Leetcode 1688: Count of Matches in Tournament
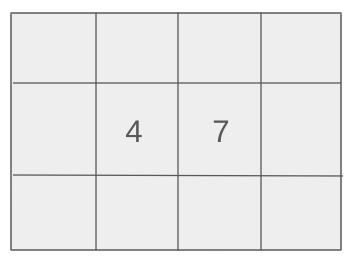
In a tournament with
n
teams, each round involves pairing teams for matches. The number of matches depends on whether n
is even or odd. Calculate the total number of matches played in the tournament until a winner is determined.Problem
Approach
Steps
Complexity
Input: The input consists of a single integer `n`, the number of teams in the tournament.
Example: Input: n = 9
Constraints:
• 1 <= n <= 200
Output: The output should be a single integer representing the total number of matches played in the tournament.
Example: Output: 8
Constraints:
Goal: The goal is to calculate the total number of matches played during the entire tournament, taking into account the number of teams in each round.
Steps:
• If the number of teams is even, `n / 2` matches are played and `n / 2` teams advance.
• If the number of teams is odd, `(n - 1) / 2` matches are played and `(n - 1) / 2 + 1` teams advance.
• Repeat this process until only one team remains, counting the matches at each step.
Goal: The input number of teams `n` will be within the given bounds.
Steps:
• 1 <= n <= 200
Assumptions:
• At least one team is always present in the tournament.
• Input: Input: n = 9
• Explanation: The tournament progresses through multiple rounds. In each round, the number of matches is determined based on whether the number of teams is even or odd. The total matches are accumulated as we move towards the final winner.
Approach: To solve the problem, simulate the tournament by calculating the number of matches played in each round based on the current number of teams. Keep track of the total number of matches until only one team is left.
Observations:
• The number of matches in each round is simple to calculate based on whether the number of teams is even or odd.
• We can use recursion or iteration to repeatedly reduce the number of teams and accumulate the match count until we reach the final winner.
Steps:
• Start with the initial number of teams `n`.
• In each round, calculate the number of matches, reduce the number of teams, and add the match count to the total.
• Repeat this process until only one team remains.
Empty Inputs:
• The input `n` should always be a valid positive integer, so empty inputs will not be encountered.
Large Inputs:
• The algorithm must efficiently handle the maximum number of teams, which is 200.
Special Values:
• If `n` is 1, no matches are played, and the total matches is 0.
Constraints:
• Ensure that the solution works within the constraint `1 <= n <= 200`.
int numberOfMatches(int n) {
if(n==0) return 0;
if(n==1) return 0;
int ans = n / 2;
if(n & 1)
ans += numberOfMatches(n/2 + 1);
else
ans += numberOfMatches(n/2);
return ans;
}
1 : Function Definition
int numberOfMatches(int n) {
Define the function 'numberOfMatches' that takes an integer `n`, representing the number of players, and returns the total number of matches in the tournament.
2 : Base Case 1
if(n==0) return 0;
Base case: If there are no players (`n == 0`), return 0 matches as there are no players to compete.
3 : Base Case 2
if(n==1) return 0;
Base case: If there is only one player (`n == 1`), return 0 matches as a single player cannot compete.
4 : Initialize Answer
int ans = n / 2;
Initialize the answer `ans` as the integer division of `n` by 2, representing the matches in the current round (each match eliminates one player).
5 : Odd Players Check
if(n & 1)
Check if the number of players `n` is odd using bitwise AND (`n & 1`). If `n` is odd, there will be one player left after the matches in the current round.
6 : Recursive Call (Odd Players)
ans += numberOfMatches(n/2 + 1);
If the number of players is odd, recursively call `numberOfMatches` with `n/2 + 1` players, as one player will have a 'bye' and not participate in the current round.
7 : Else Condition
else
If the number of players is even, proceed to the next step without any players getting a 'bye'.
8 : Recursive Call (Even Players)
ans += numberOfMatches(n/2);
If the number of players is even, recursively call `numberOfMatches` with `n/2` players, as there are no 'byes' in this round.
9 : Return Statement
return ans;
Return the total number of matches calculated for the current round and all subsequent rounds.
Best Case: O(log n)
Average Case: O(log n)
Worst Case: O(log n)
Description: The time complexity is O(log n) because the number of teams is halved in each round.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is O(1) since the solution uses constant space.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus