Leetcode 1786: Number of Restricted Paths From First to Last Node
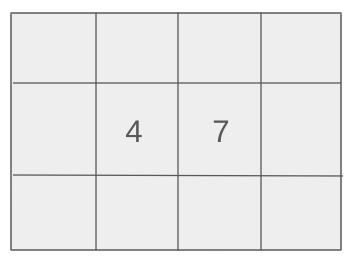
You are given a connected, undirected, weighted graph with n nodes, labeled from 1 to n. An array ’edges’ represents the edges in the graph where each element edges[i] = [ui, vi, weighti] indicates that there is an edge between nodes ui and vi with a weight of weighti. A path from node start to node end is a sequence of nodes [z0, z1, …, zk] such that z0 = start, zk = end, and there is an edge between zi and zi+1 for each 0 <= i <= k-1.
The distance of a path is the sum of the weights of the edges along the path. A restricted path is a path where the distance from node ’n’ to node zi is strictly greater than the distance from node ’n’ to node zi+1 for each 0 <= i <= k-1.
You need to return the number of restricted paths from node 1 to node n, modulo 10^9 + 7.
int countRestrictedPaths(int n, vector<vector<int>>& es) {
vector<vector<pii>> gph(n + 1);
for(int i = 0; i < es.size(); i++) {
int u = es[i][0], v = es[i][1], d = es[i][2];
gph[u].push_back({d, v});
gph[v].push_back({d, u});
}
vector<int> dst(n + 1, INT_MAX);
priority_queue<pii, vector<pii>, greater<>> pq;
pq.push({0, n});
dst[n] = 0;
while(!pq.empty()) {
auto [d, u] = pq.top();
pq.pop();
for(auto & x: gph[u]) {
int nxt = x.second, t = x.first;
// if(t != dst[nxt]) continue;
if(dst[nxt] > t + d) {
dst[nxt] = t + d;
pq.push({t + d, nxt});
}
}
}
vector<int> dp(n + 1, -1);
return dfs(gph, dst, n, dp);
}
int dfs(vector<vector<pii>> &gph, vector<int> &dst, int s, vector<int> &dp) {
if (s == 1) return 1;
if (dp[s] != -1) return dp[s];
int mod = 1e9 + 7;
int sum = 0, w = 0, val = 0;
for(auto &v : gph[s]) {
w = dst[s];
val = dst[v.second];
if (val > w) {
sum = (sum % mod+ dfs(gph, dst, v.second, dp) % mod) % mod;
}
}
return dp[s] = sum % mod;
}
int countRestrictedPaths(int n, vector<vector<int>>& es) {
vector<vector<pii>> gph(n + 1);
for(int i = 0; i < es.size(); i++) {
int u = es[i][0], v = es[i][1], d = es[i][2];
gph[u].push_back({d, v});
gph[v].push_back({d, u});
vector<int> dst(n + 1, INT_MAX);
priority_queue<pii, vector<pii>, greater<>> pq;
pq.push({0, n});
dst[n] = 0;
while(!pq.empty()) {
auto [d, u] = pq.top();
pq.pop();
for(auto & x: gph[u]) {
int nxt = x.second, t = x.first;
if(dst[nxt] > t + d) {
dst[nxt] = t + d;
pq.push({t + d, nxt});
vector<int> dp(n + 1, -1);
return dfs(gph, dst, n, dp);
int dfs(vector<vector<pii>> &gph, vector<int> &dst, int s, vector<int> &dp) {
if (s == 1) return 1;
if (dp[s] != -1) return dp[s];
int mod = 1e9 + 7;
int sum = 0, w = 0, val = 0;
for(auto &v : gph[s]) {
w = dst[s];
val = dst[v.second];
if (val > w) {
sum = (sum % mod+ dfs(gph, dst, v.second, dp) % mod) % mod;
return dp[s] = sum % mod;
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|