Leetcode 1775: Equal Sum Arrays With Minimum Number of Operations
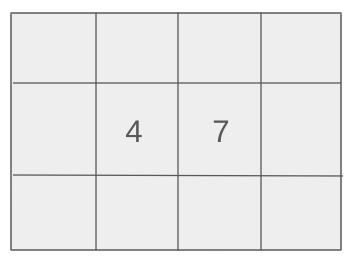
You are given two arrays, nums1 and nums2, with integers between 1 and 6. The lengths of the two arrays can differ. In one operation, you can change any element in either array to any value between 1 and 6. The task is to find the minimum number of operations required to make the sums of the two arrays equal. If it is not possible to make the sums equal, return -1.
Problem
Approach
Steps
Complexity
Input: You are given two arrays nums1 and nums2 of integers between 1 and 6. You can perform any number of operations where you change an element's value in either array.
Example: nums1 = [2, 3, 4, 5, 6], nums2 = [1, 2, 2, 3]
Constraints:
• 1 <= nums1.length, nums2.length <= 10^5
• 1 <= nums1[i], nums2[i] <= 6
Output: Return the minimum number of operations required to make the sums of nums1 and nums2 equal, or return -1 if it is not possible.
Example: Input: nums1 = [2, 3, 4, 5, 6], nums2 = [1, 2, 2, 3], Output: 2
Constraints:
Goal: To equalize the sum of nums1 and nums2 with the minimum number of operations by changing elements within the allowed range (1 to 6).
Steps:
• 1. Calculate the sum of both nums1 and nums2.
• 2. If the sums are already equal, return 0.
• 3. If it is not possible to equalize the sums by the allowed changes, return -1.
• 4. Otherwise, iterate through the arrays and apply changes to the elements of nums1 or nums2 to balance the sums with the fewest changes.
Goal: The arrays nums1 and nums2 can have up to 10^5 elements, and the values within both arrays range from 1 to 6.
Steps:
• 1 <= nums1.length, nums2.length <= 10^5
• 1 <= nums1[i], nums2[i] <= 6
Assumptions:
• You can modify any element in either array within the allowed range (1 to 6).
• You are allowed to increase or decrease elements to any value between 1 and 6.
• Input: Input: nums1 = [2, 3, 4, 5, 6], nums2 = [1, 2, 2, 3]
• Explanation: By changing nums2[0] to 6 and nums2[1] to 6, we make the sums of nums1 and nums2 equal (both sums are 20), so the answer is 2 operations.
• Input: Input: nums1 = [1, 1, 1, 1], nums2 = [6, 6]
• Explanation: It is not possible to make the sums of nums1 and nums2 equal because nums1's sum cannot be reduced and nums2's sum cannot be increased sufficiently.
Approach: The approach is to iteratively compare the sums of nums1 and nums2 and apply changes to the elements that will bring the sums closer to each other, while tracking the number of changes made.
Observations:
• If one array's sum is greater than the other, we need to decrease the larger sum or increase the smaller sum.
• If the difference between the sums is too large to be adjusted with valid operations, return -1.
• We need to carefully balance the sums by considering the potential effect of changing each element by up to 5 (from 1 to 6).
Steps:
• 1. Calculate the sums of nums1 and nums2.
• 2. Sort both arrays to maximize the effect of changing the largest elements.
• 3. Iteratively apply changes to the largest elements of nums1 or nums2 to reduce the difference in their sums.
• 4. Track the number of operations and return the result once the sums are equal.
Empty Inputs:
• Ensure that neither array is empty.
Large Inputs:
• The solution should handle large arrays efficiently, up to the limit of 10^5 elements.
Special Values:
• Consider cases where the difference between the sums is too large to be fixed, such as when one sum is much larger than the other.
Constraints:
• Respect the constraint that the elements of nums1 and nums2 must be between 1 and 6.
int minOperations(vector<int>& nums1, vector<int>& nums2) {
if( nums1.size() > 6*nums2.size() ||
nums2.size() > 6*nums1.size())
return -1;
int sum1 = accumulate(nums1.begin(), nums1.end(), 0);
int sum2 = accumulate(nums2.begin(), nums2.end(), 0);
if(sum1 > sum2) return minOperations(nums2, nums1);
sort(nums1.begin(), nums1.end());
sort(nums2.begin(), nums2.end());
int i = 0, j = nums2.size() - 1, ops = 0;
while(sum2 > sum1) {
if(j < 0 || i < nums1.size() && 6 - nums1[i] > nums2[j] - 1) {
sum1 += 6 - nums1[i++];
} else {
sum2 -= nums2[j--] - 1;
}
++ops;
}
return ops;
}
1 : Function Definition
int minOperations(vector<int>& nums1, vector<int>& nums2) {
Define the function `minOperations` that takes two integer vectors `nums1` and `nums2` and returns the minimum number of operations required to balance their sums.
2 : Condition Check
if( nums1.size() > 6*nums2.size() ||
Check if the size of `nums1` is more than six times the size of `nums2`, or if `nums2` is more than six times the size of `nums1`. If so, return -1 as no solution is possible.
3 : Condition Check
nums2.size() > 6*nums1.size())
Continuing the condition check to ensure the size constraints are met. If the sizes don't meet the criteria, return -1.
4 : Return Statement
return -1;
If the size condition is not met, return `-1`, indicating it's not possible to balance the sums.
5 : Sum Calculation
int sum1 = accumulate(nums1.begin(), nums1.end(), 0);
Calculate the sum of all elements in `nums1` using the `accumulate` function.
6 : Sum Calculation
int sum2 = accumulate(nums2.begin(), nums2.end(), 0);
Calculate the sum of all elements in `nums2` using the `accumulate` function.
7 : Recursive Call
if(sum1 > sum2) return minOperations(nums2, nums1);
If the sum of `nums1` is greater than `nums2`, call the function recursively with the lists swapped to ensure `nums1` is the smaller list.
8 : Sorting
sort(nums1.begin(), nums1.end());
Sort `nums1` in ascending order to facilitate the smallest possible operations.
9 : Sorting
sort(nums2.begin(), nums2.end());
Sort `nums2` in ascending order to facilitate the largest possible operations.
10 : Variable Initialization
int i = 0, j = nums2.size() - 1, ops = 0;
Initialize indices `i` for `nums1`, `j` for `nums2`, and a variable `ops` to keep track of the number of operations.
11 : While Loop
while(sum2 > sum1) {
Start a `while` loop that runs until `sum1` is greater than or equal to `sum2`.
12 : Condition Check
if(j < 0 || i < nums1.size() && 6 - nums1[i] > nums2[j] - 1) {
Check if `j` is out of bounds or if the next operation on `nums1[i]` would be more beneficial than decrementing `nums2[j]`.
13 : Update Sum1
sum1 += 6 - nums1[i++];
If the operation on `nums1[i]` is more beneficial, increment `sum1` by the difference between 6 and the current value of `nums1[i]`, then increment `i`.
14 : Else Condition
} else {
If the condition above is false, proceed to decrement `nums2[j]` instead.
15 : Update Sum2
sum2 -= nums2[j--] - 1;
Decrement `sum2` by `nums2[j] - 1`, then decrement `j`.
16 : Operation Count
++ops;
Increment the `ops` variable to count the operation performed.
17 : Return Statement
return ops;
Return the total number of operations required.
Best Case: O(n log n)
Average Case: O(n log n)
Worst Case: O(n log n)
Description: The dominant operation is sorting the arrays.
Best Case: O(n)
Worst Case: O(n)
Description: Space complexity is O(n) due to the space required for sorting.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus