Leetcode 1673: Find the Most Competitive Subsequence
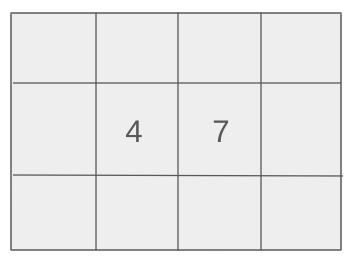
Given an integer array
nums
and a positive integer k
, return the most competitive subsequence of nums
of size k
. A subsequence is more competitive than another if at the first position where they differ, the subsequence has a smaller number.Problem
Approach
Steps
Complexity
Input: The input consists of an array of integers `nums` and an integer `k` which is the size of the subsequence.
Example: nums = [6, 3, 4, 5], k = 2
Constraints:
• 1 <= nums.length <= 10^5
• 0 <= nums[i] <= 10^9
• 1 <= k <= nums.length
Output: Return the most competitive subsequence of size `k`.
Example: Output: [3, 5]
Constraints:
Goal: Find the most competitive subsequence by maintaining a stack and ensuring that subsequences are in increasing order.
Steps:
• Initialize an empty stack.
• Iterate through each element of the array `nums`.
• For each element, pop elements from the stack if they are greater than the current element and if there are enough remaining elements to form a subsequence of size `k`.
• Push the current element to the stack if the size of the stack is less than `k`.
• After iterating through all elements, return the stack as the result.
Goal: The input will always satisfy the following constraints:
Steps:
• The length of the input list `nums` will be between 1 and 100,000.
• Each element in the list will be between 0 and 1 billion.
• The subsequence size `k` will always be less than or equal to the length of `nums`.
Assumptions:
• The input array `nums` will have at least one element.
• The value of `k` will be a valid integer between 1 and the length of `nums`.
• Input: Input: nums = [6, 3, 4, 5], k = 2
• Explanation: Among all possible subsequences of size 2, [3, 5] is the most competitive because the first position where the subsequences differ is at the second position, and 3 is smaller than 4 and 5.
Approach: We can solve this problem using a greedy approach with a stack. As we iterate through the array, we ensure that the stack contains the most competitive subsequence by removing larger elements when necessary.
Observations:
• We need to select `k` elements that form the most competitive subsequence.
• Using a stack allows us to efficiently maintain the subsequence in increasing order.
• We need to ensure that at each point we are maintaining the smallest possible elements in the subsequence, using the stack to enforce this.
Steps:
• Initialize an empty stack.
• Iterate through the list `nums`.
• If the stack is not empty and the current element is smaller than the top element of the stack, and there are enough remaining elements to form a subsequence of size `k`, pop the stack.
• Push the current element to the stack if the size of the stack is less than `k`.
• Return the stack as the final subsequence.
Empty Inputs:
• The input will always contain at least one element.
Large Inputs:
• The algorithm should handle inputs up to 100,000 elements efficiently.
Special Values:
• The array elements can be as large as 1 billion.
Constraints:
vector<int> mostCompetitive(vector<int>& nums, int k) {
vector<int> stk;
for(int i = 0; i < nums.size(); i++) {
while (!stk.empty() &&
stk.back() > nums[i] &&
(stk.size() + nums.size() - (i + 1)) >= k )
stk.pop_back();
if(stk.size() < k)
stk.push_back(nums[i]);
}
return stk;
}
1 : Variable Initialization
vector<int> mostCompetitive(vector<int>& nums, int k) {
Define the function mostCompetitive that takes a reference to a vector of integers 'nums' and an integer 'k' as input. The function will return a vector of integers representing the most competitive subsequence.
2 : Stack Initialization
vector<int> stk;
Initialize an empty vector 'stk' that will be used as a stack to store elements from 'nums' in the most competitive order.
3 : Looping Through Elements
for(int i = 0; i < nums.size(); i++) {
Start a loop to iterate through each element in the 'nums' array.
4 : Stack Operations
while (!stk.empty() && stk.back() > nums[i] && (stk.size() + nums.size() - (i + 1)) >= k )
Inside the loop, use a while loop to check if the stack is not empty, the top element of the stack is greater than the current element, and there are enough remaining elements to form a valid subsequence of length 'k'.
5 : Pop Operation
stk.pop_back();
If the above conditions are true, pop the top element from the stack to make room for potentially smaller and more competitive elements.
6 : Conditional Insertion
if(stk.size() < k)
Check if the current size of the stack is less than 'k', meaning there is space to add more elements.
7 : Push Operation
stk.push_back(nums[i]);
If there is space in the stack (i.e., the stack's size is less than 'k'), push the current element onto the stack.
8 : Return Statement
return stk;
After the loop finishes, return the stack, which now contains the most competitive subsequence of length 'k'.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: We iterate through the list once, and each element is pushed and popped from the stack at most once.
Best Case: O(k)
Worst Case: O(k)
Description: The space complexity is proportional to the size of the subsequence `k`.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus