Leetcode 2006: Count Number of Pairs With Absolute Difference K
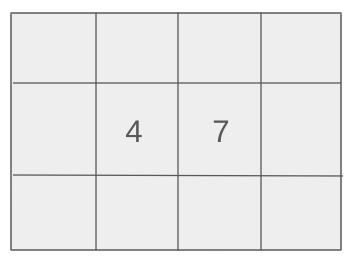
Given an integer array nums and an integer k, return the number of pairs (i, j) where i < j such that |nums[i] - nums[j]| == k.
Problem
Approach
Steps
Complexity
Input: The input consists of an integer array nums and an integer k.
Example: nums = [3, 5, 2, 6, 7], k = 3
Constraints:
• 1 <= nums.length <= 200
• 1 <= nums[i] <= 100
• 1 <= k <= 99
Output: Return the number of pairs (i, j) where i < j such that |nums[i] - nums[j]| == k.
Example: 2
Constraints:
Goal: The goal is to count the number of pairs with an absolute difference of k.
Steps:
• 1. Create a frequency count of the elements in the array.
• 2. For each element x, check if x + k or x - k exists in the array.
• 3. Add the count of valid pairs for each element.
• 4. Return the total count of valid pairs.
Goal: The input array is between 1 and 200 elements long, with values ranging from 1 to 100 for each element.
Steps:
• 1 <= nums.length <= 200
• 1 <= nums[i] <= 100
• 1 <= k <= 99
Assumptions:
• The solution should be efficient given the constraints.
• Handling of duplicate elements is necessary to avoid over-counting pairs.
• Input: nums = [3, 5, 2, 6, 7], k = 3
• Explanation: The valid pairs with an absolute difference of 3 are (3, 6) and (5, 2). Thus, the output is 2.
• Input: nums = [1, 1, 3, 5], k = 2
• Explanation: The valid pair with an absolute difference of 2 is (3, 5). Hence, the output is 1.
• Input: nums = [8, 2, 5, 6, 9], k = 4
• Explanation: The valid pairs with an absolute difference of 4 are (8, 4) and (5, 9). The output is 2.
Approach: To solve this problem efficiently, we can use a hash map to count the occurrences of each number in the array and use it to check if the corresponding pairs exist that satisfy the condition.
Observations:
• We need to check each element's potential to form a valid pair with another element based on the given difference k.
• Handling multiple occurrences of the same number requires careful counting.
• A hash map will help in efficiently checking if a number exists that satisfies the absolute difference condition.
Steps:
• 1. Create an array or hash map to store the count of each element in the array.
• 2. For each number, check if the number + k or number - k exists in the array and count the pairs accordingly.
• 3. Sum the pairs and return the result.
Empty Inputs:
• If the array is empty, the result should be 0 since there are no elements to form pairs.
Large Inputs:
• If the array has a large number of elements, ensure the solution handles them efficiently with linear or near-linear time complexity.
Special Values:
• If the array contains duplicate elements, ensure not to double-count pairs that are the same but occur at different indices.
Constraints:
• Make sure the solution handles arrays of size up to 200 elements efficiently.
int countKDifference(vector<int>& nums, int k) {
int cnt[101] = {}, res = 0;
for (auto n : nums)
++cnt[n];
for (int i = k + 1; i < 101; ++i)
res += cnt[i] * cnt[i - k];
return res;
}
1 : Function Definition
int countKDifference(vector<int>& nums, int k) {
Defines the function `countKDifference` which takes a vector `nums` and an integer `k` as inputs, and returns the number of pairs with the absolute difference of `k`.
2 : Variable Declaration
int cnt[101] = {}, res = 0;
Declares an array `cnt` of size 101 to store the frequency count of each element in the range [0, 100] and an integer `res` to store the final result.
3 : Loop Iteration
for (auto n : nums)
Starts a loop to iterate through each element `n` in the input vector `nums`.
4 : Frequency Counting
++cnt[n];
Increments the count of the number `n` in the `cnt` array, effectively counting the frequency of each number in `nums`.
5 : Loop Iteration
for (int i = k + 1; i < 101; ++i)
Starts another loop that iterates through numbers starting from `k+1` up to 100 to check if there exists a corresponding pair with the difference of `k`.
6 : Result Calculation
res += cnt[i] * cnt[i - k];
For each `i`, it calculates the number of valid pairs by multiplying the count of `i` and the count of `i-k`, adding this to the result `res`.
7 : Return Statement
return res;
Returns the final result, which is the number of valid pairs with the absolute difference equal to `k`.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n) because we loop over the array once to count elements and then check for pairs in constant time using a frequency map.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) because we use a frequency map to store counts of each element in the array.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus