Leetcode 1764: Form Array by Concatenating Subarrays of Another Array
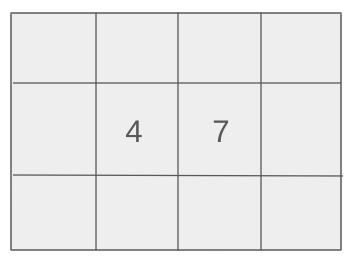
Given an array of subarrays
groups
and a single array nums
, your task is to determine whether it is possible to extract each subarray from groups
as a contiguous segment from nums
. The subarrays must appear in the same order as in groups
, and they should be disjoint, meaning no element from nums
can belong to more than one subarray. Return true if this is possible, and false otherwise.Problem
Approach
Steps
Complexity
Input: You are given a 2D array `groups` where each subarray is a sequence of integers. Additionally, you are given an integer array `nums`.
Example: Input: groups = [[2, 3], [1, 2, 3]], nums = [2, 3, 1, 2, 3, 4]
Constraints:
• 1 <= groups.length <= 1000
• 1 <= groups[i].length <= 1000
• 1 <= sum(groups[i].length) <= 1000
• 1 <= nums.length <= 1000
• nums and group elements are between -10^7 and 10^7
Output: Return true if you can find each subarray from `groups` as disjoint subarrays within `nums` while maintaining the order, otherwise return false.
Example: Output: true
Constraints:
Goal: The goal is to check whether each subarray from `groups` can be found in `nums` in the given order and that the subarrays are disjoint.
Steps:
• 1. Start iterating through `nums` while keeping track of the current subarray being matched from `groups`.
• 2. For each subarray, check if it appears in `nums` as a contiguous block.
• 3. Once a match is found, ensure no elements from `nums` are reused for the next subarray by continuing the search beyond the matched segment.
• 4. Return true if all subarrays are found, otherwise return false.
Goal: The solution must handle edge cases, such as when there are no possible matches or the input sizes are near their limits.
Steps:
• The solution must handle cases where `nums` or the subarrays in `groups` are large efficiently.
Assumptions:
• The arrays `nums` and `groups` consist of integers only.
• Subarrays in `groups` must appear in the same order in `nums`.
• Input: Input: groups = [[2, 3], [1, 2, 3]], nums = [2, 3, 1, 2, 3, 4]
• Explanation: The first subarray [2, 3] can be found at the start of `nums`. The second subarray [1, 2, 3] follows immediately after the first. Thus, the answer is true.
• Input: Input: groups = [[10, -2], [1, 2, 3, 4]], nums = [1, 2, 3, 4, 10, -2]
• Explanation: The subarrays are not in the same order in `nums`. The second subarray [1, 2, 3, 4] appears before [10, -2], so the answer is false.
• Input: Input: groups = [[3, 4], [5, 6]], nums = [3, 5, 4, 6]
• Explanation: The subarrays are disjoint, but they do not appear in the same order. Therefore, the answer is false.
Approach: The solution will iterate through `nums` and try to match each subarray from `groups` in sequence. We will attempt to find each subarray as a contiguous block, ensuring that no element is shared between subarrays.
Observations:
• The subarrays must be found in order, and no overlap should occur between the subarrays in `nums`.
• An efficient approach is to use a greedy matching strategy to find each subarray and continue searching from the end of the last match.
Steps:
• 1. Start iterating through `nums` with an index to check for the first subarray.
• 2. For each subarray in `groups`, find its exact match in `nums` while ensuring no overlap with previous matches.
• 3. Once a subarray is found, move the index in `nums` past that subarray and continue checking for the next subarray in `groups`.
• 4. Return true if all subarrays are found, otherwise return false.
Empty Inputs:
• If `nums` is empty or if the total sum of lengths of subarrays in `groups` exceeds the length of `nums`, return false.
Large Inputs:
• The solution must handle cases where the length of `nums` and the total number of elements in `groups` are close to their upper limits efficiently.
Special Values:
• If any group is empty or a group doesn't have a matching subsequence in `nums`, return false.
Constraints:
• The solution should be optimized to handle up to 1000 subarrays in `groups` and 1000 elements in `nums`.
bool canChoose(vector<vector<int>>& group, vector<int>& nums) {
int numsIdx = 0, grpIdx = 0;
while(numsIdx < nums.size() && grpIdx < group.size()) {
int matchCnt = 0;
while(numsIdx + matchCnt < nums.size() &&
matchCnt < group[grpIdx].size() &&
nums[numsIdx + matchCnt] == group[grpIdx][matchCnt])
matchCnt++;
if(matchCnt == group[grpIdx].size()) {
grpIdx++;
numsIdx += matchCnt;
} else numsIdx++;
}
return grpIdx == group.size();
}
1 : Function Declaration
bool canChoose(vector<vector<int>>& group, vector<int>& nums) {
Declares the function for determining if groups can be matched in the given array `nums`.
2 : Variable Initialization
int numsIdx = 0, grpIdx = 0;
Initializes indices for tracking the current position in `nums` and `group`.
3 : While Loop
while(numsIdx < nums.size() && grpIdx < group.size()) {
Iterates over `nums` and `group` as long as indices are within bounds.
4 : Variable Initialization
int matchCnt = 0;
Initializes a counter to track the number of matching elements for the current group.
5 : While Loop
while(numsIdx + matchCnt < nums.size() &&
Checks for matching elements between the current group and the array `nums`.
6 : Condition Check
matchCnt < group[grpIdx].size() &&
Ensures the match count does not exceed the size of the current group.
7 : Condition Check
nums[numsIdx + matchCnt] == group[grpIdx][matchCnt])
Checks if the current elements in `nums` and `group` match.
8 : Increment
matchCnt++;
Increments the match count when elements match.
9 : Block End
Empty line for separating blocks for readability.
10 : Condition Check
if(matchCnt == group[grpIdx].size()) {
Checks if all elements in the current group have been matched.
11 : Index Update
grpIdx++;
Moves to the next group after successfully matching the current group.
12 : Index Update
numsIdx += matchCnt;
Skips past the matched elements in `nums`.
13 : Else Block
} else numsIdx++;
Moves to the next element in `nums` if the current group does not match.
14 : Return Statement
return grpIdx == group.size();
Returns `true` if all groups were matched successfully; otherwise, `false`.
Best Case: O(n)
Average Case: O(n^2)
Worst Case: O(n^3)
Description: In the worst case, each subarray needs to be matched against a portion of `nums`, leading to a time complexity of O(n^3) when considering the sum of subarray lengths.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is constant since we are only using a few integer variables to track indices and matches.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus