Leetcode 1802: Maximum Value at a Given Index in a Bounded Array
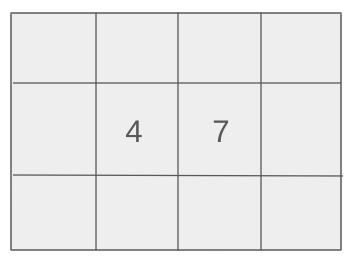
You are tasked with constructing an array
nums
of length n
such that: all elements are positive integers, the difference between adjacent elements is at most 1, the sum of elements does not exceed maxSum
, and the value at index index
is maximized. Return the value at index
in the array nums
.Problem
Approach
Steps
Complexity
Input: The input consists of three integers: `n`, `index`, and `maxSum`. These are the length of the array, the index whose value is to be maximized, and the maximum allowable sum of all elements in the array, respectively.
Example: n = 5, index = 2, maxSum = 10
Constraints:
• 1 <= n <= maxSum <= 10^9
• 0 <= index < n
Output: Return the value at the index `index` in the array `nums` after constructing it under the given conditions.
Example: Output: 3
Constraints:
• The value at `index` must be maximized while ensuring that the sum of the elements in the array does not exceed `maxSum`.
Goal: Construct the array such that the value at `index` is maximized, while adhering to the constraints of adjacent element differences and sum limits.
Steps:
• Use binary search to determine the maximum possible value for `nums[index]`.
• For each candidate value, calculate if the sum of the array can remain within `maxSum` while keeping the difference between adjacent elements at most 1.
Goal: The solution must efficiently handle the large constraints, especially for `n` and `maxSum` as they can be as large as 10^9.
Steps:
• Time complexity should be optimized for large inputs.
• The solution should use binary search to find the optimal value for `nums[index]`.
Assumptions:
• We assume the values are large enough to require an efficient solution using binary search.
• Input: n = 5, index = 2, maxSum = 10
• Explanation: In this case, the array `[1, 2, 3, 2, 1]` satisfies all conditions, and the value at index 2 is maximized as 3, with a total sum of 10.
Approach: The approach uses binary search to determine the maximum possible value for the element at `index`, checking if the sum of the array remains within `maxSum`.
Observations:
• The problem involves finding the maximum possible value at a specific index in an array, while satisfying sum and adjacent element difference constraints.
• Binary search is the most efficient method to maximize the value at the index since it allows us to narrow down the possible values for `nums[index]`.
Steps:
• Use binary search to find the maximum value that `nums[index]` can take, starting from 1 and going up to `maxSum`.
• For each midpoint in the binary search, calculate the sum of the array assuming the value at `index` is fixed at that midpoint, and check if the sum is within `maxSum`.
Empty Inputs:
• There are no empty inputs since `n` is always at least 1.
Large Inputs:
• For large values of `n` and `maxSum`, binary search ensures the solution runs efficiently even when the input values approach their upper bounds.
Special Values:
• Consider the case where `index` is at either end of the array, or when `n` is equal to `maxSum`.
Constraints:
• Ensure the solution efficiently handles the maximum constraints.
bool can(long long pk, long long n, long long i, long long sum) {
sum -= n;
long j = n - i - 1;
long long need = pk * pk - ((pk > i ? (pk - i - 1) * (pk - i) : 0) + (pk > j? (pk - j - 1) * (pk - j): 0)) / 2;
return sum - need >= 0;
}
int maxValue(int n, int index, int mx) {
int ans = 0, l = 0, r = mx;
while(l <= r) {
long mid = l + (r - l + 1) / 2;
// cout << mid << " ";
if(can(mid, n, index, mx)) {
ans = mid;
l = mid + 1;
} else r = mid - 1;
}
return ans + 1;
}
1 : Function Definition
bool can(long long pk, long long n, long long i, long long sum) {
Define the 'can' function which takes parameters pk, n, i, and sum to check if a certain value can be achieved based on the constraints.
2 : Mathematical Operations
sum -= n;
Adjust the sum by subtracting the total number of elements.
3 : Variable Initialization
long j = n - i - 1;
Initialize the variable 'j' to represent the index of the second element in the range.
4 : Mathematical Operations
Blank line for separation.
5 : Mathematical Operations
long long need = pk * pk - ((pk > i ? (pk - i - 1) * (pk - i) : 0) + (pk > j? (pk - j - 1) * (pk - j): 0)) / 2;
Calculate the amount needed based on the value of pk and the indices i and j.
6 : Return
return sum - need >= 0;
Return whether the remaining sum is greater than or equal to the calculated need.
7 : Function Definition
int maxValue(int n, int index, int mx) {
Define the 'maxValue' function which implements a binary search to find the maximum value.
8 : Variable Initialization
int ans = 0, l = 0, r = mx;
Initialize variables for binary search: ans (answer), l (lower bound), and r (upper bound).
9 : Loop and Recursion
while(l <= r) {
Start the binary search loop.
10 : Mathematical Operations
long mid = l + (r - l + 1) / 2;
Calculate the midpoint for the binary search.
11 : Flow Control
if(can(mid, n, index, mx)) {
If the 'can' function returns true for the current mid value, adjust the binary search bounds.
12 : Variable Initialization
ans = mid;
Update the answer with the current value of mid.
13 : Mathematical Operations
l = mid + 1;
Move the lower bound up to search the higher range.
14 : Flow Control
} else r = mid - 1;
Otherwise, move the upper bound down to search the lower range.
15 : Return
return ans + 1;
Return the final answer, incremented by 1 to match the problem's requirements.
Best Case: O(log(maxSum))
Average Case: O(log(maxSum))
Worst Case: O(log(maxSum))
Description: The binary search over the possible values for `nums[index]` results in a time complexity of O(log(maxSum)).
Best Case: O(1)
Worst Case: O(1)
Description: The solution only requires a constant amount of extra space.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus