Leetcode 1980: Find Unique Binary String
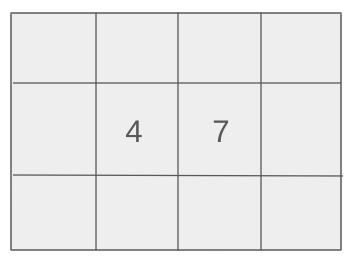
You are given an array of unique binary strings, each of length n. Your task is to find and return a binary string of length n that is not present in the given array. There can be multiple valid solutions.
Problem
Approach
Steps
Complexity
Input: You are given an integer n representing the number of binary strings in the array nums, and a list of strings nums where each string is a binary number of length n.
Example: nums = ['00', '11']
Constraints:
• 1 <= n <= 16
• nums.length == n
• nums[i].length == n
• All strings in nums are unique binary strings.
Output: Return a binary string of length n that is not present in the array nums. If there are multiple valid answers, any valid one can be returned.
Example: Output: '10'
Constraints:
• The returned string must not be in the input array.
Goal: The goal is to identify a binary string that does not match any of the given strings in nums. The approach relies on constructing a string that is guaranteed to differ from each input string at least in one position.
Steps:
• Create an empty result string.
• Iterate through the array nums and for each index i, pick the opposite of the character at index i of the i-th string in nums.
• Return the constructed binary string as the result.
Goal: Ensure the solution is efficient even for the largest inputs.
Steps:
• The maximum number of binary strings is 16, and the maximum length of each string is also 16.
Assumptions:
• The binary strings in nums are unique and of the same length.
• Input: Input: nums = ['01', '10']
• Explanation: In this case, the binary strings in nums are '01' and '10'. By flipping each corresponding bit, we can create '11', which does not appear in the input array.
• Input: Input: nums = ['11', '00']
• Explanation: The binary strings in nums are '11' and '00'. By flipping each bit in the corresponding positions, we get '00', which is already in the list. Hence, '10' or '01' can be a valid output.
Approach: The solution uses a simple technique of generating a binary string that differs from each input string at least in one position by flipping corresponding bits.
Observations:
• This is a problem of generating a unique binary string not present in the input.
• By flipping the bits at each index, we can guarantee a string that does not appear in the input array.
Steps:
• Iterate over the array nums.
• For each binary string in nums, flip the bit at the same index in the result string.
• Return the result string after completing the loop.
Empty Inputs:
• If the array nums is empty, return a string with all bits set to '1'.
Large Inputs:
• For large arrays with n = 16, the solution should handle these inputs efficiently.
Special Values:
• If nums contains a single binary string, then any binary string differing from it will work.
Constraints:
• The maximum length of the binary string and the array size should be handled within the time complexity.
string findDifferentBinaryString(vector<string>& nums) {
string ans = "";
int n = nums.size();
for(int i = 0; i < n; i++)
ans += nums[i][i] == '0'? '1':'0';
return ans;
}
1 : Function Definition
string findDifferentBinaryString(vector<string>& nums) {
Define the function `findDifferentBinaryString` that takes a vector of binary strings and returns a new binary string.
2 : Initialize Answer String
string ans = "";
Initialize an empty string `ans` which will hold the resulting binary string.
3 : Get Size of Input
int n = nums.size();
Get the size of the input vector `nums` and store it in `n`.
4 : Loop Through Strings
for(int i = 0; i < n; i++)
Loop through each binary string in the input vector `nums`.
5 : Build the Answer String
ans += nums[i][i] == '0'? '1':'0';
For each string in the input vector, if the character at index `i` is '0', append '1' to `ans`; otherwise, append '0'.
6 : Return Result
return ans;
Return the constructed binary string `ans` which is different from each of the input binary strings at the corresponding positions.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n), where n is the length of the binary strings in nums.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) due to the storage of the result string.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus