Leetcode 1738: Find Kth Largest XOR Coordinate Value
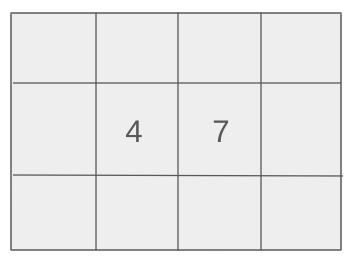
You are given a 2D matrix of size
m x n
, consisting of non-negative integers. You are also given an integer k
. The value of coordinate (a, b)
in the matrix is the XOR of all the values from matrix[0][0]
to matrix[a][b]
(inclusive), where 0 <= a < m
and 0 <= b < n
(0-indexed). Your task is to find the k
th largest value (1-indexed) among all the XOR values of matrix coordinates.Problem
Approach
Steps
Complexity
Input: You are given a 2D matrix and an integer `k`.
Example: Input: matrix = [[1, 3], [5, 7]], k = 2
Constraints:
• 1 <= m, n <= 1000
• 0 <= matrix[i][j] <= 10^6
• 1 <= k <= m * n
Output: Return the `k`th largest value among all the XOR values of the coordinates in the matrix.
Example: Output: 7
Constraints:
• The value of `k` will always be valid for the given matrix.
Goal: To calculate the XOR of all coordinates in the matrix, store these values, and then return the `k`th largest value.
Steps:
• 1. For each row, calculate the XOR of all the elements up to that point (left to right).
• 2. For each column, calculate the XOR of all the elements up to that point (top to bottom).
• 3. Store all the XOR values in a list.
• 4. Use a priority queue to find the `k`th largest value in the list.
Goal: The constraints for this problem are as follows:
Steps:
• Matrix dimensions `m` and `n` can be up to 1000.
• Matrix elements are non-negative integers and can go up to 10^6.
• The value of `k` is guaranteed to be within the valid range for the given matrix.
Assumptions:
• The input matrix will always have at least one row and column.
• Input: Input: matrix = [[1, 3], [5, 7]], k = 2
• Explanation: The XOR values of all coordinates are: [1, 2, 7, 5]. The 2nd largest value is 7.
• Input: Input: matrix = [[1, 2, 3], [4, 5, 6]], k = 3
• Explanation: The XOR values of all coordinates are: [1, 3, 0, 4, 1, 7]. The 3rd largest value is 4.
Approach: The solution involves calculating the XOR of each matrix coordinate, then sorting these values to find the `k`th largest value.
Observations:
• The XOR operation combines elements in a way that is sensitive to the order of operations, so we need to compute the values step by step.
• The XOR values are dependent on both the row and column, and we must consider both dimensions while calculating.
Steps:
• 1. Precompute the XOR values for each coordinate by iterating over each row and column.
• 2. Store the XOR values in a list.
• 3. Use a priority queue to efficiently retrieve the `k`th largest value.
• 4. Return the `k`th largest value.
Empty Inputs:
• The matrix will always have at least one row and one column.
Large Inputs:
• Ensure the solution handles the largest possible matrix sizes efficiently.
Special Values:
• Consider cases where all matrix values are the same or the XOR results in repeating values.
Constraints:
• The algorithm must handle matrices with dimensions up to 1000 x 1000.
int kthLargestValue(vector<vector<int>>& mtx, int k) {
int m = mtx.size(), n = mtx[0].size();
for(int i = 0; i < m; i++) {
for(int j = 1; j < n; j++) {
mtx[i][j] = mtx[i][j-1]^mtx[i][j];}
}
for(int i = 0; i < n; i++) {
for(int j = 1; j < m; j++) {
mtx[j][i] = mtx[j -1][i]^mtx[j][i]; }
}
priority_queue<int, vector<int>, greater<int>> pq;
for(int i = 0; i < m; i++) {
for(int j= 0; j < n; j++) {
if(pq.size() < k) {
pq.push(mtx[i][j]); }
else {
if(pq.top() < mtx[i][j]) {
pq.pop();
pq.push(mtx[i][j]);
}
}
}
}
return pq.top();
}
1 : Function Declaration
int kthLargestValue(vector<vector<int>>& mtx, int k) {
This is the function declaration for `kthLargestValue`, which takes a matrix `mtx` and an integer `k` as input, returning the kth largest value from the matrix.
2 : Variable Initialization
int m = mtx.size(), n = mtx[0].size();
Here, the dimensions of the matrix `mtx` are stored in variables `m` (number of rows) and `n` (number of columns).
3 : Loop Start
for(int i = 0; i < m; i++) {
This loop starts iterating through each row of the matrix.
4 : Loop Start
for(int j = 1; j < n; j++) {
This nested loop iterates through each column of the current row, starting from the second column.
5 : XOR Operation
mtx[i][j] = mtx[i][j-1]^mtx[i][j];}
This line performs an XOR operation on the current element and the previous element in the row, updating the matrix with the result. It builds a running XOR prefix sum along each row.
6 : Loop Start
for(int i = 0; i < n; i++) {
This loop starts iterating through each column of the matrix.
7 : Loop Start
for(int j = 1; j < m; j++) {
This nested loop iterates through each row of the current column, starting from the second row.
8 : XOR Operation
mtx[j][i] = mtx[j -1][i]^mtx[j][i]; }
This line performs an XOR operation on the current element and the previous element in the column, updating the matrix with the result. It builds a running XOR prefix sum along each column.
9 : Priority Queue Declaration
priority_queue<int, vector<int>, greater<int>> pq;
This line declares a priority queue (min-heap) `pq` that will store integers and always keep the smallest value at the top.
10 : Loop Start
for(int i = 0; i < m; i++) {
This loop starts iterating through each row of the matrix to process the elements.
11 : Loop Start
for(int j= 0; j < n; j++) {
This nested loop iterates through each column in the current row.
12 : Condition Check
if(pq.size() < k) {
This condition checks if the priority queue contains fewer than `k` elements.
13 : Queue Operation
pq.push(mtx[i][j]); }
If the queue has fewer than `k` elements, the current matrix element is pushed into the priority queue.
14 : Else Block
else {
If the queue already contains `k` elements, the program enters the `else` block to potentially replace the smallest element.
15 : Condition Check
if(pq.top() < mtx[i][j]) {
This condition checks if the smallest element in the queue is smaller than the current matrix element.
16 : Queue Operation
pq.pop();
If the smallest element in the queue is smaller than the current element, it is removed from the queue.
17 : Queue Operation
pq.push(mtx[i][j]);
The current matrix element is pushed into the queue.
18 : Return
return pq.top();
This returns the top element of the priority queue, which is the kth largest element in the matrix.
Best Case: O(m * n), where `m` and `n` are the dimensions of the matrix.
Average Case: O(m * n log(m * n)), due to sorting or using a priority queue.
Worst Case: O(m * n log(m * n)), as we need to compute XOR for every coordinate and then find the `k`th largest value.
Description: The time complexity is dominated by the computation of XOR values and the retrieval of the `k`th largest value.
Best Case: O(m * n), as we need to store all the XOR values.
Worst Case: O(m * n), since we store the XOR values of all coordinates.
Description: The space complexity is proportional to the number of coordinates in the matrix.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus