Leetcode 1906: Minimum Absolute Difference Queries
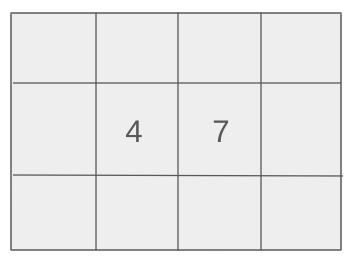
Given an array of integers
nums
and a list of queries, where each query is defined as a subarray nums[li...ri]
, compute the minimum absolute difference between any two distinct elements in that subarray. If all elements in the subarray are the same, return -1.Problem
Approach
Steps
Complexity
Input: An array `nums` of integers and an array of queries where each query is represented as a pair of integers `[li, ri]`.
Example: nums = [1, 3, 4, 8], queries = [[0,1], [1,2], [2,3], [0,3]]
Constraints:
• 2 <= nums.length <= 10^5
• 1 <= nums[i] <= 100
• 1 <= queries.length <= 2 * 10^4
• 0 <= li < ri < nums.length
Output: Return an array of integers where each element corresponds to the minimum absolute difference for the respective query.
Example: [2, 1, 4, 1]
Constraints:
• Each element of the output should be the minimum absolute difference for the respective subarray from the queries list.
Goal: For each query, extract the subarray and compute the minimum absolute difference by comparing all unique pairs of elements.
Steps:
• For each query, slice the array from index li to ri.
• Find the minimum absolute difference between distinct elements in that subarray.
• If all elements are the same, return -1.
Goal: The input size constraints ensure the algorithm should be efficient, especially in the case of large arrays and numerous queries.
Steps:
• The algorithm should handle up to 10^5 elements in the array and 2 * 10^4 queries efficiently.
Assumptions:
• All numbers in `nums` are positive integers.
• The query indices are valid within the bounds of the array.
• Input: Input: nums = [1, 3, 4, 8], queries = [[0,1], [1,2], [2,3], [0,3]]
• Explanation: The subarrays for each query are processed to calculate the minimum absolute difference for distinct elements.
Approach: We can efficiently calculate the minimum absolute difference for each query by leveraging the frequency of each element within the subarrays.
Observations:
• Iterating through each query and calculating the minimum difference might take time for large arrays.
• We need a strategy to process the queries efficiently.
• Precomputing frequency counts of numbers within subarrays can help optimize the solution.
Steps:
• Compute the frequency of each number in `nums` and maintain a prefix frequency array.
• For each query, use the prefix frequency array to extract the frequencies for the relevant subarray.
• Find the minimum absolute difference by comparing the smallest non-zero frequency differences.
Empty Inputs:
• Queries with no valid subarrays or an empty array.
Large Inputs:
• Handling cases with the maximum array size and query count.
Special Values:
• When all elements in a subarray are the same, the answer should be -1.
Constraints:
• Ensure the solution runs efficiently with the upper limits of constraints.
vector<int> minDifference(vector<int>& nums, vector<vector<int>>& q) {
int prefix[100001][101] = {}, cnt[101] = {};
int n = nums.size(), m = q.size();
for(int i = 0; i < n; i++) {
cnt[nums[i]]++;
for(int j = 1; j < 101; j++) prefix[i + 1][j] = cnt[j];
}
vector<int> ans;
for(int i = 0; i < m; i++) {
int l = q[i][0], r = q[i][1];
int frq[101] = {};
for(int j = 1; j < 101; j++) frq[j] = prefix[r + 1][j] - prefix[l][j];
int prv = -1, mn = INT_MAX;
for(int j = 1; j < 101; j++) {
if(frq[j] == 0) continue;
if(prv != -1 && j - prv < mn) mn = j - prv;
prv = j;
}
ans.push_back(mn == INT_MAX? -1: mn);
}
return ans;
}
1 : Function Declaration
vector<int> minDifference(vector<int>& nums, vector<vector<int>>& q) {
Declares the function 'minDifference', which takes a vector of integers 'nums' and a 2D vector 'q' representing multiple queries.
2 : Prefix Array Initialization
int prefix[100001][101] = {}, cnt[101] = {};
Initializes a 2D prefix sum array 'prefix' to store frequencies up to index 'i', and an array 'cnt' to count occurrences of each number.
3 : Size Determination
int n = nums.size(), m = q.size();
Stores the sizes of the 'nums' and 'q' arrays, where 'n' is the length of 'nums' and 'm' is the number of queries.
4 : Prefix Calculation
for(int i = 0; i < n; i++) {
Iterates through the 'nums' array to calculate the frequency of each number.
5 : Count Update
cnt[nums[i]]++;
Increments the count of the number at index 'i' in the 'nums' array.
6 : Prefix Update
for(int j = 1; j < 101; j++) prefix[i + 1][j] = cnt[j];
Updates the 'prefix' array to store the cumulative frequency counts for each number up to index 'i'.
7 : Query Processing
vector<int> ans;
Initializes a vector 'ans' to store the results for each query.
8 : Query Iteration
for(int i = 0; i < m; i++) {
Iterates through each query in the 2D array 'q'.
9 : Range Initialization
int l = q[i][0], r = q[i][1];
Extracts the left and right range indices for the current query.
10 : Frequency Calculation
int frq[101] = {};
Initializes an array 'frq' to store the frequency of each number within the current query range.
11 : Frequency Update
for(int j = 1; j < 101; j++) frq[j] = prefix[r + 1][j] - prefix[l][j];
Calculates the frequency of each number in the range [l, r] by using the 'prefix' sum array.
12 : Variable Initialization
int prv = -1, mn = INT_MAX;
Initializes variables 'prv' to track the previous number and 'mn' to store the minimum difference between two numbers.
13 : Finding Minimum Difference
for(int j = 1; j < 101; j++) {
Iterates through the frequency array 'frq' to find the minimum difference between non-zero frequencies.
14 : Skip Zero Frequencies
if(frq[j] == 0) continue;
Skips the number if its frequency is zero.
15 : Minimum Difference Update
if(prv != -1 && j - prv < mn) mn = j - prv;
Updates the minimum difference 'mn' if the difference between 'j' and 'prv' is smaller than the current 'mn'.
16 : Update Previous Value
prv = j;
Updates the previous number to the current number 'j'.
17 : Result Handling
ans.push_back(mn == INT_MAX? -1: mn);
Pushes the result of the current query into the 'ans' vector. If no valid difference is found, it pushes -1.
18 : Return Statement
return ans;
Returns the vector 'ans' containing the results for all queries.
Best Case: O(1) when there is only one query and the subarray has all identical elements.
Average Case: O(n + m), where n is the length of the array and m is the number of queries.
Worst Case: O(n * m), considering the worst-case scenario for large input sizes.
Description: The solution processes each query independently after preprocessing the frequency data.
Best Case: O(1) when queries and subarrays are minimal.
Worst Case: O(n + 100) for the prefix frequency array and the result storage.
Description: The space complexity is primarily driven by the frequency array used for storing the counts of the elements.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus