Leetcode 1658: Minimum Operations to Reduce X to Zero
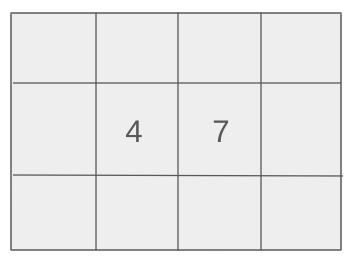
You are given an array of integers
nums
and an integer x
. In each operation, you can either remove the leftmost or rightmost element from the array and subtract its value from x
. The task is to determine the minimum number of operations required to reduce x
to exactly 0, or return -1 if it’s not possible.Problem
Approach
Steps
Complexity
Input: You are given two inputs: an integer array `nums` and an integer `x`.
Example: nums = [2, 3, 1, 4, 1], x = 5
Constraints:
• 1 <= nums.length <= 10^5
• 1 <= nums[i] <= 10^4
• 1 <= x <= 10^9
Output: Return the minimum number of operations needed to reduce `x` to 0, or -1 if it's not possible.
Example: 2
Constraints:
• The output will be a single integer: either the minimum number of operations or -1.
Goal: Determine the minimum number of operations to reduce `x` to 0, by removing elements from either end of the array and subtracting their value from `x`.
Steps:
• Calculate the total sum of elements in `nums`. If the sum is smaller than `x`, return -1 immediately.
• Use a sliding window approach to check the sum of subarrays of `nums` that can contribute to reducing `x` to 0.
• Keep track of the longest subarray that matches the condition of reducing `x` to 0, and calculate the number of operations.
Goal: The constraints are designed to ensure the solution can handle large arrays and large values of `x` efficiently.
Steps:
• 1 <= nums.length <= 10^5
• 1 <= nums[i] <= 10^4
• 1 <= x <= 10^9
Assumptions:
• The array `nums` contains only positive integers.
• The array has a length between 1 and 100,000, and `x` can be as large as 1 billion.
• Input: nums = [2, 3, 1, 4, 1], x = 5
• Explanation: In this case, we can remove the last two elements (4 + 1 = 5) to reduce `x` to 0, requiring exactly 2 operations.
• Input: nums = [4, 5, 6, 7, 8], x = 3
• Explanation: In this case, it’s impossible to remove elements from the array to sum to `x = 3`. Hence, the answer is -1.
Approach: We will use a sliding window approach to find the longest subarray that can reduce `x` to 0 by removing elements from the array.
Observations:
• The problem can be reduced to finding a subarray whose sum is equal to `sum(nums) - x`.
• Using a sliding window or prefix sum technique will help optimize the solution for large inputs.
• Start by checking if the sum of all elements in `nums` is smaller than `x`. If it is, return -1 immediately.
• Otherwise, calculate the difference between the sum of the array and `x`.
Steps:
• Compute the total sum of elements in `nums`.
• Calculate the target sum as `total_sum - x`.
• Use a sliding window or prefix sum approach to find the longest subarray with the target sum.
• If such a subarray is found, the number of operations is `n - length of the subarray`. If no such subarray exists, return -1.
Empty Inputs:
• If `nums` is empty, return -1 immediately.
Large Inputs:
• For large arrays, ensure that the algorithm handles the input within acceptable time limits.
Special Values:
• If `x` is greater than the sum of all elements in `nums`, return -1.
Constraints:
• Ensure that the solution works efficiently with up to 100,000 elements.
int minOperations(vector<int>& nums, int x) {
int res = -1;
long sum = -x;
for(int y: nums) sum += y;
int n = nums.size();
if (sum == 0) return n;
map<int, int> mp;
mp[0] = -1;
int s = 0;
for(int i = 0; i < n; i++) {
s += nums[i];
if(mp.count(s - sum)) {
res = max(res, i - mp[s - sum]);
}
mp[s] = i;
}
return res == -1? res :n - res;
}
1 : Function Definition
int minOperations(vector<int>& nums, int x) {
Defines the function `minOperations` which takes an array and a target value as inputs.
2 : Variable Initialization
int res = -1;
Initializes the result variable `res` to -1 to track the maximum subarray length.
3 : Variable Initialization
long sum = -x;
Initializes `sum` to -x to adjust the target for finding a subarray with a specific sum.
4 : Looping
for(int y: nums) sum += y;
Calculates the total sum of the array and adjusts `sum` for further processing.
5 : Variable Initialization
int n = nums.size();
Stores the size of the array in `n` for use in subsequent logic.
6 : Condition Check
if (sum == 0) return n;
Checks if the total sum equals `x`. If true, the entire array is the solution.
7 : Map Initialization
map<int, int> mp;
Initializes a map to store prefix sums and their corresponding indices.
8 : Map Update
mp[0] = -1;
Adds an initial entry to the map for handling prefix sums starting from the beginning.
9 : Variable Initialization
int s = 0;
Initializes the prefix sum variable `s` to zero.
10 : Looping
for(int i = 0; i < n; i++) {
Iterates through the array to compute prefix sums and check for subarray matches.
11 : Sum Update
s += nums[i];
Updates the prefix sum `s` with the current element.
12 : Condition Check
if(mp.count(s - sum)) {
Checks if there exists a prefix sum in the map such that the difference equals the target sum.
13 : Update Result
res = max(res, i - mp[s - sum]);
Updates `res` to the maximum length of a valid subarray found so far.
14 : Map Update
mp[s] = i;
Adds the current prefix sum and its index to the map for future reference.
15 : Return
return res == -1? res :n - res;
Returns the minimum operations needed by converting the subarray length to elements removed.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is linear because we scan the array once using the sliding window technique.
Best Case: O(1)
Worst Case: O(n)
Description: The space complexity is O(n) due to the storage of intermediate results like prefix sums or hash maps.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus