Leetcode 1911: Maximum Alternating Subsequence Sum
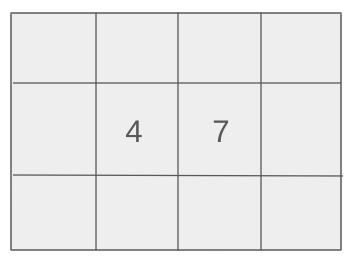
You are given an array nums. The alternating sum of a sequence is calculated as the sum of elements at even indices minus the sum of elements at odd indices. Your task is to find the maximum alternating sum of any subsequence formed by deleting some elements from the array without changing the relative order of the remaining elements.
Problem
Approach
Steps
Complexity
Input: The input consists of an array nums, where each element is a positive integer.
Example: nums = [4,2,5,3]
Constraints:
• 1 <= nums.length <= 10^5
• 1 <= nums[i] <= 10^5
Output: Return the maximum alternating sum that can be achieved from any subsequence of nums.
Example: 7
Constraints:
Goal: To calculate the maximum alternating sum of any subsequence of nums.
Steps:
• Use dynamic programming to track the maximum alternating sum at each index, considering both including and excluding the current element.
Goal: Ensure the solution handles the constraints efficiently for large inputs.
Steps:
• nums has at least 1 element and at most 10^5 elements.
• Each element in nums is between 1 and 10^5.
Assumptions:
• The elements of the array are positive integers.
• Input: nums = [4,2,5,3]
• Explanation: The optimal subsequence to pick is [4, 2, 5], resulting in an alternating sum of (4 + 5) - 2 = 7.
Approach: The problem can be approached using dynamic programming where we maintain two variables: one for the maximum sum when considering the current element and another for the sum when excluding it.
Observations:
• The alternating sum involves switching between adding and subtracting elements.
• We can use a greedy dynamic approach to decide at each step whether to include or exclude the current element.
Steps:
• Initialize two variables: odd and even, where 'odd' stores the max sum considering the current element as part of the odd index, and 'even' stores the max sum considering it as part of the even index.
• Iterate through the array nums and update the variables accordingly.
• Return the value stored in the 'even' variable as it will represent the maximum alternating sum.
Empty Inputs:
• If nums is empty, handle gracefully by returning 0.
Large Inputs:
• Ensure the solution works efficiently with arrays of size up to 10^5.
Special Values:
• Handle cases where all elements are the same, as well as where all elements are very large or small.
Constraints:
• Ensure the solution handles the constraints efficiently.
long long maxAlternatingSum(vector<int>& nums) {
long long odd = 0, even = 0;
for(int num : nums) {
even = max(even, odd + num);
odd = even - num;
}
return even;
}
1 : Function Definition
long long maxAlternatingSum(vector<int>& nums) {
Define the function 'maxAlternatingSum' which takes a reference to a vector of integers 'nums'. The function will return a long long value representing the maximum alternating sum.
2 : Variable Initialization
long long odd = 0, even = 0;
Initialize two variables 'odd' and 'even' to store the maximum alternating sums for odd and even indices in the sequence, respectively.
3 : For Loop Setup
for(int num : nums) {
Start a loop to iterate through each element 'num' in the vector 'nums'.
4 : Dynamic Programming Update
even = max(even, odd + num);
Update the 'even' sum by considering the maximum of the previous 'even' value and the sum of 'odd' plus the current 'num'. This ensures we alternate between adding and subtracting the elements.
5 : Odd Sum Update
odd = even - num;
Update the 'odd' sum by subtracting the current 'num' from 'even', following the alternating sum pattern.
6 : Return Statement
return even;
Return the 'even' value as the result, representing the maximum alternating sum after considering all numbers.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is linear because we are iterating through the array once.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is constant as we are only using a few extra variables for tracking the sums.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus