Leetcode 1848: Minimum Distance to the Target Element
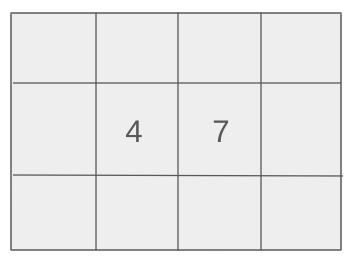
You are given an array nums, along with two integers target and start. Your task is to find an index i such that nums[i] == target, and the absolute difference between i and start is minimized. Return abs(i - start), where abs(x) is the absolute value of x.
Problem
Approach
Steps
Complexity
Input: The input consists of an integer array nums, an integer target, and an integer start.
Example: [10, 20, 30, 40, 50], target = 40, start = 2
Constraints:
• 1 <= nums.length <= 1000
• 1 <= nums[i] <= 10^4
• 0 <= start < nums.length
• target is in nums
Output: Return the minimum absolute difference abs(i - start) for the index i where nums[i] == target.
Example: 1
Constraints:
Goal: The goal is to find the index of target in nums that minimizes the absolute difference with the start index.
Steps:
• Iterate through the array to find all indices where nums[i] equals target.
• Calculate the absolute difference between each index and the start index.
• Return the minimum of these differences.
Goal: The input size and value constraints ensure the problem is manageable in terms of performance.
Steps:
• 1 <= nums.length <= 1000
• 1 <= nums[i] <= 10^4
• 0 <= start < nums.length
• target is guaranteed to exist in nums.
Assumptions:
• The input array contains at least one element.
• The target value is guaranteed to be present in the array.
• Input: [10, 20, 30, 40, 50], target = 40, start = 2
• Explanation: The element 40 is located at index 3, and the minimum distance to the start index (2) is 1.
Approach: The approach involves iterating through the array to find all indices where nums[i] equals target and then calculating the minimum distance between the indices and the start index.
Observations:
• Iterating through the array once is sufficient to find all occurrences of target.
• By keeping track of the minimum distance while iterating, we can achieve an optimal solution.
Steps:
• Initialize a variable to store the minimum distance (initially set to a large value).
• Iterate over the array and check for occurrences of target.
• For each occurrence, calculate the absolute difference between the current index and the start index.
• Update the minimum distance if the calculated distance is smaller.
• Return the minimum distance after the loop.
Empty Inputs:
• The array will never be empty, as the input length is guaranteed to be at least 1.
Large Inputs:
• Ensure that the algorithm handles arrays with the maximum length of 1000 efficiently.
Special Values:
• Consider arrays where the target appears multiple times, or where the target is at the start or end of the array.
Constraints:
• The approach needs to efficiently handle arrays of size up to 1000.
int getMinDistance(vector<int>& nums, int target, int start) {
int res = INT_MAX;
for (int i = 0; i < nums.size() && res > abs(start - i); ++i)
if (nums[i] == target)
res = abs(start - i);
return res;
}
1 : Function Definition
int getMinDistance(vector<int>& nums, int target, int start) {
Defines the function `getMinDistance`, which takes a vector `nums`, a target value, and a start index, returning the minimum distance to the target.
2 : Variable Initialization
int res = INT_MAX;
Initializes a variable `res` to store the minimum distance, starting with the maximum possible value, `INT_MAX`.
3 : Loop Initialization
for (int i = 0; i < nums.size() && res > abs(start - i); ++i)
Begins a loop that iterates through the array `nums`. It also ensures that the loop continues until the distance becomes smaller than `res`.
4 : Condition Check
if (nums[i] == target)
Checks if the current element in the array `nums[i]` is equal to the `target`.
5 : Distance Update
res = abs(start - i);
Updates the value of `res` with the absolute difference between the current index `i` and the start index, which represents the distance.
6 : Return Statement
return res;
Returns the minimum distance found during the iteration.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is linear, O(n), where n is the length of the array, as we need to check each element in the array.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is constant, O(1), as we only need a few variables to track the minimum distance.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus