Leetcode 1832: Check if the Sentence Is Pangram
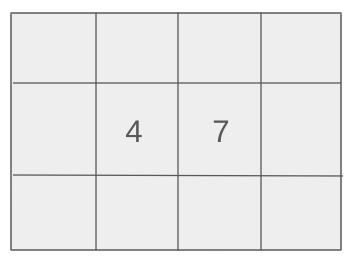
Given a string
sentence
consisting of lowercase English letters, determine whether the sentence contains every letter of the English alphabet at least once.Problem
Approach
Steps
Complexity
Input: The input consists of a string `sentence` containing only lowercase English letters.
Example: sentence = "abcdefghijklmnopqrstuvwxyz"
Constraints:
• 1 <= sentence.length <= 1000
• sentence consists of lowercase English letters.
Output: Return `true` if the sentence is a pangram, otherwise return `false`.
Example: true
Constraints:
• The output will be a boolean value indicating whether the sentence is a pangram.
Goal: Check if all the letters of the alphabet are present in the given sentence.
Steps:
• Initialize a set or bitmask to track the unique letters in the sentence.
• Iterate through each character in the sentence and update the set or bitmask.
• If all 26 letters are present, return `true`; otherwise, return `false`.
Goal: The string `sentence` must be between 1 and 1000 characters long, and it will only contain lowercase English letters.
Steps:
• 1 <= sentence.length <= 1000
• sentence consists only of lowercase English letters.
Assumptions:
• The string `sentence` contains lowercase English letters and is not empty.
• The sentence length is within the given constraint.
• Input: sentence = "abcdefghijklmnopqrstuvwxyz"
• Explanation: The sentence contains every letter from 'a' to 'z', so the output is `true`.
• Input: sentence = "hello"
• Explanation: The sentence does not contain every letter of the alphabet, so the output is `false`.
Approach: To solve this problem, we will check if the sentence contains every letter of the alphabet using a set or bitmask.
Observations:
• The problem can be efficiently solved using a set or bitmask to track the presence of each letter.
• We can utilize a bitmask of 26 bits to represent each letter of the alphabet. Each bit corresponds to a letter, and if the bit is set, it means that letter is present in the sentence.
Steps:
• Initialize a bitset of size 26 to track the letters found in the sentence.
• Loop through each character in the sentence and mark the corresponding bit in the bitset.
• After processing all characters, check if all 26 bits are set. If they are, return `true`; otherwise, return `false`.
Empty Inputs:
• The sentence will always have at least one character, based on the constraints.
Large Inputs:
• For large inputs, the solution should efficiently check for the presence of all alphabet letters.
Special Values:
• If the sentence is too short to contain all 26 letters, the result will be `false`.
Constraints:
• Ensure the solution works efficiently within the provided input size constraints.
bool checkIfPangram(string set) {
bitset<26> bit;
for(char x: set) bit.set(x - 'a');
return bit.count() == 26;
}
1 : Function Definition
bool checkIfPangram(string set) {
Defines the function `checkIfPangram`, which takes a string `set` as input and returns a boolean indicating whether the string contains all 26 letters of the alphabet.
2 : Bitset Initialization
bitset<26> bit;
Initializes a bitset of size 26, where each bit represents one of the 26 letters of the alphabet. A set bit indicates the presence of that letter.
3 : Loop Through Characters
for(char x: set) bit.set(x - 'a');
Loops through each character `x` in the string `set` and sets the corresponding bit in the bitset. The expression `x - 'a'` maps each letter to an index between 0 and 25.
4 : Bitset Count Check
return bit.count() == 26;
Checks if all 26 bits in the bitset are set. If all bits are set (i.e., all letters are present), the count will be 26, and the function returns `true`; otherwise, it returns `false`.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n), where n is the length of the sentence. We only need one pass through the sentence to check for the presence of all letters.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is O(1) since we are only using a fixed-size bitset or set for tracking the letters, regardless of the sentence length.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus