Leetcode 1863: Sum of All Subset XOR Totals
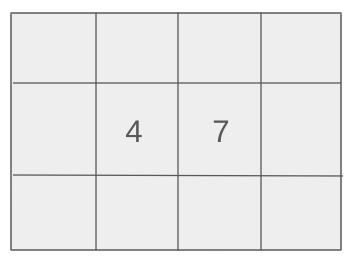
You are given an array of integers
nums
. The XOR total of an array is defined as the bitwise XOR of all its elements, or 0 if the array is empty. For every subset of nums
, calculate the XOR total and return the sum of all XOR totals.Problem
Approach
Steps
Complexity
Input: The input is an array `nums` consisting of integers.
Example: nums = [1, 3]
Constraints:
• 1 <= nums.length <= 12
• 1 <= nums[i] <= 20
Output: The output is an integer representing the sum of all XOR totals for every subset of the array `nums`.
Example: Output = 6
Constraints:
• The output is the sum of XOR totals for every subset of the array.
Goal: The goal is to calculate the XOR total for every subset and return their sum.
Steps:
• Step 1: Generate all subsets of the array.
• Step 2: For each subset, calculate the XOR total by performing a bitwise XOR of all elements in the subset.
• Step 3: Add the XOR totals of all subsets and return the sum.
Goal: The constraints ensure the array is small enough for generating all subsets and calculating XOR totals efficiently.
Steps:
• The array contains between 1 and 12 elements.
• Each integer in the array is between 1 and 20.
Assumptions:
• The input array contains at least one element.
• Input: Input: [1, 3]
• Explanation: There are 4 subsets: the empty subset, [1], [3], and [1,3]. The XOR totals for each subset are 0, 1, 3, and 2, respectively. The sum of these totals is 6.
• Input: Input: [4, 1, 7]
• Explanation: The eight subsets of [4, 1, 7] and their corresponding XOR totals are: 0, 4, 1, 7, 5, 3, 6, and 2. The sum of these totals is 35.
Approach: To solve this problem, we generate all possible subsets of the array and compute the XOR total for each subset. Then, we sum all these XOR totals.
Observations:
• The problem requires generating all subsets and computing XOR totals for each subset.
• Given that the number of elements in the array is small (at most 12), generating all subsets (2^n subsets) is feasible.
Steps:
• Step 1: Loop through all numbers from 1 to 2^n - 1, where n is the size of the array. Each number represents a subset using its binary representation.
• Step 2: For each subset, calculate the XOR total by checking which elements are included (using the binary number) and performing XOR.
• Step 3: Sum all the XOR totals and return the result.
Empty Inputs:
• The input array is never empty as per the constraints.
Large Inputs:
• The maximum length of the array is 12, so generating subsets and calculating XOR totals will work efficiently within the time limits.
Special Values:
• Consider cases where all elements are the same, which will result in subsets with identical XOR totals.
Constraints:
• The array contains between 1 and 12 elements, each between 1 and 20.
int subsetXORSum(vector<int>& nums) {
int res = 0;
for (auto i = 1; i < (1 << nums.size()); ++i) {
int total = 0;
for (auto j = 0; j < nums.size(); ++j)
if (i & (1 << j))
total ^= nums[j];
res += total;
}
return res;
}
1 : Function Definition
int subsetXORSum(vector<int>& nums) {
Define the function `subsetXORSum`, which takes a reference to a vector `nums` containing integers and returns an integer.
2 : Variable Initialization
int res = 0;
Initialize a variable `res` to store the cumulative XOR sum of all subsets.
3 : Outer Loop (Subset Generation)
for (auto i = 1; i < (1 << nums.size()); ++i) {
Start a loop to iterate through all possible subsets of `nums` by generating numbers from 1 to `2^n - 1` using bitwise operations.
4 : Variable Initialization (Subset XOR)
int total = 0;
Initialize a variable `total` to store the XOR of the current subset.
5 : Inner Loop (Element Check)
for (auto j = 0; j < nums.size(); ++j)
Start a loop to iterate through each element of the `nums` array to check if the current element belongs to the current subset.
6 : Bitwise Check
if (i & (1 << j))
Check if the `j`-th element is included in the current subset by using a bitwise AND operation. If the `j`-th bit of `i` is set, the element is included.
7 : Subset XOR Calculation
total ^= nums[j];
XOR the value of the `j`-th element of `nums` with the current `total` to update the XOR for the current subset.
8 : Update Result
res += total;
Add the XOR result of the current subset (`total`) to the running total `res`.
9 : Return Result
return res;
Return the final result `res`, which contains the sum of XORs of all subsets.
Best Case: O(2^n * n)
Average Case: O(2^n * n)
Worst Case: O(2^n * n)
Description: The time complexity is O(2^n * n), where n is the length of the input array. There are 2^n subsets and for each subset, we need to compute the XOR total which takes O(n) time.
Best Case: O(2^n)
Worst Case: O(2^n)
Description: The space complexity is O(2^n) due to the space needed for storing the subsets and the XOR totals.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus