Leetcode 1678: Goal Parser Interpretation
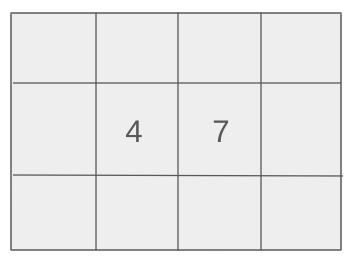
You are given a string
command
consisting of the characters ‘G’, ‘()’, and ‘(al)’ in some order. The Goal Parser interprets the following patterns: ‘G’ as the string ‘G’, ‘()’ as the string ‘o’, and ‘(al)’ as the string ‘al’. Return the interpreted string after replacing all instances of ‘()’, ‘(al)’, and ‘G’ according to the above rules.Problem
Approach
Steps
Complexity
Input: The input consists of a string `command` that can contain the characters 'G', '()', and '(al)'.
Example: command = 'G()(al)'
Constraints:
• 1 <= command.length <= 100
• command consists of 'G', '()', and/or '(al)' in some order.
Output: Return the interpreted string after replacing '()', '(al)', and 'G' according to the specified rules.
Example: Output: 'Goal'
Constraints:
Goal: The goal is to convert the input string into a new string based on the specified patterns.
Steps:
• Iterate through the input string `command` and check each character or sequence of characters.
• If 'G' is encountered, append 'G' to the result.
• If '()' is encountered, append 'o' to the result.
• If '(al)' is encountered, append 'al' to the result.
• Return the concatenated result.
Goal: The input string `command` satisfies the following constraints:
Steps:
• The length of `command` is between 1 and 100.
• The string `command` consists of 'G', '()', and/or '(al)' in some order.
Assumptions:
• The input string `command` will always be valid and will follow the given structure.
• Input: Input: command = 'G()(al)'
• Explanation: The Goal Parser interprets 'G' as 'G', '()' as 'o', and '(al)' as 'al', resulting in the concatenated string 'Goal'.
Approach: To solve this problem, we need to scan through the input string `command`, identify the patterns, and replace them with their corresponding interpretations.
Observations:
• The string can be interpreted by scanning for specific patterns ('G', '()', and '(al)').
• We can use a loop to iterate through the string and handle the patterns accordingly.
Steps:
• Initialize an empty result string.
• Iterate through the input string character by character.
• For each character or sequence, append the corresponding interpretation to the result.
• Return the final concatenated result.
Empty Inputs:
• The input string will always have at least one character.
Large Inputs:
• The input size will not exceed 100 characters, so performance concerns are minimal.
Special Values:
• There are no special values other than the given patterns 'G', '()', and '(al)'.
Constraints:
• The input string will always consist of valid characters.
string interpret(string command) {
string result= "";
for(int i=0; i<command.size(); i++)
{
if(command[i] == '(')
{
if(command[i+1] == ')')
result += "o";
if(command[i+1] == 'a' && command[i+2] == 'l')
{
result += "al";
i += 2;
}
}
if(command[i] == 'G')
result += "G";
}
return result;
}
1 : Function Definition
string interpret(string command) {
Define the function 'interpret' that takes a string 'command' as input and returns a modified string.
2 : Variable Initialization
string result= "";
Initialize an empty string 'result' to store the final modified output.
3 : Loop Setup
for(int i=0; i<command.size(); i++)
Start a loop to iterate over each character in the input string 'command'.
4 : Condition Check
if(command[i] == '(')
Check if the current character is an opening parenthesis '(' to begin interpreting a command.
5 : Condition Check
if(command[i+1] == ')')
Check if the next character is a closing parenthesis ')'. If so, it represents '()' and we append 'o' to the result.
6 : String Manipulation
result += "o";
Append 'o' to the 'result' string when encountering the '()' pattern.
7 : Condition Check
if(command[i+1] == 'a' && command[i+2] == 'l')
Check if the characters after '(' are 'al'. If so, append 'al' to the result and skip the next two characters.
8 : String Manipulation
result += "al";
Append 'al' to the 'result' string when encountering the '(al)' pattern.
9 : Variable Update
i += 2;
Skip the next two characters ('a' and 'l') after processing the '(al)' pattern.
10 : Condition Check
if(command[i] == 'G')
Check if the current character is 'G'. If so, append 'G' to the result.
11 : String Manipulation
result += "G";
Append 'G' to the 'result' string when encountering the 'G' character.
12 : Return Statement
return result;
Return the final modified string 'result'.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The algorithm iterates through the string once, processing each character or sequence in constant time.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) due to the space used to store the resulting string.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus