Leetcode 1818: Minimum Absolute Sum Difference
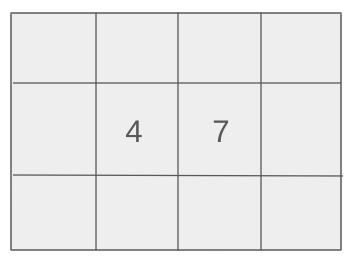
You are given two positive integer arrays nums1 and nums2 of the same length n. You are allowed to change at most one element of nums1 to any other value from nums1 to minimize the total absolute sum difference between nums1 and nums2. Return the minimum absolute sum difference after making the replacement. Since the answer may be large, return it modulo 10^9 + 7.
Problem
Approach
Steps
Complexity
Input: The input consists of two integer arrays nums1 and nums2, both of length n.
Example: nums1 = [3, 7, 6], nums2 = [4, 2, 6]
Constraints:
• 1 <= n <= 10^5
• 1 <= nums1[i], nums2[i] <= 10^5
Output: Return the minimum absolute sum difference after making the replacement, modulo 10^9 + 7.
Example: Output: 2
Constraints:
• The answer should be returned modulo 10^9 + 7.
Goal: Minimize the absolute sum difference by changing one element in nums1.
Steps:
• Calculate the initial absolute sum difference between nums1 and nums2.
• Try replacing one element in nums1 and compute the new sum difference.
• Keep track of the maximum gain (reduction in sum) and compute the result.
Goal: Constraints on the input arrays nums1 and nums2.
Steps:
• n == nums1.length
• n == nums2.length
• 1 <= n <= 10^5
• 1 <= nums1[i], nums2[i] <= 10^5
Assumptions:
• The arrays nums1 and nums2 are non-empty.
• You can only replace one element in nums1.
• Input: nums1 = [3, 7, 6], nums2 = [4, 2, 6]
• Explanation: By replacing one element of nums1 with another from nums1, we minimize the absolute sum difference.
Approach: We aim to minimize the absolute sum difference by trying different replacements in nums1 and comparing the results.
Observations:
• The initial absolute sum difference is computed for the given nums1 and nums2.
• For each element in nums1, we check the possible replacements to minimize the sum.
• Efficiently search for the best replacement by using binary search or sorted data structures.
Steps:
• Calculate the initial absolute sum difference between nums1 and nums2.
• Find the maximum gain that can be obtained by replacing one element in nums1.
• Return the result after applying the best replacement, modulo 10^9 + 7.
Empty Inputs:
• n = 1, nums1 and nums2 are the smallest possible arrays.
Large Inputs:
• The arrays nums1 and nums2 have lengths near the upper limit, n = 10^5.
Special Values:
• All elements in nums1 and nums2 are identical, leading to an absolute sum difference of 0.
Constraints:
• The algorithm should work efficiently for large inputs within the given constraints.
int minAbsoluteSumDiff(vector<int>& nums1, vector<int>& nums2) {
int n = nums1.size();
set<int> sel(begin(nums1), end(nums1));
long gain = 0, res = 0;
for(int i = 0; i < n; i++) {
long og = abs(nums1[i] - nums2[i]);
res += og;
if (gain < og) {
auto num = sel.lower_bound(nums2[i]);
if (num != end(sel)) gain = max(gain, og - abs(*num - nums2[i]));
if (num != begin(sel)) gain = max(gain, og - abs(*prev(num) - nums2[i]));
}
}
return (res - gain) % 1000000007;
}
1 : Function Definition
int minAbsoluteSumDiff(vector<int>& nums1, vector<int>& nums2) {
This is the function definition of `minAbsoluteSumDiff`, which takes two integer vectors `nums1` and `nums2` and returns the minimum possible sum of absolute differences between the two arrays, considering optimal substitutions.
2 : Variable Initialization
int n = nums1.size();
The variable `n` stores the size of the input arrays `nums1` (and `nums2`), assuming both arrays are of the same size.
3 : Set Initialization
set<int> sel(begin(nums1), end(nums1));
A set `sel` is initialized with the elements of `nums1` to allow efficient lookup of elements for calculating the minimum absolute difference.
4 : Variable Initialization
long gain = 0, res = 0;
The variables `gain` and `res` are initialized to 0. `gain` will store the maximum gain that can be achieved by replacing an element, and `res` will accumulate the original sum of absolute differences.
5 : Loop Over Arrays
for(int i = 0; i < n; i++) {
This loop iterates through each index `i` of the arrays `nums1` and `nums2`.
6 : Calculate Original Difference
long og = abs(nums1[i] - nums2[i]);
The variable `og` stores the absolute difference between the elements `nums1[i]` and `nums2[i]` for the current index `i`.
7 : Accumulate Original Sum
res += og;
The absolute difference `og` is added to the `res` variable, which accumulates the total sum of absolute differences between the two arrays.
8 : Gain Calculation
if (gain < og) {
This conditional checks if the current difference `og` is greater than the previously calculated `gain`. If true, it attempts to minimize the difference by checking nearby elements.
9 : Set Lookup
auto num = sel.lower_bound(nums2[i]);
The `lower_bound` function is used to find the smallest element in the set `sel` that is greater than or equal to `nums2[i]`. This helps in finding the best possible replacement to minimize the absolute difference.
10 : Gain Update
if (num != end(sel)) gain = max(gain, og - abs(*num - nums2[i]));
If a valid element `num` is found in the set `sel`, the potential gain is calculated as the difference between the original difference `og` and the new difference after replacing `nums1[i]` with `num`.
11 : Gain Update
if (num != begin(sel)) gain = max(gain, og - abs(*prev(num) - nums2[i]));
This line checks if there is a valid element before `num` in the set `sel` and calculates the potential gain by considering that element as a replacement for `nums1[i]`.
12 : Return Result
return (res - gain) % 1000000007;
The final result is returned as the total sum of absolute differences, reduced by the maximum gain, and taken modulo 1000000007.
Best Case: O(n log n)
Average Case: O(n log n)
Worst Case: O(n log n)
Description: The algorithm requires sorting or binary search, making the time complexity O(n log n).
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) due to the space required for sorting or storing elements.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus