Leetcode 1959: Minimum Total Space Wasted With K Resizing Operations
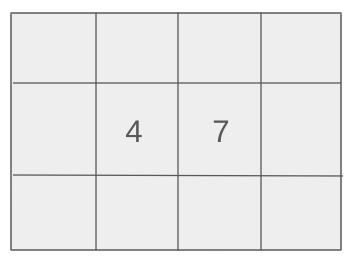
You are tasked with designing a dynamic array where the number of elements that should be in the array at each time is provided in an integer array
nums
. Additionally, you are given an integer k
, representing the maximum number of times you can resize the array. At each time step, the array’s size must be large enough to hold the elements in nums[i]
and any extra space will be wasted. Your goal is to minimize the total wasted space by resizing the array at most k
times.Problem
Approach
Steps
Complexity
Input: The input consists of an array `nums` where each `nums[i]` represents the number of elements that need to be in the array at time `i`. You are also given an integer `k` which specifies the maximum number of resizes allowed.
Example: nums = [15, 25, 10], k = 1
Constraints:
• 1 <= nums.length <= 200
• 1 <= nums[i] <= 10^6
• 0 <= k <= nums.length - 1
Output: The output should be the minimum total space wasted if you can resize the array at most `k` times.
Example: Output: 5
Constraints:
• The array can be resized at most `k` times.
Goal: The objective is to minimize the total wasted space by determining when and how to resize the array optimally.
Steps:
• Step 1: Start by setting an initial size for the array.
• Step 2: Check the amount of space wasted at each time step based on the current size and the number of elements required.
• Step 3: Resize the array up to `k` times if it reduces the total wasted space.
Goal: Ensure that the array is resized no more than `k` times and that the array size can hold all the required elements at each time step.
Steps:
• The size of the array at each time `t` must be at least `nums[t]`.
• Resizing the array can be done at most `k` times.
Assumptions:
• The input array will always be valid with non-negative values.
• The number of resizes will not exceed the length of the array minus one.
• Input: Input: nums = [15, 25, 10], k = 1
• Explanation: In this case, the initial array size could be set to 25 to accommodate the first two values, and a resize can be made at time 3 to accommodate the value 10. The total wasted space is 5.
Approach: The approach is to start by choosing an initial size for the array and then check the space wasted at each step. The key to minimizing wasted space is to resize the array optimally and avoid unnecessary resizes.
Observations:
• Resizing too frequently could increase wasted space, but resizing too infrequently may result in larger wasted space.
• The problem is about finding a balance between resizing the array and minimizing wasted space at each step.
Steps:
• Step 1: For each potential resizing scenario, calculate the total space wasted if the array is resized.
• Step 2: Use dynamic programming to check different ways of resizing the array to minimize space waste.
• Step 3: Keep track of the best solution and return the minimum wasted space.
Empty Inputs:
• There will always be at least one element in the `nums` array.
Large Inputs:
• Ensure that the solution works efficiently for the maximum input size.
Special Values:
• Consider scenarios where no resizes are needed, i.e., `k = 0`.
Constraints:
• The number of resizes will be constrained to `k` times.
int n;
vector<int> nums;
int memo[201][200];
int dp(int k, int idx) {
if(idx == n) return 0;
if(k == 0) return INT_MAX;
if(memo[k][idx] != -1) return memo[k][idx];
int ans = INT_MAX, sum = 0, mx = nums[idx];
for(int j = idx; j < n; j++) {
mx = max(mx, nums[j]);
sum += nums[j];
int wasted = mx * (j - idx + 1) - sum;
int res = dp(k - 1, j + 1);
ans = min(ans, wasted + (res == INT_MAX? INT_MAX - wasted: res));
}
return memo[k][idx] = ans;
}
int minSpaceWastedKResizing(vector<int>& nums, int k) {
n = nums.size();
this->nums = nums;
memset(memo, -1, sizeof(memo));
return dp(k + 1, 0);
}
1 : Initialization
Initializing variables for the dynamic programming function.
2 : Variable Declaration
int n;
Declare variable n to store the size of the input vector nums.
3 : Vector Declaration
vector<int> nums;
Declare a vector nums to store the input integers.
4 : Array Declaration
int memo[201][200];
Declare a 2D array memo for memoization, to store the results of subproblems.
5 : Function Definition
int dp(int k, int idx) {
Define the recursive function dp, which calculates the minimum space wasted given k partitions and the current index.
6 : Base Case
if(idx == n) return 0;
Base case: if the index has reached the end of the array, return 0 since there is no remaining space.
7 : Base Case
if(k == 0) return INT_MAX;
Base case: if no partitions are allowed, return a large number to signify an invalid case.
8 : Memoization Check
Check if the result for the current state (k, idx) has already been computed.
9 : Memoization Check
if(memo[k][idx] != -1) return memo[k][idx];
Return the precomputed result from the memoization array if it exists.
10 : Variable Initialization
int ans = INT_MAX, sum = 0, mx = nums[idx];
Initialize variables: ans stores the minimum space wasted, sum tracks the current sum, and mx stores the maximum value in the current partition.
11 : Loop
for(int j = idx; j < n; j++) {
Iterate over all possible ending indices for the current partition.
12 : Max Calculation
mx = max(mx, nums[j]);
Update the maximum value in the current partition.
13 : Sum Calculation
sum += nums[j];
Add the current element to the sum for the current partition.
14 : Wasted Space Calculation
int wasted = mx * (j - idx + 1) - sum;
Calculate the wasted space for the current partition by comparing the area that would be taken by the max value with the actual sum.
15 : Recursive Call
int res = dp(k - 1, j + 1);
Recursively call dp to calculate the result for the remaining partitions after the current partition.
16 : Result Calculation
ans = min(ans, wasted + (res == INT_MAX? INT_MAX - wasted: res));
Update the minimum answer by considering the wasted space for the current partition and the result from the recursive call.
17 : Return Statement
return memo[k][idx] = ans;
Store and return the result for the current state (k, idx) to avoid redundant calculations.
18 : Function Definition
int minSpaceWastedKResizing(vector<int>& nums, int k) {
Define the function to find the minimum space wasted after resizing the vector with k partitions.
19 : Vector Initialization
n = nums.size();
Initialize n with the size of the input vector.
20 : Vector Assignment
this->nums = nums;
Assign the input vector nums to the member variable nums.
21 : Memoization Setup
memset(memo, -1, sizeof(memo));
Set all entries in the memo array to -1 to signify that no subproblems have been solved yet.
22 : Return Result
return dp(k + 1, 0);
Call the dp function with k + 1 partitions and starting from index 0, and return the result.
Best Case: O(n)
Average Case: O(n^2)
Worst Case: O(n^2)
Description: The worst case involves checking every possible combination of resizes, which leads to a time complexity of O(n^2).
Best Case: O(n)
Worst Case: O(n*k)
Description: The space complexity is proportional to the number of resizes and the length of the array.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus