Leetcode 1800: Maximum Ascending Subarray Sum
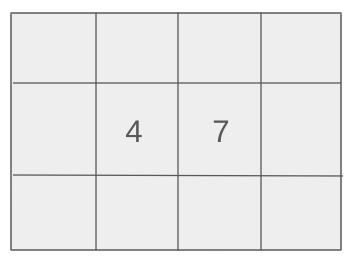
Given an array of positive integers ’nums’, return the maximum possible sum of an ascending subarray. A subarray is ascending if for each i, num[i] < num[i+1].
Problem
Approach
Steps
Complexity
Input: You are given an array 'nums' of positive integers. Your task is to find the maximum sum of a strictly ascending subarray.
Example: nums = [5, 10, 15, 2, 8, 30]
Constraints:
• 1 <= nums.length <= 100
• 1 <= nums[i] <= 100
Output: Return the maximum sum of any strictly ascending subarray.
Example: For nums = [5, 10, 15, 2, 8, 30], the output will be 55.
Constraints:
Goal: The goal is to find the sum of the maximum ascending subarray in 'nums'.
Steps:
• 1. Iterate through the array 'nums'.
• 2. Track the sum of the current ascending subarray. Reset the sum whenever the sequence stops being strictly ascending.
• 3. Keep a variable to track the maximum sum found.
Goal: The problem has constraints regarding the array size and values.
Steps:
• 1 <= nums.length <= 100
• 1 <= nums[i] <= 100
Assumptions:
• The array will contain at least one number.
• Input: nums = [5, 10, 15, 2, 8, 30]
• Explanation: In this example, the ascending subarrays are [5, 10, 15] and [2, 8, 30]. The maximum sum is 5 + 10 + 15 + 30 = 55.
Approach: We will iterate through the array and calculate the sum of all strictly ascending subarrays while updating the maximum sum whenever needed.
Observations:
• An ascending subarray is defined by consecutive elements where each element is greater than the previous one.
• We need to check each subarray's sum and compare it with the maximum sum so far.
Steps:
• 1. Initialize 'max_sum' and 'current_sum' to the first element of the array.
• 2. Loop through the array starting from the second element.
• 3. If the current element is greater than the previous one, add it to the 'current_sum'. Otherwise, reset 'current_sum' to the current element.
• 4. After each addition, update 'max_sum' with the maximum value between 'max_sum' and 'current_sum'.
• 5. Return 'max_sum'.
Empty Inputs:
• If the array is empty, return 0.
Large Inputs:
• Ensure that the solution can handle arrays with up to 100 elements.
Special Values:
• For arrays with only one element, the maximum sum is the value of that element.
Constraints:
• Make sure the solution runs efficiently within the given constraints.
int maxAscendingSum(vector<int>& nums) {
int sum = nums[0];
int maxi = sum;
if(nums.size() == 1) return nums[0];
for(int i = 1; i < nums.size(); i++){
if(nums[i] <= nums[i-1]){
sum = 0;
}
sum += nums[i];
maxi = max(sum,maxi);
}
return maxi;
}
1 : Function Definition
int maxAscendingSum(vector<int>& nums) {
This is the function definition. The input is a vector of integers, `nums`, and the output is an integer representing the maximum sum of an ascending subarray.
2 : Variable Initialization
int sum = nums[0];
Initialize `sum` to the first element of the `nums` array. This variable tracks the sum of the current ascending subarray.
3 : Variable Initialization
int maxi = sum;
Initialize `maxi` to the value of `sum`. This will track the maximum sum encountered during the iteration.
4 : Edge Case Handling
if(nums.size() == 1) return nums[0];
Handle the edge case where the input list has only one element. In this case, the only possible sum is the element itself.
5 : Loop Start
for(int i = 1; i < nums.size(); i++){
Start a loop from the second element to the last element of the `nums` array to evaluate each element in relation to its predecessor.
6 : Condition Check
if(nums[i] <= nums[i-1]){
Check if the current element is less than or equal to the previous element. If so, the ascending subarray has ended.
7 : Reset Sum
sum = 0;
Reset `sum` to 0 since the current subarray is no longer strictly increasing.
8 : Sum Update
sum += nums[i];
Add the current element to `sum` as part of the current ascending subarray.
9 : Maximum Update
maxi = max(sum,maxi);
Update `maxi` with the larger of the current sum (`sum`) or the previous maximum (`maxi`).
10 : Return Statement
return maxi;
Return the maximum sum of the strictly increasing subarray found during the iteration.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n) where n is the length of the array, as we only iterate through the array once.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is O(1) as we only need a few variables to track the current sum and the maximum sum.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus