Leetcode 1947: Maximum Compatibility Score Sum
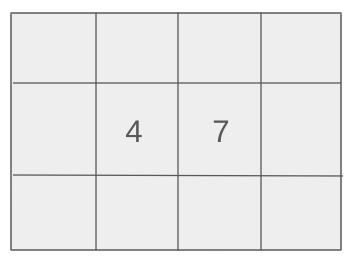
You are given a survey with n questions where each question’s answer is either 0 (no) or 1 (yes). The survey is completed by m students and m mentors. Each student’s answers are represented by a 2D array of size m x n, and similarly, each mentor’s answers are represented by another 2D array. The compatibility score of a student-mentor pair is calculated as the number of answers that are the same for both the student and the mentor. Your task is to find the optimal pairing of students to mentors that maximizes the sum of the compatibility scores.
Problem
Approach
Steps
Complexity
Input: You are given two 2D arrays: one representing the students' answers and another representing the mentors' answers. Both arrays have m rows (one for each student and mentor) and n columns (one for each question's answer). Each entry in the arrays is either 0 or 1.
Example: students = [[1,1,0],[1,0,1],[0,0,1]], mentors = [[1,0,0],[0,0,1],[1,1,0]]
Constraints:
• m == students.length == mentors.length
• n == students[i].length == mentors[j].length
• 1 <= m, n <= 8
• students[i][k] is either 0 or 1
• mentors[j][k] is either 0 or 1
Output: Return the maximum sum of compatibility scores that can be achieved by optimally pairing students to mentors.
Example: Output: 8
Constraints:
• The output should be a single integer representing the maximum compatibility score sum.
Goal: The goal is to find the optimal pairings between students and mentors that maximize the sum of compatibility scores.
Steps:
• Step 1: For each student-mentor pairing, calculate the compatibility score by counting the number of matching answers.
• Step 2: Find the optimal pairing by checking all possible permutations of student-mentor assignments.
• Step 3: Sum the compatibility scores of the optimal pairings and return the result.
Goal: The problem involves finding the best student-mentor pairings using the given constraints on input size and values.
Steps:
• 1 <= m, n <= 8
• students and mentors contain only 0 or 1 values.
Assumptions:
• The input sizes are small enough (maximum 8 students/mentors and 8 questions) that checking all permutations is computationally feasible.
• Each student will be assigned to exactly one mentor, and vice versa.
• Input: Input: students = [[1,1,0],[1,0,1],[0,0,1]], mentors = [[1,0,0],[0,0,1],[1,1,0]]
• Explanation: The possible pairings are evaluated to find the one with the highest sum of compatibility scores. The optimal pairings in this case are student 0 with mentor 2 (compatibility score 3), student 1 with mentor 0 (score 2), and student 2 with mentor 1 (score 3). The total score is 8.
• Input: Input: students = [[0,0],[0,0],[0,0]], mentors = [[1,1],[1,1],[1,1]]
• Explanation: In this case, all compatibility scores are 0 because no student-answer matches any mentor-answer, so the total score is 0.
Approach: The approach is to explore all possible pairings between students and mentors, calculate the compatibility score for each pairing, and select the optimal combination that maximizes the total score.
Observations:
• Since m and n are relatively small (maximum of 8), brute-forcing through all possible permutations of student-mentor pairings is computationally feasible.
• The problem is essentially about finding the maximum matching between two sets (students and mentors) based on a compatibility function.
Steps:
• Step 1: Iterate through all possible permutations of students to mentors.
• Step 2: For each permutation, calculate the compatibility score by comparing the answers of the student and mentor.
• Step 3: Keep track of the highest compatibility score sum encountered.
• Step 4: Return the highest score as the result.
Empty Inputs:
• Empty student or mentor arrays are not valid inputs as per the problem constraints.
Large Inputs:
• Since m and n are capped at 8, the input size is small and does not require special handling for large inputs.
Special Values:
• When all answers are 0 or 1, ensure the compatibility scores are calculated correctly.
Constraints:
• Ensure that the input arrays are of size m x n and contain only 0 or 1 values.
int maxCompatibilitySum(vector<vector<int>>& students, vector<vector<int>>& mentors) {
vector<int> pos;
int ans = 0;
for(int i = 0; i < students.size(); i++)
pos.push_back(i);
do {
int curr = 0;
for(int i = 0; i < students.size(); i++)
for(int j = 0; j < students[pos[i]].size(); j++)
curr += (students[pos[i]][j] == mentors[i][j]);
ans = max(ans, curr);
} while(next_permutation(pos.begin(), pos.end()));
return ans;
}
1 : Function Definition
int maxCompatibilitySum(vector<vector<int>>& students, vector<vector<int>>& mentors) {
The function begins by accepting two parameters: 'students' and 'mentors', both of which are 2D vectors representing their respective compatibility values.
2 : Variable Initialization
vector<int> pos;
A vector 'pos' is initialized to store the indices of the students for permutation. This will help in exploring different student-mentor assignments.
3 : Variable Initialization
int ans = 0;
The variable 'ans' is initialized to 0. It will hold the maximum compatibility sum found through all permutations of student-mentor pairings.
4 : Loop over Students
for(int i = 0; i < students.size(); i++)
A loop is initiated to iterate over the students, used to generate the initial list of indices in 'pos'.
5 : Filling pos
pos.push_back(i);
Each student's index 'i' is added to the vector 'pos'. This will be used to explore different permutations of student-mentor assignments.
6 : Do-While Loop
do {
A do-while loop is used to iterate through all permutations of the student assignments (in 'pos') using the 'next_permutation' function.
7 : Permutation Evaluation
int curr = 0;
A temporary variable 'curr' is initialized to 0, which will store the current compatibility sum for this specific permutation of student-mentor pairings.
8 : Loop over Students
for(int i = 0; i < students.size(); i++)
The second loop iterates over the students, evaluating the compatibility of each student-mentor pair for the current permutation.
9 : Inner Loop over Attributes
for(int j = 0; j < students[pos[i]].size(); j++)
This nested loop iterates over the compatibility attributes of each student-mentor pair.
10 : Compatibility Check
curr += (students[pos[i]][j] == mentors[i][j]);
For each attribute, the compatibility of the student and mentor pair is checked. If they match, 'curr' is incremented by 1.
11 : Update Maximum Compatibility
ans = max(ans, curr);
After evaluating a permutation, 'ans' is updated to the maximum value between the current maximum compatibility sum and the compatibility sum of the current permutation.
12 : End of Permutation Evaluation
} while(next_permutation(pos.begin(), pos.end()));
The do-while loop continues until all permutations of the 'pos' vector have been evaluated.
13 : Return Statement
return ans;
Once all permutations have been evaluated, the maximum compatibility sum 'ans' is returned.
Best Case: O(m!)
Average Case: O(m!)
Worst Case: O(m!)
Description: Since we need to check all permutations of student-mentor pairings, the time complexity is factorial in m, i.e., O(m!).
Best Case: O(m)
Worst Case: O(m)
Description: The space complexity is linear in m, as we need space to store the current permutation of students and mentors.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus