Leetcode 1674: Minimum Moves to Make Array Complementary
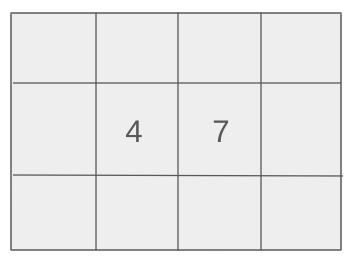
You are given an integer array
nums
of even length n
and an integer limit
. In one move, you can replace any element of nums
with another integer between 1
and limit
(inclusive). The array nums
is complementary if for all indices i
, nums[i] + nums[n - 1 - i]
equals the same number. Return the minimum number of moves required to make nums
complementary.Problem
Approach
Steps
Complexity
Input: The input consists of an integer array `nums` of even length `n` and an integer `limit`.
Example: nums = [1, 2, 4, 3], limit = 4
Constraints:
• 2 <= n <= 10^5
• 1 <= nums[i] <= limit <= 10^5
• n is even
Output: Return the minimum number of moves required to make the array complementary.
Example: Output: 1
Constraints:
Goal: To determine the minimum number of moves to make `nums` complementary, we need to adjust pairs of elements such that their sums are consistent across the array.
Steps:
• Iterate over the first half of the array and consider pairs of elements from the beginning and the end of the array.
• For each pair of elements, calculate the range of possible sums (from their minimum to their maximum possible sum).
• Track the frequency of each sum using a differential array.
• Determine the minimum number of moves required by finding the sum with the smallest number of adjustments.
Goal: The input array `nums` has an even length `n` and satisfies the following constraints:
Steps:
• The length of `nums` is between 2 and 100,000.
• Each element in `nums` is between 1 and `limit`.
• The value of `limit` is between 1 and 100,000.
• The length of `nums` is even.
Assumptions:
• The input array `nums` will always have an even length.
• The value of `limit` will be valid and within the given constraints.
• Input: Input: nums = [1, 2, 4, 3], limit = 4
• Explanation: In this case, we need to change `nums` to make the sum of each pair equal to 4. This can be achieved by changing the second element `4` to `2`.
Approach: To solve this problem, we can use a greedy approach with a differential array to track the changes required for each possible sum of the pairs.
Observations:
• We need to find the optimal sum for each pair and count the number of changes required to make all sums equal.
• Using a differential array will allow us to efficiently track the changes needed for each sum.
• We need to ensure that we minimize the total number of changes, which can be done by finding the most frequent sum and adjusting all pairs to match that sum.
Steps:
• Initialize a differential array to track the changes required for each sum.
• For each pair of elements, calculate their possible sum range.
• Update the differential array with the number of changes required for each sum.
• Iterate through the differential array to find the sum with the smallest number of changes.
Empty Inputs:
• The array `nums` will always have an even length, so no empty input scenarios are considered.
Large Inputs:
• The solution should be optimized to handle input arrays up to the size of 100,000 efficiently.
Special Values:
• The array elements and the limit will always be within the range of 1 to 100,000.
Constraints:
• The value of `limit` must be between 1 and 100,000, and each element in the array `nums` must be between 1 and `limit`.
int minMoves(vector<int>& num, int lmt) {
int n = num.size();
vector<int> dp(2*lmt +2,0);
for(int i = 0; i < n/2; i++) {
int a = num[i];
int b = num[n-1-i];
dp[2] += 2;
dp[min(a,b)+1] -= 1;
dp[a+b] -= 1;
dp[a+b+1] += 1;
dp[max(a,b) + lmt +1] += 1;
}
//for(int i = 1; i < n; i++)
// dp[i] += dp[i - 1];
int cur = 0, res = 2* n;
for(int i = 2; i < 2 * lmt + 1; i++) {
cur += dp[i];
res = min(res, cur);
}
return res;
}
1 : Function Definition
int minMoves(vector<int>& num, int lmt) {
Define the function 'minMoves' that accepts a reference to a vector of integers 'num' and an integer 'lmt' representing the limit for the calculation.
2 : Variable Initialization
int n = num.size();
Store the size of the input array 'num' in variable 'n'.
3 : Dynamic Programming Array Initialization
vector<int> dp(2*lmt +2,0);
Initialize a dynamic programming array 'dp' of size '2*lmt + 2' with all elements set to 0.
4 : Loop Setup
for(int i = 0; i < n/2; i++) {
Start a loop to iterate through the first half of the array, where the element pairs are compared.
5 : Variable Assignment
int a = num[i];
Assign the element at the 'i'-th index of the array 'num' to the variable 'a'.
6 : Variable Assignment
int b = num[n-1-i];
Assign the element at the corresponding index from the end of the array 'num' to the variable 'b'.
7 : Dynamic Programming Update
dp[2] += 2;
Increase the value at index 2 in the dynamic programming array 'dp' by 2.
8 : Dynamic Programming Update
dp[min(a,b)+1] -= 1;
Decrease the value at index 'min(a,b) + 1' in the DP array 'dp' by 1 to track a move.
9 : Dynamic Programming Update
dp[a+b] -= 1;
Decrease the value at index 'a + b' in the DP array 'dp' by 1 to track a move based on the sum of 'a' and 'b'.
10 : Dynamic Programming Update
dp[a+b+1] += 1;
Increase the value at index 'a + b + 1' in the DP array 'dp' by 1 to adjust the count based on the sum of 'a' and 'b'.
11 : Dynamic Programming Update
dp[max(a,b) + lmt +1] += 1;
Increase the value at index 'max(a,b) + lmt + 1' in the DP array 'dp' by 1, considering the maximum value between 'a' and 'b'.
12 : Variable Initialization
int cur = 0, res = 2* n;
Initialize variables 'cur' to 0 and 'res' to '2 * n'. 'cur' will hold the current cumulative sum of DP values, and 'res' will store the minimum result.
13 : Final Loop
for(int i = 2; i < 2 * lmt + 1; i++) {
Start a loop to iterate over the DP array from index 2 to '2 * lmt + 1' to find the minimum number of moves.
14 : Dynamic Programming Update
cur += dp[i];
Update the variable 'cur' by adding the value from the DP array at index 'i'.
15 : Result Calculation
res = min(res, cur);
Update the result 'res' by taking the minimum between the current 'res' and 'cur'. This keeps track of the least number of moves.
16 : Return Statement
return res;
Return the final result 'res', which holds the minimum number of moves required.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The algorithm performs a linear scan of the array and updates the differential array for each pair of elements, leading to O(n) time complexity.
Best Case: O(limit)
Worst Case: O(limit)
Description: The space complexity is proportional to the size of the `limit` because we maintain a differential array for sums.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus