Leetcode 1732: Find the Highest Altitude
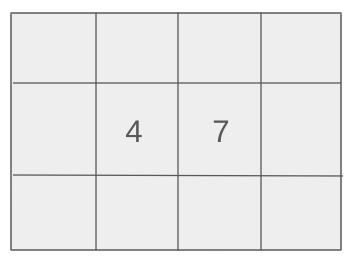
A biker is going on a road trip, where the trip consists of several points with different altitudes. The biker starts at point 0, which has an altitude of 0. You are given an array of integers
gain
, where each value represents the change in altitude between two consecutive points. Your task is to find the highest altitude the biker reaches during the trip.Problem
Approach
Steps
Complexity
Input: The input consists of a single integer array `gain` of length `n` (1 ≤ n ≤ 100), where each element of the array represents the change in altitude between two consecutive points. The biker starts at altitude 0.
Example: Input: gain = [-3, 2, 1, -4, 3]
Constraints:
• n == gain.length
• 1 <= n <= 100
• -100 <= gain[i] <= 100
Output: Return the highest altitude the biker reaches during the trip, including the starting point.
Example: Output: 3
Constraints:
• The output should be a single integer representing the highest altitude reached.
Goal: The goal is to find the highest point the biker reaches on the trip by computing the cumulative altitude after applying each gain value sequentially.
Steps:
• 1. Initialize the current altitude as 0.
• 2. Iterate through the `gain` array, updating the current altitude after each step.
• 3. Keep track of the maximum altitude reached during the journey.
• 4. Return the maximum altitude.
Goal: The array `gain` contains integer values that represent the net change in altitude between points, and the biker starts at altitude 0.
Steps:
• The array `gain` must have at least 1 element.
• The change in altitude for each step is between -100 and 100.
Assumptions:
• The biker starts at altitude 0.
• The altitude changes are applied sequentially as per the gain array.
• Input: Input: gain = [-3, 2, 1, -4, 3]
• Explanation: Starting at altitude 0, the biker reaches altitudes [-3, -1, 0, -4, 3]. The highest altitude reached is 3.
• Input: Input: gain = [2, -1, 3, -2, 0]
• Explanation: Starting at altitude 0, the biker reaches altitudes [2, 1, 4, 2, 2]. The highest altitude reached is 4.
Approach: To solve this problem, we need to simulate the biker's journey, calculating the cumulative altitude at each step while tracking the highest altitude encountered.
Observations:
• We can solve this problem in one pass through the `gain` array, keeping track of the current altitude and updating the maximum altitude reached.
• The solution requires O(n) time complexity since we are iterating through the array once.
Steps:
• 1. Initialize `currentAltitude` as 0 and `maxAltitude` as 0.
• 2. For each element in `gain`, add it to `currentAltitude` and update `maxAltitude` if `currentAltitude` exceeds it.
• 3. After iterating through the array, return `maxAltitude`.
Empty Inputs:
• An empty input array should not be possible due to the problem's constraints (n ≥ 1).
Large Inputs:
• The algorithm must be efficient enough to handle arrays with up to 100 elements.
Special Values:
• The gain array could have only negative values, in which case the highest altitude will still be 0.
Constraints:
• The solution should handle both positive and negative values in the gain array correctly.
int largestAltitude(vector<int>& gain) {
int mx = 0;
int cur = 0;
for(int x: gain) {
cur += x;
mx = max(mx, cur);
}
return mx;
}
1 : Function Definition
int largestAltitude(vector<int>& gain) {
The function `largestAltitude` is defined, taking a vector of integers `gain`, where each element represents the altitude change, as input.
2 : Initialization
int mx = 0;
The variable `mx` is initialized to 0. It will store the maximum altitude encountered during the traversal of the `gain` array.
3 : Initialization
int cur = 0;
The variable `cur` is initialized to 0. It will track the current altitude as we iterate through the `gain` array.
4 : Loop - Gain Iteration
for(int x: gain) {
A for-loop is used to iterate over each element `x` in the `gain` vector. Each `x` represents the change in altitude.
5 : Update Current Altitude
cur += x;
The current altitude `cur` is updated by adding the altitude change `x`.
6 : Update Maximum Altitude
mx = max(mx, cur);
The maximum altitude `mx` is updated to be the larger of the current maximum and the current altitude `cur`.
7 : Return Result
return mx;
The function returns `mx`, which contains the largest altitude encountered during the traversal of the `gain` array.
Best Case: O(n), since the algorithm must iterate through the entire `gain` array once.
Average Case: O(n), as there is no variation in time complexity based on input values.
Worst Case: O(n), as we process each element in the array sequentially.
Description: The time complexity is linear in terms of the length of the `gain` array.
Best Case: O(1), since no additional space is required beyond the variables for tracking the current and maximum altitude.
Worst Case: O(1), as we are only storing a few integer variables.
Description: The space complexity is constant because the solution uses only a fixed amount of extra space.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus