Leetcode 1838: Frequency of the Most Frequent Element
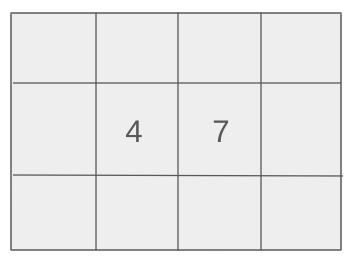
You are given an integer array nums and an integer k. In one operation, you can increment any element of the array by 1. Your task is to return the maximum possible frequency of any element after performing at most k operations.
Problem
Approach
Steps
Complexity
Input: The input consists of an array nums containing integers and an integer k representing the maximum number of operations allowed.
Example: nums = [3, 5, 6], k = 5
Constraints:
• 1 <= nums.length <= 10^5
• 1 <= nums[i] <= 10^5
• 1 <= k <= 10^5
Output: The output is the maximum frequency of any element in the array after performing at most k operations.
Example: 3
Constraints:
Goal: To maximize the frequency of any element in the array by performing at most k operations.
Steps:
• Sort the array to easily calculate the number of increments needed to make elements equal.
• Use a sliding window to calculate the number of operations required to make the elements in the window equal.
• Maximize the frequency by checking the number of elements that can be converted to the same value using the available operations.
Goal: The input values nums and k are constrained to ensure that the problem can be solved efficiently.
Steps:
• 1 <= nums.length <= 10^5
• 1 <= nums[i] <= 10^5
• 1 <= k <= 10^5
Assumptions:
• The array contains at least one element.
• k is the total number of allowed operations, and it is always greater than or equal to 1.
• Input: nums = [3, 5, 6], k = 5
• Explanation: We first sort the array to get [3, 5, 6]. Then we can increment 3 by 2 and 5 by 3 to make them equal to 5, resulting in a frequency of 3 for 5.
Approach: To maximize the frequency of an element, we will use a sliding window technique. By sorting the array and keeping track of the total operations needed to make the elements equal, we can find the maximum frequency.
Observations:
• Sorting the array will make it easier to check the operations required for each element.
• The sliding window approach will help efficiently compute the number of operations needed for different ranges of elements.
Steps:
• Sort the input array.
• Initialize a window and track the number of operations needed to make all elements in the window equal.
• Use the window to maximize the frequency of the elements by incrementing them up to k times.
Empty Inputs:
• An empty input array is not valid based on the constraints.
Large Inputs:
• Ensure that the solution is efficient enough to handle large arrays up to size 10^5.
Special Values:
• If k is very small compared to the differences between elements, the maximum frequency will be limited.
Constraints:
• The array should always contain at least one element, and k should be positive.
int maxFrequency(vector<int>& A, int t) {
int i = 0, j;
long k = t;
sort(A.begin(), A.end());
for (j = 0; j < A.size(); ++j) {
k += A[j];
if (k < (long)A[j] * (j - i + 1))
k -= A[i++];
}
return j - i;
}
1 : Function Definition
int maxFrequency(vector<int>& A, int t) {
Defines the function `maxFrequency` which takes a vector `A` representing the elements and an integer `t` representing the budget, and returns the maximum frequency of elements that can be purchased without exceeding the budget.
2 : Variable Initialization
int i = 0, j;
Initializes the variables `i` and `j` for the sliding window. `i` is the start of the window, and `j` will iterate through the vector `A`.
3 : Budget Initialization
long k = t;
Initializes the budget `k` with the value of `t`. This will be updated as the algorithm processes the elements of `A`.
4 : Sorting
sort(A.begin(), A.end());
Sorts the vector `A` in ascending order to allow the sliding window approach to work efficiently by trying to add the smallest elements first.
5 : For Loop
for (j = 0; j < A.size(); ++j) {
Starts a for loop where `j` iterates over the sorted elements in vector `A`. This loop will explore the possible subsets of elements that can be purchased with the available budget.
6 : Update Budget
k += A[j];
Adds the current element `A[j]` to the budget `k`. This simulates spending money on the current item.
7 : Check Feasibility
if (k < (long)A[j] * (j - i + 1))
Checks if the total budget `k` is less than the total cost of the current subset of elements, which is calculated as the current element `A[j]` multiplied by the number of elements in the window `(j - i + 1)`. If this condition is true, it means the subset cannot be purchased with the budget.
8 : Adjust Window
k -= A[i++];
If the budget is exceeded, adjusts the window by removing the first element `A[i]` from the budget and moving the start of the window `i` to the next element.
9 : Return Result
return j - i;
Returns the difference between `j` and `i`, which represents the number of elements in the largest valid subset that can be purchased within the budget.
Best Case: O(n log n)
Average Case: O(n log n)
Worst Case: O(n log n)
Description: The time complexity is dominated by the sorting step, which is O(n log n), where n is the size of the array.
Best Case: O(1)
Worst Case: O(n)
Description: The space complexity is O(n) for storing the sorted array in the worst case.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus