Leetcode 1846: Maximum Element After Decreasing and Rearranging
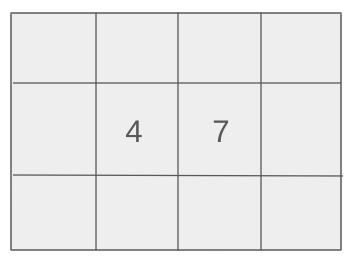
You are given an array of positive integers arr. Perform a series of operations (possibly none) on arr to make it satisfy the following conditions: The value of the first element in arr must be 1, and the absolute difference between any two adjacent elements must be less than or equal to 1. You can decrease the value of any element and rearrange the elements in any order. Return the maximum possible value of an element in arr after performing the operations.
Problem
Approach
Steps
Complexity
Input: You are given an array of positive integers. The operations allowed are to rearrange the array and decrease the value of any element.
Example: [3, 2, 1, 3, 1]
Constraints:
• 1 <= arr.length <= 10^5
• 1 <= arr[i] <= 10^9
Output: Return the maximum value of an element in the array after performing the operations that satisfy the given conditions.
Example: 3
Constraints:
Goal: The goal is to rearrange and possibly decrease values in the array to ensure the first element is 1 and the absolute difference between adjacent elements is less than or equal to 1.
Steps:
• Sort the array to make the smallest elements come first.
• Set the first element to 1 if it's not already.
• For each element after the first, ensure that the difference between the current element and the previous one is no greater than 1. If it is, decrease the current element to be one greater than the previous element.
• The largest element after modifications will be the result.
Goal: The input array must have a size between 1 and 100,000, and the values in the array must be between 1 and 1 billion.
Steps:
• 1 <= arr.length <= 10^5
• 1 <= arr[i] <= 10^9
Assumptions:
• The input array is guaranteed to contain positive integers.
• The array will have at least one element.
• Input: [3, 2, 1, 3, 1]
• Explanation: After rearranging and adjusting the values, the largest element in the array is 3.
Approach: The solution approach is to first sort the array to make it easier to adjust the elements and then iteratively decrease elements where necessary while ensuring that adjacent elements differ by no more than 1.
Observations:
• Sorting the array simplifies the process of making adjustments between adjacent elements.
• By iterating over the sorted array and adjusting elements that violate the absolute difference condition, we can efficiently arrive at the correct answer.
Steps:
• Sort the array.
• If the first element is not 1, set it to 1.
• Iterate through the array and for each element, make sure the difference between adjacent elements is no more than 1.
• Return the largest element after the operations.
Empty Inputs:
• There are no empty inputs, as the array always has at least one element.
Large Inputs:
• For large inputs, ensure that the sorting and adjustments are efficient enough to handle up to 100,000 elements.
Special Values:
• Consider arrays where all elements are the same or where they are already in a sequence that satisfies the conditions.
Constraints:
• The operations should be efficient to handle arrays of length up to 100,000.
int maximumElementAfterDecrementingAndRearranging(vector<int>& arr) {
sort(arr.begin(), arr.end());
if(arr[0] != 1) arr[0] = 1;
int n = arr.size();
for(int i = 1; i < n; i++) {
if(arr[i] - arr[i - 1] > 1) arr[i] = arr[i - 1] + 1;
}
return arr[n - 1];
}
1 : Function Definition
int maximumElementAfterDecrementingAndRearranging(vector<int>& arr) {
Defines the function `maximumElementAfterDecrementingAndRearranging`, which accepts a vector `arr` and returns an integer.
2 : Sorting
sort(arr.begin(), arr.end());
Sorts the array `arr` in ascending order.
3 : Condition Check
if(arr[0] != 1) arr[0] = 1;
Checks if the first element in the sorted array is not 1, and if so, sets it to 1.
4 : Array Size Calculation
int n = arr.size();
Calculates the size of the array `arr` and stores it in the variable `n`.
5 : Loop Start
for(int i = 1; i < n; i++) {
Starts a loop from the second element in the array to the end.
6 : Condition Check Inside Loop
if(arr[i] - arr[i - 1] > 1) arr[i] = arr[i - 1] + 1;
Checks if the difference between the current element and the previous element is greater than 1. If so, it adjusts the current element to be one greater than the previous element.
7 : Return Statement
return arr[n - 1];
Returns the last element in the array, which is now the maximum after adjustments.
Best Case: O(n log n)
Average Case: O(n log n)
Worst Case: O(n log n)
Description: The time complexity is dominated by the sorting step, which takes O(n log n), where n is the length of the array.
Best Case: O(1)
Worst Case: O(n)
Description: The space complexity is O(n) due to the space required for sorting the array.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus