Leetcode 1737: Change Minimum Characters to Satisfy One of Three Conditions
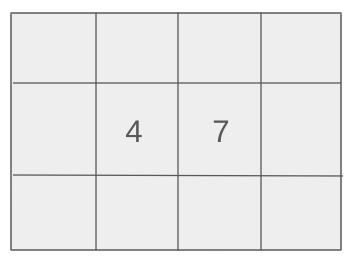
You are given two strings, a
and b
, consisting of lowercase letters. In one operation, you can change any character in either string to any lowercase letter. Your goal is to perform the minimum number of operations to satisfy one of the following three conditions:
- Every character in string
a
is strictly less than every character in stringb
alphabetically. - Every character in string
b
is strictly less than every character in stringa
alphabetically. - Both
a
andb
consist of only one distinct character.
Return the minimum number of operations needed to achieve one of these conditions.
Problem
Approach
Steps
Complexity
Input: You are given two strings `a` and `b` that consist of lowercase letters.
Example: Input: a = "cbd", b = "bbf"
Constraints:
• 1 <= a.length, b.length <= 10^5
• Strings `a` and `b` consist only of lowercase letters.
Output: Return the minimum number of operations required to satisfy one of the three conditions mentioned above.
Example: Output: 3
Constraints:
• The number of operations should be minimized while achieving one of the valid conditions.
Goal: The goal is to determine the minimum number of operations needed to achieve one of the valid conditions by analyzing the frequency of characters in both strings.
Steps:
• 1. Calculate the frequency of characters in both strings `a` and `b`.
• 2. Check each of the three conditions and calculate the number of operations needed for each.
• 3. Return the minimum number of operations required.
Goal: The following constraints hold for the problem:
Steps:
• Strings `a` and `b` have a length of at least 1 and at most 10^5.
• Both strings consist only of lowercase letters.
Assumptions:
• The input strings will always be valid, containing only lowercase letters.
• Input: Input: a = "aba", b = "caa"
• Explanation: We can achieve a valid condition in 2 operations: Change `b` to `ccc` (operation 1), making all characters in `a` less than those in `b`.
• Input: Input: a = "dabadd", b = "cda"
• Explanation: The best solution is to change all characters in `b` to 'e' (operation 3), making every character in `a` less than those in `b`.
Approach: We can solve this problem by analyzing the frequency of each character in both strings `a` and `b`. Then, we calculate the number of operations required for each condition and return the minimum number.
Observations:
• We need to compare the characters in both strings to find the best way to satisfy one of the conditions.
• Instead of modifying characters one by one, we can analyze the frequency distribution to determine the minimum number of operations needed.
Steps:
• 1. Count the frequency of each letter in both strings `a` and `b`.
• 2. Check how many operations it takes to make all characters in `a` strictly less than those in `b` or vice versa.
• 3. Calculate the number of operations needed to make both strings consist of one distinct letter.
• 4. Return the minimum number of operations from the three conditions.
Empty Inputs:
• The input strings will always have at least one character.
Large Inputs:
• For large inputs (e.g., 10^5 characters), ensure that the solution handles the character counting efficiently.
Special Values:
• Consider edge cases where the strings already satisfy one of the conditions.
Constraints:
• The solution must work within the constraints where `a` and `b` can have lengths up to 10^5.
class Solution {
typedef long long ll;
public:
int minCharacters(string a, string b) {
int m = a.size(), n = b.size();
vector<int> ca(26, 0), cb(26, 0);
for(int i = 0; i < m; i++) {
ca[a[i] - 'a']++; }
for(int j = 0; j < n; j++) {
cb[b[j] - 'a']++; }
int res = m + n;
for(int i = 0; i < 26; i++) {
res = min(res, m + n - ca[i] - cb[i]);
if(i > 0) {
ca[i] += ca[i - 1];
cb[i] += cb[i - 1];
}
if(i < 25) {
res = min(res, m - ca[i] + cb[i]);
res = min(res, n - cb[i] + ca[i]);
}
}
return res;
}
1 : Class Declaration
class Solution {
This line defines the class `Solution`, which contains the main logic for solving the problem.
2 : Type Alias
typedef long long ll;
Here, a type alias `ll` is defined for `long long` to simplify the code and make it more readable.
3 : Access Modifier
public:
This access modifier makes the following methods accessible outside the class.
4 : Function Declaration
int minCharacters(string a, string b) {
The function `minCharacters` is declared, taking two string inputs `a` and `b`. It returns the minimum number of changes required to make the strings distinct.
5 : Variable Initialization
int m = a.size(), n = b.size();
Here, the sizes of the input strings `a` and `b` are stored in variables `m` and `n` respectively.
6 : Array Initialization
vector<int> ca(26, 0), cb(26, 0);
Two vectors `ca` and `cb` are initialized to store the frequency of each character (from 'a' to 'z') in the strings `a` and `b`.
7 : Loop Start
for(int i = 0; i < m; i++) {
This loop iterates through the characters in string `a`.
8 : Character Counting
ca[a[i] - 'a']++; }
For each character in `a`, the frequency count in vector `ca` is incremented based on the character's position in the alphabet.
9 : Loop Start
for(int j = 0; j < n; j++) {
This loop iterates through the characters in string `b`.
10 : Character Counting
cb[b[j] - 'a']++; }
For each character in `b`, the frequency count in vector `cb` is incremented based on the character's position in the alphabet.
11 : Blank
A blank line used for clarity between code sections.
12 : Initial Result Calculation
int res = m + n;
The variable `res` is initialized to the sum of the lengths of `a` and `b`, representing the worst case where all characters need to be modified.
13 : Loop Start
for(int i = 0; i < 26; i++) {
This loop iterates through each character (from 'a' to 'z') to calculate the minimum number of changes.
14 : Frequency Comparison
res = min(res, m + n - ca[i] - cb[i]);
For each character, this line updates `res` to the minimum number of changes by comparing the sum of `m + n` and the frequency counts in `ca` and `cb`.
15 : Condition Check
if(i > 0) {
This condition checks if `i` is greater than 0 to avoid out-of-bounds errors when updating the frequencies.
16 : Blank
An empty line for better readability.
17 : Prefix Sum Calculation
ca[i] += ca[i - 1];
This line accumulates the frequencies in `ca` to create a prefix sum for efficient range queries.
18 : Prefix Sum Calculation
cb[i] += cb[i - 1];
This line accumulates the frequencies in `cb` to create a prefix sum for efficient range queries.
19 : Condition Check
if(i < 25) {
This condition checks if `i` is less than 25 to ensure no out-of-bounds error occurs when accessing elements beyond the last valid index.
20 : Frequency Comparison
res = min(res, m - ca[i] + cb[i]);
This line updates `res` by considering the frequency of characters between the two strings after the prefix sums have been calculated.
21 : Frequency Comparison
res = min(res, n - cb[i] + ca[i]);
This line updates `res` again by considering the reversed situation, checking the frequency differences.
22 : Blank
An empty line for readability.
23 : Block End
}
Closing the block of code that compares the frequencies and updates the result `res`.
24 : Return
return res;
This line returns the calculated result `res`, which represents the minimum number of changes required.
Best Case: O(n + m), where `n` and `m` are the lengths of strings `a` and `b`, respectively.
Average Case: O(n + m), as we need to process both strings.
Worst Case: O(n + m), due to the same reasons above.
Description: The time complexity is linear in the length of the input strings, O(n + m), where `n` and `m` are the lengths of the strings `a` and `b`.
Best Case: O(1), as the space required does not change with the input size.
Worst Case: O(1), as the space required is constant and depends only on the size of the character frequency array (26 elements).
Description: The space complexity is constant, O(1), since we only store the frequency counts for the 26 lowercase letters.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus