Leetcode 1662: Check If Two String Arrays are Equivalent
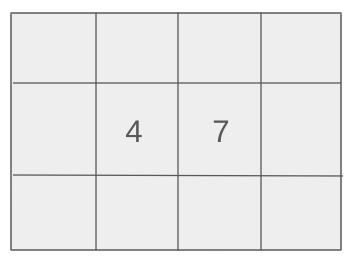
You are given two string arrays
word1
and word2
. Return true
if these two arrays represent the same string when their elements are concatenated in order, otherwise return false
.Problem
Approach
Steps
Complexity
Input: You are given two arrays of strings, `word1` and `word2`.
Example: word1 = ["hello", "world"], word2 = ["hell", "oworld"]
Constraints:
• 1 <= word1.length, word2.length <= 10^3
• 1 <= word1[i].length, word2[i].length <= 10^3
• 1 <= sum(word1[i].length), sum(word2[i].length) <= 10^3
• word1[i] and word2[i] consist of lowercase letters.
Output: Return `true` if the two arrays represent the same string, otherwise return `false`.
Example: true
Constraints:
• The output will be a boolean value.
Goal: Check if the concatenated strings from `word1` and `word2` are equal.
Steps:
• Concatenate all strings in `word1` into a single string `str1`.
• Concatenate all strings in `word2` into a single string `str2`.
• Compare the two resulting strings and return `true` if they are equal, otherwise return `false`.
Goal: The constraints ensure the solution can handle large string arrays efficiently.
Steps:
• 1 <= word1.length, word2.length <= 10^3
• 1 <= word1[i].length, word2[i].length <= 10^3
• 1 <= sum(word1[i].length), sum(word2[i].length) <= 10^3
Assumptions:
• The arrays `word1` and `word2` will only contain lowercase letters.
• Input: word1 = ["hello", "world"], word2 = ["hell", "oworld"]
• Explanation: Both `word1` and `word2` form the string "helloworld" when concatenated, so the answer is `true`.
• Input: word1 = ["abc", "def"], word2 = ["abcd", "ef"]
• Explanation: The strings formed by `word1` and `word2` are "abcdef" and "abcde", respectively, which are not equal. Therefore, return `false`.
Approach: We need to compare the concatenated strings from `word1` and `word2` and check if they are identical.
Observations:
• We can solve this by converting both arrays into strings and then comparing them.
• Iterate through both arrays, concatenate their elements into two separate strings, and compare the results.
Steps:
• Initialize two empty strings, `str1` and `str2`.
• Concatenate all elements from `word1` to `str1`.
• Concatenate all elements from `word2` to `str2`.
• If `str1` is equal to `str2`, return `true`; otherwise, return `false`.
Empty Inputs:
• If either `word1` or `word2` is empty, return `false` if the other is not empty.
Large Inputs:
• The solution should efficiently handle arrays with a total length of up to 1000 characters.
Special Values:
• If the strings formed from both arrays are the same, return `true`.
Constraints:
• The total length of the strings in both arrays will not exceed 1000 characters.
bool arrayStringsAreEqual(vector<string>& word1, vector<string>& word2) {
string a,b;
for(auto x:word1)
a += x;
for(auto x:word2)
b += x;
if(a == b)
return 1;
return 0;
}
1 : Function Definition
bool arrayStringsAreEqual(vector<string>& word1, vector<string>& word2) {
Defines the function `arrayStringsAreEqual` that takes two arrays of strings and returns a boolean indicating if their concatenated forms are equal.
2 : Variable Initialization
string a,b;
Initializes two strings `a` and `b` to store concatenated results of the arrays `word1` and `word2`.
3 : Looping
for(auto x:word1)
Iterates through each string in `word1` to construct the concatenated string `a`.
4 : String Concatenation
a += x;
Appends the current string `x` from `word1` to the concatenated string `a`.
5 : Looping
for(auto x:word2)
Iterates through each string in `word2` to construct the concatenated string `b`.
6 : String Concatenation
b += x;
Appends the current string `x` from `word2` to the concatenated string `b`.
7 : Condition Check
if(a == b)
Checks if the concatenated strings `a` and `b` are equal.
8 : Return
return 1;
Returns `true` (1) if the concatenated strings are equal.
9 : Return
return 0;
Returns `false` (0) if the concatenated strings are not equal.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n) since we concatenate and compare strings, where n is the total number of characters across both arrays.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) due to the storage of concatenated strings.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus