++', decrement if the operation is '--X' or 'X--'.
• Return the final value of X.
Goal: The operations array will contain at least one operation, and no more than 100 operations.
Steps:
• 1 <= operations.length <= 100
• operations[i] will be either '++X', 'X++', '--X', or 'X--'.
Assumptions:
• The initial value of X is 0.
• The operations are applied in the order they appear in the list.
• Input: Example 1: Input: operations = ["X++", "--X", "++X"]
• Explanation: The operations are applied as follows: X = 0 initially. 'X++' increments X to 1, '--X' decrements X to 0, '++X' increments X to 1. The final value of X is 1.
• Input: Example 2: Input: operations = ["++X", "++X", "X++"]
• Explanation: The operations are applied as follows: X = 0 initially. '++X' increments X to 1, '++X' increments X to 2, 'X++' increments X to 3. The final value of X is 3.
• Input: Example 3: Input: operations = ["X++", "--X", "X--"]
• Explanation: The operations are applied as follows: X = 0 initially. 'X++' increments X to 1, '--X' decrements X to 0, 'X--' decrements X to -1. The final value of X is -1.
Approach: We can simply iterate over the operations list, update the value of X based on each operation, and return the final value.
Observations:
• The operations only involve simple increments and decrements of X.
• The operations are applied in sequence, and each operation affects the value of X directly.
• Since the operations are straightforward and independent, we can solve this problem using a simple loop to apply each operation sequentially.
Steps:
• Initialize a variable X with a value of 0.
• Loop through each operation in the operations array.
• Update the value of X based on the operation (increment or decrement).
• Return the final value of X after all operations are applied.
Empty Inputs:
• The input array will never be empty, as per the constraints.
Large Inputs:
• The solution should handle the maximum input size of 100 operations efficiently.
Special Values:
• The initial value of X is always 0.
Constraints:
• Ensure that the operations are valid as per the given constraints.
int finalValueAfterOperations(vector<string>& operations)
{
int X = 0;
for(int i=0;i<operations.size();i++)
{
if(operations[i]=="X++")
{
X++;
}
else if(operations[i] == "++X")
{
++X;
}
else if(operations[i] == "X--")
{
X--;
}
else if(operations[i] == "--X")
{
--X;
}
}
return X;
}
1 : Function Definition
int finalValueAfterOperations(vector<string>& operations)
Defines the function `finalValueAfterOperations` that takes a vector of strings representing operations and returns the final value of `X`.
2 : Variable Initialization
int X = 0;
Initializes the variable `X` to 0, which will hold the result of the operations.
3 : Loop Initialization
for(int i=0;i<operations.size();i++)
Starts a for loop to iterate over each operation in the `operations` vector.
4 : Conditional Check
if(operations[i]=="X++")
Checks if the current operation is `X++`, which increments `X`.
5 : Increment Operation
X++;
Increments the value of `X` by 1.
6 : Conditional Check
else if(operations[i] == "++X")
Checks if the current operation is `++X`, which also increments `X`.
7 : Increment Operation
++X;
Increments `X` by 1 before returning the value.
8 : Conditional Check
else if(operations[i] == "X--")
Checks if the current operation is `X--`, which decrements `X`.
9 : Decrement Operation
X--;
Decrements `X` by 1.
10 : Conditional Check
else if(operations[i] == "--X")
Checks if the current operation is `--X`, which also decrements `X`.
11 : Decrement Operation
--X;
Decrements `X` by 1 before returning the value.
12 : Return Statement
return X;
Returns the final value of `X` after all operations have been performed.
Best Case: O(n), where n is the number of operations.
Average Case: O(n), where n is the number of operations.
Worst Case: O(n), where n is the number of operations.
Description: The time complexity is linear because we need to process each operation once.
Best Case: O(1), as the space requirement does not depend on the input size.
Worst Case: O(1), as we only need a constant amount of space to store the value of X.
Description: The space complexity is constant because we only store the value of X.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus