Leetcode 1913: Maximum Product Difference Between Two Pairs
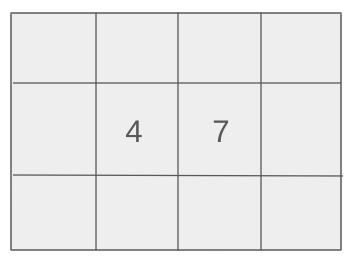
Given an integer array nums, your goal is to select four distinct indices w, x, y, and z such that the product difference between two pairs of numbers, (nums[w], nums[x]) and (nums[y], nums[z]), is maximized. The product difference is defined as (nums[w] * nums[x]) - (nums[y] * nums[z]). Return the maximum product difference between these two pairs.
Problem
Approach
Steps
Complexity
Input: The input consists of an array nums, where each element is a positive integer.
Example: nums = [5,6,2,7,4]
Constraints:
• 4 <= nums.length <= 10^4
• 1 <= nums[i] <= 10^4
Output: Return the maximum product difference between the two pairs.
Example: 34
Constraints:
Goal: To calculate the maximum product difference by finding the optimal pairs of elements.
Steps:
• Identify the two largest elements in the array and the two smallest elements in the array.
• Calculate the product difference using these values: (max1 * max2) - (min1 * min2).
Goal: Ensure that the solution handles the constraints efficiently for large inputs.
Steps:
• nums has at least 4 elements and at most 10^4 elements.
• Each element in nums is between 1 and 10^4.
Assumptions:
• The array has at least 4 elements, as the problem requires selecting four distinct indices.
• Input: nums = [5,6,2,7,4]
• Explanation: The maximum product difference is obtained by selecting indices 1 and 3 for the first pair (6, 7) and indices 2 and 4 for the second pair (2, 4). Thus, the product difference is (6 * 7) - (2 * 4) = 34.
Approach: To solve this problem, we need to identify the two largest and two smallest values in the array and then compute their product difference.
Observations:
• We need to find the largest and smallest elements efficiently.
• Using a single loop to identify the two largest and two smallest elements in the array would be the most efficient approach.
Steps:
• Initialize four variables: max1, max2, min1, and min2.
• Iterate through the array, updating these variables to track the two largest and two smallest elements.
• Calculate the product difference using the formula (max1 * max2) - (min1 * min2).
Empty Inputs:
• Handle cases where the input array has fewer than 4 elements, though this won't occur based on the problem constraints.
Large Inputs:
• Ensure that the solution works efficiently with arrays of size up to 10^4.
Special Values:
• Handle cases where all elements are the same or all are very large.
Constraints:
• Ensure that the solution handles the constraints efficiently.
int maxProductDifference(vector<int>& nums) {
//we have to return the result of
// (firstMax*secondMax) - (firstMin*secondMin)
int max1=INT_MIN;
int max2=INT_MIN;
int min1=INT_MAX;
int min2=INT_MAX;
for(int i=0;i<nums.size();i++)
{
if(nums[i]>max1)
{
//assign the second max to max2
max2=max1;
max1=nums[i];
}
else if(nums[i]>max2)
{
//it can become second max
max2= nums[i];
}
//check for the minimum
if(nums[i]<min1)
{
//it can become first minimum
min2=min1;
min1=nums[i];
}
else if(nums[i]<min2)
{
//it can become second minimum
min2=nums[i];
}
}
return (max1*max2)- (min1*min2);
}
1 : Function Signature
int maxProductDifference(vector<int>& nums) {
This is the function signature, defining a function called 'maxProductDifference' which takes a vector of integers as input.
2 : Variable Initialization
int max1=INT_MIN;
This initializes 'max1' to the smallest possible integer, which will hold the maximum value from the array.
3 : Variable Initialization
int max2=INT_MIN;
This initializes 'max2' to the smallest possible integer, which will hold the second maximum value from the array.
4 : Variable Initialization
int min1=INT_MAX;
This initializes 'min1' to the largest possible integer, which will hold the minimum value from the array.
5 : Variable Initialization
int min2=INT_MAX;
This initializes 'min2' to the largest possible integer, which will hold the second minimum value from the array.
6 : Loop
for(int i=0;i<nums.size();i++)
A for-loop iterates through each element of the 'nums' array to evaluate the maximum and minimum values.
7 : Condition
if(nums[i]>max1)
Checks if the current number is greater than 'max1' (the largest number found so far).
8 : Assignment
max2=max1;
Assigns the previous 'max1' to 'max2' because a new larger number has been found.
9 : Assignment
max1=nums[i];
Assigns the current number to 'max1' as it is now the largest number found.
10 : Condition
else if(nums[i]>max2)
Checks if the current number is greater than 'max2' but less than or equal to 'max1'.
11 : Assignment
max2= nums[i];
Assigns the current number to 'max2' as it is now the second largest number.
12 : Condition
if(nums[i]<min1)
Checks if the current number is smaller than 'min1' (the smallest number found so far).
13 : Assignment
min2=min1;
Assigns the previous 'min1' to 'min2' because a new smaller number has been found.
14 : Assignment
min1=nums[i];
Assigns the current number to 'min1' as it is now the smallest number found.
15 : Condition
else if(nums[i]<min2)
Checks if the current number is smaller than 'min2' but greater than or equal to 'min1'.
16 : Assignment
min2=nums[i];
Assigns the current number to 'min2' as it is now the second smallest number.
17 : Return
return (max1*max2)- (min1*min2);
Returns the product difference between the largest two numbers and the smallest two numbers.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is linear because we are iterating through the array once to find the two largest and two smallest values.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is constant because we are only using a fixed amount of extra space for tracking the four values.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus