Leetcode 1929: Concatenation of Array
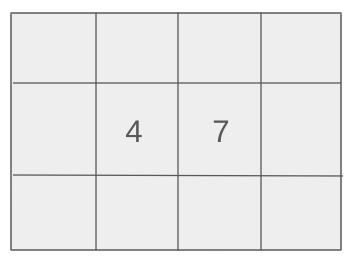
You are given an integer array nums and are required to return a new array where nums is repeated twice in a row.
Problem
Approach
Steps
Complexity
Input: The input consists of a single array nums of integers.
Example: nums = [3, 4, 5]
Constraints:
• 1 <= n <= 1000
• 1 <= nums[i] <= 1000
Output: Return the array where nums is concatenated with itself.
Example: [3, 4, 5, 3, 4, 5]
Constraints:
Goal: Concatenate the array nums with itself.
Steps:
• Create a new array ans with a length twice as long as nums.
• Copy the elements of nums into the first half of ans.
• Copy the elements of nums into the second half of ans.
Goal: Ensure that the result array is formed by concatenating nums with itself, and handle up to 1000 elements in the array.
Steps:
• The length of nums is at most 1000.
• Each element of nums is a positive integer.
Assumptions:
• nums contains positive integers.
• The result array will not exceed the constraints set by the problem.
• Input: nums = [3, 4, 5]
• Explanation: The array ans is formed by concatenating nums with itself: [3, 4, 5, 3, 4, 5].
Approach: The problem can be solved by creating a new array with twice the length of nums, then copying the elements of nums twice.
Observations:
• The array needs to be expanded to twice its size.
• The solution can be efficiently achieved with a time complexity of O(n).
• Concatenation can be done in a single pass using a for loop or array operations.
Steps:
• Create an array of size 2n.
• Copy elements from nums to the first half of the new array.
• Copy elements from nums to the second half of the new array.
Empty Inputs:
• If nums is empty, the output should also be an empty array.
Large Inputs:
• The solution should efficiently handle large arrays with the maximum length of 1000.
Special Values:
• Ensure that values in nums are handled properly when they are at the upper constraint of 1000.
Constraints:
• The time complexity of O(n) is sufficient to handle arrays with the maximum length.
vector<int> getConcatenation(vector<int>& nums) {
int n=nums.size();
for(int i=0;i<n;i++)
{
nums.push_back(nums[i]);
}
return nums;
}
1 : Function Header
vector<int> getConcatenation(vector<int>& nums) {
Defines the function `getConcatenation`, which takes a vector `nums` by reference and returns a vector of integers.
2 : Variable Declaration
int n=nums.size();
Calculates and stores the size of the input vector `nums` in the variable `n`.
3 : Loop Initialization
for(int i=0;i<n;i++)
Starts a loop that iterates through each element of the vector `nums` using the index `i`.
4 : Array Modification
nums.push_back(nums[i]);
Appends each element from the original vector `nums` to the end of the same vector, effectively duplicating its contents.
5 : Return Statement
return nums;
Returns the modified vector `nums`, which now contains its original contents followed by a concatenation of those contents.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n), where n is the length of the input array nums, because we iterate through nums twice.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n), as we create a new array of size 2n.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus