Leetcode 1758: Minimum Changes To Make Alternating Binary String
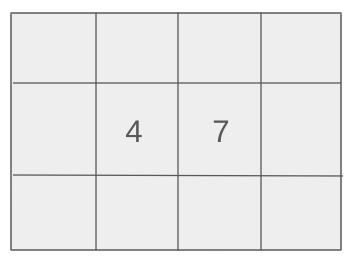
You are given a string
s
consisting only of the characters ‘0’ and ‘1’. The string is considered alternating if no two adjacent characters are the same. Your task is to determine the minimum number of operations needed to make the string alternating, where in one operation, you can change any ‘0’ to ‘1’ or vice versa.Problem
Approach
Steps
Complexity
Input: You are given a string `s` which only contains the characters '0' and '1'.
Example: Input: s = '1001'
Constraints:
• 1 <= s.length <= 10^4
• s[i] is either '0' or '1'.
Output: Return the minimum number of operations needed to make `s` alternating.
Example: Output: 1
Constraints:
• The number of operations should be minimized.
Goal: The goal is to minimize the number of changes required to convert the given string into an alternating string.
Steps:
• 1. Traverse the string `s` and check each adjacent pair of characters.
• 2. If two adjacent characters are the same, a change is needed.
• 3. Calculate the number of changes required for both possible alternating patterns: '010101...' and '101010...'.
• 4. Return the minimum of these two values.
Goal: Make sure the solution works for large inputs and performs within time limits.
Steps:
• The solution should handle strings of length up to 10^4 efficiently.
Assumptions:
• The string `s` is not empty and contains only '0' or '1'.
• Input: Input: s = '1001'
• Explanation: To make the string alternating, change the second '0' to '1' or the last '1' to '0'. The minimum number of operations needed is 1.
• Input: Input: s = '1101'
• Explanation: Here, the first two '1's are adjacent and need to be altered to make the string alternating. Two changes are required.
Approach: The approach is to calculate the number of operations required for both alternating patterns: '010101...' and '101010...'. For each pattern, count how many characters need to be changed and then choose the minimum number of changes.
Observations:
• The problem is about comparing the string to two alternating patterns and finding the minimum number of changes needed.
• Since there are only two possible alternating patterns, we can compare the string to each pattern and count the differences.
Steps:
• 1. Initialize two counters, one for each pattern ('010101...' and '101010...').
• 2. Traverse the string and compare each character with the corresponding character in both alternating patterns.
• 3. Count the differences for both patterns.
• 4. Return the minimum count as the result.
Empty Inputs:
• If the string is empty, return 0 (no changes needed).
Large Inputs:
• Ensure the solution can handle strings of length up to 10^4 efficiently.
Special Values:
• If the string is already alternating, the result is 0.
Constraints:
• The solution should be optimized to handle the maximum string length.
int minOperations(string s) {
int zero = 0, one = 0, zf = false, of = false;
for(int i = 0; i < s.size(); i++) {
if(zf && s[i] == '0') zero++;
if(!zf && s[i] == '1') zero++;
if(!of && s[i] == '0') one++;
if(of && s[i] == '1') one++;
zf = !zf, of = !of;
}
return min(zero, one);
}
1 : Function Declaration
int minOperations(string s) {
Declares the function to calculate the minimum operations for an alternating binary string.
2 : Variable Initialization
int zero = 0, one = 0, zf = false, of = false;
Initializes counters and flags for tracking mismatches with alternating patterns.
3 : Loop
for(int i = 0; i < s.size(); i++) {
Iterates over the characters of the input string to count mismatches.
4 : Condition Check
if(zf && s[i] == '0') zero++;
Checks if the current character matches the '0' expectation for the first pattern.
5 : Condition Check
if(!zf && s[i] == '1') zero++;
Checks if the current character matches the '1' expectation for the first pattern.
6 : Condition Check
if(!of && s[i] == '0') one++;
Checks if the current character matches the '0' expectation for the second pattern.
7 : Condition Check
if(of && s[i] == '1') one++;
Checks if the current character matches the '1' expectation for the second pattern.
8 : Flag Update
zf = !zf, of = !of;
Flips the flags to alternate the expected pattern for the next iteration.
9 : Return Statement
return min(zero, one);
Returns the minimum of the two mismatch counts as the result.
Best Case: O(n), where n is the length of the string.
Average Case: O(n), as each character is checked against both alternating patterns.
Worst Case: O(n), where n is the length of the string.
Description: The time complexity is linear, as the string is traversed once.
Best Case: O(1), as no additional space is needed regardless of the input.
Worst Case: O(1), since only a few variables are used for comparison.
Description: The space complexity is constant, as only a few counters are used.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus