Leetcode 1690: Stone Game VII
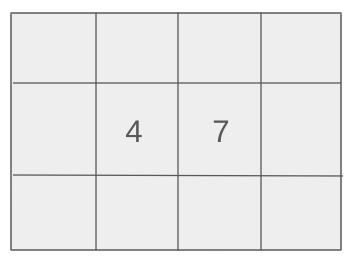
Alice and Bob are playing a game with a row of
n
stones. On each player’s turn, they can remove the leftmost or the rightmost stone, and their score is the sum of the remaining stones. Alice tries to maximize the score difference, while Bob aims to minimize it. Calculate the score difference between Alice and Bob when both play optimally.Problem
Approach
Steps
Complexity
Input: The input consists of an integer array `stones`, where each element represents the value of a stone.
Example: Input: stones = [4, 2, 7, 5, 3]
Constraints:
• 2 <= n <= 1000
• 1 <= stones[i] <= 1000
Output: The output should be an integer, representing the difference in scores between Alice and Bob.
Example: Output: 8
Constraints:
Goal: The goal is to calculate the difference in scores between Alice and Bob when both play optimally.
Steps:
• Start by calculating the total sum of the stones.
• Use dynamic programming to simulate the optimal plays of both players.
• At each step, choose either the leftmost or rightmost stone to remove and update the score accordingly.
• Track the difference in scores until all stones are removed.
Goal: The input array `stones` contains at least 2 elements, and each element is between 1 and 1000.
Steps:
• 2 <= n <= 1000
• 1 <= stones[i] <= 1000
Assumptions:
• Both players always play optimally.
• Input: Input: stones = [4, 2, 7, 5, 3]
• Explanation: The game proceeds through multiple turns, with Alice and Bob alternately removing stones. The final score difference is the result of their optimal decisions.
Approach: To solve this problem, we will use dynamic programming to simulate the game, where we compute the optimal score difference for each possible subarray of stones.
Observations:
• The players make decisions based on the remaining stones in the row.
• We can use a memoization table to store intermediate results and avoid redundant calculations.
Steps:
• Start with an array of stones and calculate the total sum of all stones.
• Create a memoization table to store the difference in score for each subarray of stones.
• Use dynamic programming to compute the score difference for each subarray by considering removing the leftmost or rightmost stone.
Empty Inputs:
• The input will always have at least two stones, so empty inputs are not possible.
Large Inputs:
• Ensure the algorithm can handle the maximum input size of `n = 1000`.
Special Values:
• When there are only two stones, the game will involve removing both stones in turn.
Constraints:
• The input will always be a valid array of integers as per the given constraints.
int memo[1001][1001] = {};
int dp(vector<int>& s, int i, int j, int sum) {
if(i == j) {
return 0;
}
return memo[i][j] ? memo[i][j] : memo[i][j] = max(sum - s[i] - dp(s, i + 1, j, sum - s[i]),
sum - s[j] - dp(s, i, j - 1, sum - s[j]));
}
int stoneGameVII(vector<int>& s) {
int n = s.size();
return dp(s, 0,n-1, accumulate(begin(s), end(s), 0));
}
1 : Memoization Table Initialization
int memo[1001][1001] = {};
Initialize a memoization table `memo` to store the results of subproblems. This avoids recalculating the same subproblems multiple times and speeds up the solution using dynamic programming.
2 : DP Function Definition
int dp(vector<int>& s, int i, int j, int sum) {
Define the recursive dynamic programming function `dp` that computes the maximum score between the indices `i` and `j` of the array `s`, with the remaining total `sum` of the stones.
3 : Base Case Check
if(i == j) {
Check if there is only one stone left (i.e., `i == j`). In this case, no more matches can be made, and the score is 0.
4 : Base Case Return
return 0;
Return 0 as there are no more stones to remove, so no points can be gained.
5 : Memoization Check
return memo[i][j] ? memo[i][j] : memo[i][j] = max(sum - s[i] - dp(s, i + 1, j, sum - s[i]),
Check if the result for the current subproblem (`i`, `j`) is already computed and stored in `memo`. If not, calculate it by choosing the optimal move: remove the stone at index `i` or `j`.
6 : Recursive Call (Left Stone Removed)
sum - s[i] - dp(s, i + 1, j, sum - s[i]),
Make a recursive call to calculate the score if the leftmost stone (`s[i]`) is removed. The remaining sum of stones is updated accordingly.
7 : Recursive Call (Right Stone Removed)
sum - s[j] - dp(s, i, j - 1, sum - s[j]));
Make a recursive call to calculate the score if the rightmost stone (`s[j]`) is removed. The remaining sum of stones is updated accordingly.
8 : Main Function Definition
int stoneGameVII(vector<int>& s) {
Define the main function `stoneGameVII` that initializes the memoization table and calls the recursive `dp` function to calculate the maximum score.
9 : Size Calculation
int n = s.size();
Calculate the size `n` of the input array `s`, which represents the number of stones.
10 : Final Calculation and Return
return dp(s, 0, n - 1, accumulate(begin(s), end(s), 0));
Call the `dp` function with the initial indices `0` and `n - 1` and the total sum of all stones (calculated using `accumulate`). Return the result of the dynamic programming computation.
Best Case: O(n^2)
Average Case: O(n^2)
Worst Case: O(n^2)
Description: The time complexity is O(n^2) because we compute the score difference for each subarray using dynamic programming.
Best Case: O(n^2)
Worst Case: O(n^2)
Description: The space complexity is O(n^2) due to the memoization table used to store the score differences for subarrays.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus