Leetcode 1656: Design an Ordered Stream
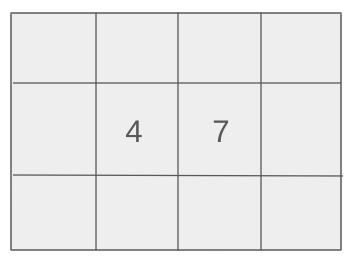
You are given a stream of
n
(id, value) pairs, where id
is an integer between 1 and n
, and value
is a string. The task is to design a stream that returns the values in increasing order of their id
. After each insertion, the stream should return the largest possible chunk of values that appear next in the order.Problem
Approach
Steps
Complexity
Input: The input consists of a series of operations, where the first operation is to create an OrderedStream of size `n`. Subsequent operations insert `id` and `value` pairs into the stream.
Example: Input: ["OrderedStream", "insert", "insert", "insert", "insert", "insert"] [[5], [3, "ccccc"], [1, "aaaaa"], [2, "bbbbb"], [5, "eeeee"], [4, "ddddd"]]
Constraints:
• 1 <= n <= 1000
• 1 <= id <= n
• value.length == 5
• value consists only of lowercase letters
• Each call to insert will have a unique id
• Exactly n calls will be made to insert
Output: The output should be a list of lists, where each list represents the chunk of values returned after each insertion.
Example: Output: [null, [], ["aaaaa"], ["bbbbb", "ccccc"], [], ["ddddd", "eeeee"]]
Constraints:
Goal: The goal is to keep track of the inserted values and return the ordered chunks after each insertion.
Steps:
• Initialize a stream of size n.
• For each insert operation, place the value at the appropriate index in the stream.
• After each insertion, check the values in the stream from the current insertion point to the next possible value in the order and return the largest chunk.
Goal: The solution must handle up to 1000 insertions efficiently.
Steps:
• 1 <= n <= 1000
• Each value has a length of 5 characters and only contains lowercase letters.
Assumptions:
• The insertions will be done in arbitrary order, and each insertion will have a unique id.
• Input: Input: ["OrderedStream", "insert", "insert", "insert", "insert", "insert"] [[5], [3, "ccccc"], [1, "aaaaa"], [2, "bbbbb"], [5, "eeeee"], [4, "ddddd"]]
• Explanation: The insertions happen in an arbitrary order, but the values are returned in the order of their ids. Chunks are returned each time an insertion happens, and they contain the values in increasing id order.
Approach: The approach involves maintaining a stream of values and ensuring that after each insertion, the largest possible chunk of sorted values is returned.
Observations:
• The values must be inserted and then returned in sorted order based on their id. We need to track the next expected id in the stream.
• Using a pointer to track the next expected id and dynamically creating chunks as values are inserted seems like an optimal approach.
Steps:
• Initialize a list to represent the stream.
• Track the next expected value to insert, and use a pointer to return chunks.
• For each insert, place the value in its correct position and then collect values in order, returning the largest chunk.
Empty Inputs:
• An edge case would be when the stream is initialized with `n = 1`.
Large Inputs:
• Test the algorithm with the maximum possible size of `n = 1000`.
Special Values:
• Consider inputs where values are inserted in reverse order and ensure the correct chunk is returned.
Constraints:
• Ensure the solution works efficiently for the maximum constraint sizes.
vector<string> s;
int ptr = 1;
OrderedStream(int n) : s(n + 1) {}
vector<string> insert(int id, string value) {
s[id] = value;
vector<string> res;
while (ptr < s.size() && !s[ptr].empty())
res.push_back(s[ptr++]);
return res;
}
};
/**
* Your OrderedStream object will be instantiated and called as such:
* OrderedStream* obj = new OrderedStream(n);
* vector<string> param_1 = obj->insert(idKey,value);
1 : Variable Declaration
vector<string> s;
Declares a vector of strings to store the stream elements.
2 : Pointer Initialization
int ptr = 1;
Initializes a pointer to track the next expected element in the ordered stream.
3 : Constructor
OrderedStream(int n) : s(n + 1) {}
Defines the constructor for the `OrderedStream` class, initializing the vector with a size of `n + 1`.
4 : Method Declaration
vector<string> insert(int id, string value) {
Begins the `insert` method, which inserts a value into the stream at the specified ID and returns all consecutive values starting from the pointer.
5 : Array Insertion
s[id] = value;
Inserts the given value into the stream at the specified index.
6 : Variable Declaration
vector<string> res;
Declares a vector to store the result of consecutive values.
7 : While Loop
while (ptr < s.size() && !s[ptr].empty())
Loops through the stream to collect consecutive values starting from the pointer.
8 : Push Back
res.push_back(s[ptr++]);
Adds the current value at the pointer to the result and increments the pointer.
9 : Return Statement
return res;
Returns the collected consecutive values as the result.
Best Case: O(1)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity for each insert operation is O(n) in the worst case, as we may need to check all values to form the chunk.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) due to the storage of values in the stream.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus