Leetcode 1827: Minimum Operations to Make the Array Increasing
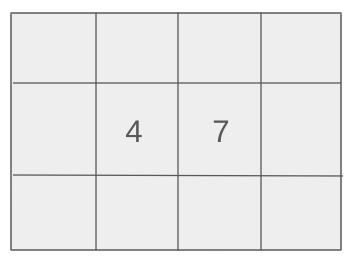
You are given an integer array nums. In one operation, you can increment any element of the array by 1. The goal is to make the array strictly increasing, meaning that each element should be smaller than the next. Return the minimum number of operations required to achieve this.
Problem
Approach
Steps
Complexity
Input: You are given an integer array nums of length n where 1 <= n <= 5000 and 1 <= nums[i] <= 10^4.
Example: For example, nums = [2, 3, 1, 5] means the array consists of four elements: 2, 3, 1, and 5.
Constraints:
• 1 <= nums.length <= 5000
• 1 <= nums[i] <= 10^4
Output: Return an integer, which is the minimum number of operations needed to make the array strictly increasing.
Example: For nums = [2, 3, 1, 5], the output is 2 because we need to increment nums[2] (1) to 4 and nums[3] (5) to 6 to make the array strictly increasing.
Constraints:
• The result should be a non-negative integer.
Goal: The goal is to compute the minimum number of increment operations to make the array strictly increasing.
Steps:
• 1. Iterate over the array starting from the second element.
• 2. If an element is not greater than the previous one, calculate the number of increments needed to make it strictly larger.
• 3. Keep track of the total number of operations (increments).
Goal: The array must be adjusted such that each element is strictly smaller than the next. The minimum number of operations required should be computed efficiently.
Steps:
• The array must contain at least one element.
• The elements of the array can range from 1 to 10,000.
Assumptions:
• If the array is already strictly increasing, no operations are needed.
• The solution should handle arrays with both small and large values efficiently.
• Input: Input: nums = [2, 3, 1, 5]
• Explanation: The array is not strictly increasing because 1 is not greater than 3. To make it strictly increasing, we need to increment 1 to 4 and 5 to 6. This results in 2 operations.
• Input: Input: nums = [1, 2, 3]
• Explanation: The array is already strictly increasing, so no operations are needed.
• Input: Input: nums = [8, 7, 5, 5]
• Explanation: The array is not strictly increasing because the elements 7 and 5 are not greater than the preceding elements. We need to increment 7 to 9 and 5 to 10 to make the array strictly increasing. This results in 4 operations.
Approach: The problem can be solved by iterating through the array and ensuring that each element is strictly greater than the previous one by applying the necessary number of increments.
Observations:
• The key observation is that for any element nums[i], it must be greater than nums[i-1]. If it is not, the difference between nums[i-1] and nums[i] tells us how many increments are needed.
• The problem involves minimizing the number of increments, so we should compute the required increments in a single pass.
• The solution is linear in complexity, processing each element in the array once. This ensures that we can solve the problem efficiently even for large arrays.
Steps:
• 1. Initialize a variable to keep track of the total number of increments.
• 2. Iterate through the array starting from the second element.
• 3. For each element, if it's not greater than the previous one, compute the required increments to make it larger.
• 4. Add the required increments to the total count and continue to the next element.
• 5. Return the total number of increments.
Empty Inputs:
• The array has only one element (no operation needed).
Large Inputs:
• When the array has the maximum length of 5000 elements, the solution must handle this efficiently with linear time complexity.
Special Values:
• Arrays where all elements are the same or where the array is already strictly increasing.
Constraints:
• Ensure that the solution handles arrays with large values and ensures efficiency with O(n) time complexity.
int minOperations(vector<int>& nums) {
int res = 0, need = nums[0] + 1;
int n = nums.size();
for(int i = 1; i < n; i++) {
res += max(0, need - nums[i]);
need = max(nums[i], need) + 1;
}
return res;
}
1 : Function Definition
int minOperations(vector<int>& nums) {
This defines the main function `minOperations`, which takes a vector of integers as input and computes the minimum operations needed to make the array strictly increasing.
2 : Variable Initialization
int res = 0, need = nums[0] + 1;
Here, `res` is initialized to 0 to store the number of operations, and `need` is set to one more than the first element in the array, representing the smallest required value for the next element.
3 : Array Size Calculation
int n = nums.size();
This line calculates the size of the `nums` array to determine the number of iterations needed for the loop.
4 : Loop Setup
for(int i = 1; i < n; i++) {
A for-loop starts from the second element (index 1) to iterate through the array and adjust the values to ensure it is strictly increasing.
5 : Operation Calculation
res += max(0, need - nums[i]);
This line computes the number of operations needed to adjust the current element (`nums[i]`). If the element is less than `need`, the difference is added to `res`.
6 : Updating Need
need = max(nums[i], need) + 1;
The `need` value is updated to be the maximum of the current element and the previous `need` value, then incremented by 1. This ensures that the next element will be strictly greater than the current one.
7 : Return Statement
return res;
The function returns the total number of operations stored in `res`.
Best Case: O(n), when the array is already strictly increasing.
Average Case: O(n), since we are iterating through the array once.
Worst Case: O(n), when we have to increment each element of the array.
Description: The time complexity is linear because we only traverse the array once.
Best Case: O(1), since no additional space is needed.
Worst Case: O(1), since we only need a constant amount of extra space for variables.
Description: The space complexity is constant because we only use a few variables to store intermediate results.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus