Leetcode 1700: Number of Students Unable to Eat Lunch
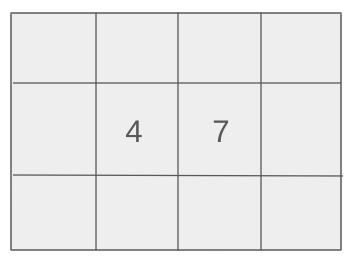
In a school cafeteria, sandwiches are placed in a stack and students queue up to get one. Each student has a preference for the type of sandwich, and they either take the sandwich from the stack or move to the back of the queue if the sandwich is not to their liking. The goal is to determine how many students are unable to get a sandwich.
Problem
Approach
Steps
Complexity
Input: The input consists of two arrays: 'students' representing the preference of each student (0 for circular, 1 for square), and 'sandwiches' representing the stack of sandwiches (with 0 for circular, 1 for square).
Example: [0, 1, 0, 1], [1, 0, 1, 0]
Constraints:
• 1 <= students.length, sandwiches.length <= 100
• students.length == sandwiches.length
• students[i] and sandwiches[i] are either 0 or 1
Output: The output should be a single integer representing the number of students who are unable to get a sandwich.
Example: 2
Constraints:
• The returned integer should be between 0 and the total number of students.
Goal: To determine how many students are unable to eat based on their preferences and the order of the sandwiches.
Steps:
• Initialize a count for each type of sandwich in the stack and for each student preference.
• Iterate over the queue of students and check if the sandwich at the top of the stack matches the student's preference.
• If the student prefers the sandwich, remove it from the stack and move the student out of the queue.
• If the student does not want the sandwich, move them to the end of the queue.
• Continue the process until no student at the front of the queue can take the sandwich at the top of the stack.
Goal: The array lengths for both students and sandwiches will always be the same, and both will contain only the values 0 and 1.
Steps:
• Both 'students' and 'sandwiches' arrays have a length between 1 and 100.
• The values in 'students' and 'sandwiches' are either 0 or 1.
Assumptions:
• There will always be enough sandwiches for each student at the start, but some may not get one by the end of the process.
• Input: [0, 1, 0, 1], [1, 0, 1, 0]
• Explanation: All students are able to take a sandwich after a few moves, resulting in no students left without food.
• Input: [1, 0, 1, 0, 1, 1], [0, 1, 1, 1, 0, 0]
• Explanation: Two students are unable to get a sandwich by the end of the process due to mismatched preferences.
Approach: The approach to solving this problem involves simulating the sandwich distribution process, keeping track of which students are still waiting for a sandwich.
Observations:
• The process involves moving students around the queue and keeping track of their preferences relative to the sandwiches on top of the stack.
• We can optimize by using a simple queue to manage the students and a counter for the sandwich types to ensure we don't perform unnecessary operations.
Steps:
• Count the number of students preferring each type of sandwich.
• Simulate the process by iterating through the students' queue and checking if the sandwich matches their preference.
• If a student can't get the sandwich they want, move them to the end of the queue.
• Stop when no student at the front of the queue can take the top sandwich.
Empty Inputs:
• The input will never be empty as there is always at least one student and one sandwich.
Large Inputs:
• The solution should efficiently handle arrays up to 100 elements in length.
Special Values:
• If all students prefer the same type of sandwich, the outcome depends on the sandwiches available in the stack.
Constraints:
• The solution must be able to handle up to 100 students and sandwiches efficiently.
int countStudents(vector<int>& A, vector<int>& B) {
int count[] = {0, 0}, n = A.size(), k;
for (int a : A)
count[a]++;
for (k = 0; k < n && count[B[k]] > 0; ++k)
count[B[k]]--;
return n - k;
}
1 : Function Definition
int countStudents(vector<int>& A, vector<int>& B) {
Defines the function `countStudents` that takes two integer vectors, `A` and `B`, representing students and their preferences, and returns the number of students in `B` who cannot find a matching student in `A`.
2 : Variable Initialization
int count[] = {0, 0}, n = A.size(), k;
Initializes an array `count` to count the occurrences of each student in array `A`, a variable `n` to store the size of array `A`, and a variable `k` for iteration.
3 : Counting Students in A
for (int a : A)
Iterates through each student in array `A` to count the occurrences of each student using the `count` array.
4 : Counting Students in A
count[a]++;
For each student `a` in array `A`, increments the corresponding count in the `count` array.
5 : Matching Students
for (k = 0; k < n && count[B[k]] > 0; ++k)
Iterates through each student in array `B` and checks if the student can be matched with a student in `A` based on the `count` array. Decreases the count if a match is found.
6 : Decreasing Count for Matches
count[B[k]]--;
If a match is found, decreases the count for student `B[k]` in the `count` array.
7 : Return Unmatched Students
return n - k;
Returns the number of unmatched students, which is the total number of students (`n`) minus the number of students that were matched (`k`).
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The algorithm processes each student and sandwich once, so the time complexity is O(n), where n is the number of students.
Best Case: O(1)
Worst Case: O(n)
Description: The space complexity is O(n) for storing the queue of students, and O(1) if the queue is not explicitly stored.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus