Leetcode 1995: Count Special Quadruplets
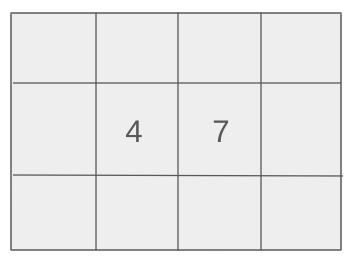
Given a 0-indexed integer array ’nums’, return the number of distinct quadruplets (a, b, c, d) such that:
- nums[a] + nums[b] + nums[c] == nums[d], and
- a < b < c < d.
Problem
Approach
Steps
Complexity
Input: The input consists of a single integer array nums of length n (4 <= n <= 50) where each element nums[i] satisfies 1 <= nums[i] <= 100.
Example: nums = [1, 2, 3, 6]
Constraints:
• 4 <= nums.length <= 50
• 1 <= nums[i] <= 100
Output: Return the number of distinct quadruplets (a, b, c, d) such that nums[a] + nums[b] + nums[c] == nums[d], with a < b < c < d.
Example: 1
Constraints:
Goal: We need to find quadruplets where the sum of three elements equals the fourth element, and the indices are in increasing order.
Steps:
• 1. Iterate through the array starting from the end.
• 2. Use a hashmap to track previous sums and their frequencies.
• 3. For each new element, check if the sum of two previous elements matches any previously tracked sum.
• 4. Update the result accordingly.
Goal: The constraints ensure the input size is manageable and the problem is solvable within time limits.
Steps:
• The input array length is between 4 and 50.
• Each element in the array is between 1 and 100.
Assumptions:
• All quadruplets should have distinct indices.
• The array is non-empty and follows the given constraints.
• Input: nums = [1, 1, 1, 3, 5]
• Explanation: Here, there are 4 valid quadruplets: (0, 1, 2, 3), (0, 1, 3, 4), (0, 2, 3, 4), and (1, 2, 3, 4).
Approach: We can solve this problem efficiently using a hashmap to track sums of pairs and their frequencies as we iterate through the array.
Observations:
• The problem requires us to check sums of three elements against another element.
• A brute-force approach would involve checking all possible quadruplets, but this would be inefficient for larger arrays. A hashmap can help reduce the complexity.
Steps:
• 1. Initialize a hashmap to store the frequency of sums.
• 2. Traverse the array from right to left, updating the hashmap with the current number.
• 3. For each element, check if there exists a sum in the hashmap that matches the current number.
• 4. Accumulate the count of valid quadruplets.
Empty Inputs:
• There are no edge cases involving empty inputs since the array length is guaranteed to be at least 4.
Large Inputs:
• For larger inputs, the algorithm should perform efficiently due to the hashmap optimization.
Special Values:
• The input values can be the same, so we need to handle duplicates.
Constraints:
• The constraints are manageable for the given approach.
int countQuadruplets(vector<int>& nums) {
const auto n = nums.size();
unordered_map<int, int> freq;
freq[nums[n - 1]] = 1;
size_t answ = 0;
for (int i = n - 2; i > 1; --i)
{
for (int j = i - 1; j > 0; --j)
{
for (int k = j - 1; k >= 0; --k)
{
if (freq.count(nums[i] + nums[j] + nums[k]))
{
answ += freq[nums[i] + nums[j] + nums[k]];
}
}
}
freq[nums[i]] += 1;
}
return answ;
}
1 : Function Definition
int countQuadruplets(vector<int>& nums) {
The function definition for `countQuadruplets` that takes in a vector of integers, `nums`, and returns the count of quadruplets.
2 : Variable Initialization
const auto n = nums.size();
This initializes the variable `n` to hold the size of the `nums` vector, which is used to control the iteration limits.
3 : Variable Initialization
unordered_map<int, int> freq;
An unordered map `freq` is initialized to store the frequency of sum values encountered during iteration.
4 : Map Update
freq[nums[n - 1]] = 1;
The last element of `nums` is added to the `freq` map with an initial count of 1. This prepares the map to store the frequencies of potential sums.
5 : Variable Initialization
size_t answ = 0;
A variable `answ` is initialized to 0 to keep track of the count of quadruplets that satisfy the required sum condition.
6 : Outer Loop
for (int i = n - 2; i > 1; --i)
The outer loop starts from the second last element of the array `nums` and iterates backwards.
7 : Middle Loop
for (int j = i - 1; j > 0; --j)
The middle loop iterates backwards from one element before the current element in the outer loop.
8 : Inner Loop
for (int k = j - 1; k >= 0; --k)
The inner loop iterates backwards from one element before the current element in the middle loop.
9 : Condition Check
if (freq.count(nums[i] + nums[j] + nums[k]))
Checks if the sum of `nums[i] + nums[j] + nums[k]` is present in the `freq` map.
10 : Block Start
{
Marks the start of the block for the condition where a sum is found in the `freq` map.
11 : Count Update
answ += freq[nums[i] + nums[j] + nums[k]];
If the sum exists in `freq`, the corresponding frequency is added to the `answ` variable, incrementing the count of valid quadruplets.
12 : Map Update
freq[nums[i]] += 1;
Updates the frequency of the current element `nums[i]` in the `freq` map.
13 : Return Statement
return answ;
Returns the total count of quadruplets that satisfy the given condition.
Best Case: O(n^3)
Average Case: O(n^3)
Worst Case: O(n^3)
Description: In the worst case, we check all pairs of elements in the array, leading to a time complexity of O(n^3).
Best Case: O(n^2)
Worst Case: O(n^2)
Description: The space complexity is O(n^2) due to the hashmap used for storing sums.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus