Leetcode 1974: Minimum Time to Type Word Using Special Typewriter
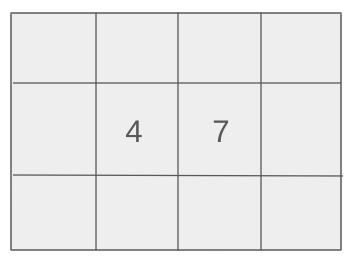
You are given a special typewriter with lowercase English letters (‘a’ to ‘z’) arranged in a circle. A pointer initially starts at the character ‘a’. Each second, you may perform one of two operations: move the pointer one character clockwise or counterclockwise, or type the character the pointer is currently on. Your task is to determine the minimum number of seconds needed to type the given string
word
.Problem
Approach
Steps
Complexity
Input: The input consists of a string `word` of length `n`, where 1 <= n <= 100, consisting of lowercase English letters.
Example: word = 'xyz'
Constraints:
• 1 <= word.length <= 100
• word consists of lowercase English letters.
Output: The output is a single integer, which represents the minimum number of seconds required to type the entire word.
Example: Output: 12
Constraints:
• The result should be an integer.
Goal: To compute the minimum number of seconds to type out the characters in the word.
Steps:
• Step 1: Initialize a variable to keep track of the current pointer position, starting at 'a'.
• Step 2: For each character in the word, calculate the time required to move the pointer to the target character (either clockwise or counterclockwise).
• Step 3: Accumulate the time spent on each move and typing action to get the total time.
• Step 4: Return the total time as the result.
Goal: Ensure the solution handles up to 100 characters efficiently.
Steps:
• The word will not be empty and will only consist of lowercase English letters.
• The maximum length of the word is 100 characters.
Assumptions:
• The word consists only of lowercase letters from 'a' to 'z'.
• There are no special characters or spaces in the input word.
• Input: Input: word = 'abc'
• Explanation: Starting with the pointer at 'a', the steps would be: Type 'a' (1 second), move to 'b' (1 second), type 'b' (1 second), move to 'c' (1 second), and type 'c' (1 second). The total time is 5 seconds.
• Input: Input: word = 'bza'
• Explanation: Starting with the pointer at 'a', the steps would be: Move to 'b' (1 second), type 'b' (1 second), move counterclockwise to 'z' (2 seconds), type 'z' (1 second), move clockwise to 'a' (1 second), and type 'a' (1 second). The total time is 7 seconds.
• Input: Input: word = 'zjpc'
• Explanation: Starting with the pointer at 'a', the steps would be: Move counterclockwise to 'z' (1 second), type 'z' (1 second), move clockwise to 'j' (10 seconds), type 'j' (1 second), move to 'p' (6 seconds), type 'p' (1 second), move counterclockwise to 'c' (13 seconds), and type 'c' (1 second). The total time is 34 seconds.
Approach: To solve this problem, we calculate the minimal time required to move the pointer to each character in the word. The key observation is to find the shortest distance between the current pointer position and the target character, either clockwise or counterclockwise, and add the time to type that character.
Observations:
• The problem can be approached by calculating the minimal distance between two characters, considering the circular nature of the alphabet.
• A direct approach involves iterating through the string and updating the pointer position as we compute the minimum time to reach each successive character.
Steps:
• Step 1: Initialize the total time to be the length of the word, since each character requires 1 second to type.
• Step 2: Track the current pointer position, starting at 'a'.
• Step 3: For each character in the word, calculate the minimum number of seconds to move the pointer to the target character, either clockwise or counterclockwise.
• Step 4: Add the time taken to move the pointer and type the character to the total time.
• Step 5: Return the total time after processing all characters in the word.
Empty Inputs:
• The word will always have at least one character, as per the constraints.
Large Inputs:
• The solution must efficiently handle strings of up to 100 characters.
Special Values:
• If the word consists of the same repeated character (e.g., 'aaaa'), the time will be minimized as no movement is required after the first character.
Constraints:
• The alphabet is circular, so ensure that the minimal distance between characters is always correctly calculated.
int minTimeToType(string word) {
int res = word.size(), point = 'a';
for (auto ch : word) {
res += min(abs(ch - point), 26 - abs(point - ch));
point = ch;
}
return res;
}
1 : Function Definition
int minTimeToType(string word) {
This function calculates the minimum time to type a given word on a circular keyboard. It takes the word as input and returns the total time required.
2 : Variable Initialization
int res = word.size(), point = 'a';
The variable 'res' is initialized to the length of the word, representing the base time to type the word. The variable 'point' keeps track of the current position on the keyboard (starting from 'a').
3 : Loop
for (auto ch : word) {
This loop iterates over each character in the word to calculate the time required to move from the current character to the next.
4 : Min Time Calculation
res += min(abs(ch - point), 26 - abs(point - ch));
This line computes the minimum time to move from the current 'point' to the character 'ch'. It calculates both the direct and circular distances between the characters and adds the minimum of these two to 'res'.
5 : Update Point
point = ch;
After typing the current character, 'point' is updated to the current character 'ch' as the new starting point for the next character.
6 : Return Result
return res;
The function returns the total time accumulated in 'res', which represents the minimum time required to type the word.
Best Case: O(n), where `n` is the length of the word.
Average Case: O(n)
Worst Case: O(n)
Description: For each character in the word, we calculate the minimal movement to the target character, making the time complexity O(n), where `n` is the length of the word.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is O(1), as we only use a few variables to track the current pointer and total time.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus