Leetcode 1646: Get Maximum in Generated Array
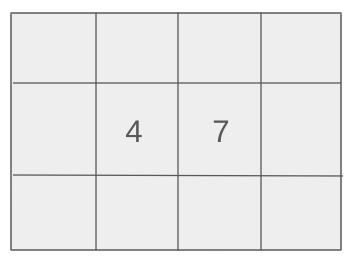
Given an integer n, an array nums of length n+1 is generated with the following rules:
- nums[0] = 0
- nums[1] = 1
- nums[2 * i] = nums[i] when 2 <= 2 * i <= n
- nums[2 * i + 1] = nums[i] + nums[i + 1] when 2 <= 2 * i + 1 <= n
Return the maximum value in the array nums.
Problem
Approach
Steps
Complexity
Input: An integer n.
Example: n = 6
Constraints:
• 0 <= n <= 100
Output: Return the maximum integer in the generated array.
Example: Output: 3
Constraints:
Goal: Generate the array nums based on the given rules and find the maximum integer in the array.
Steps:
• Initialize an array nums of length n+1.
• Iterate through the array to generate values based on the given rules.
• Return the maximum value in the array.
Goal: The integer n must be between 0 and 100 inclusive.
Steps:
• 0 <= n <= 100
Assumptions:
• The input integer n will always be a valid non-negative integer.
• Input: n = 6
• Explanation: Following the given rules, the array nums is generated as [0, 1, 1, 2, 1, 3, 2], and the maximum value in the array is 3.
Approach: To solve this problem, generate the array nums using the rules provided and track the maximum value while populating the array.
Observations:
• We need to iterate through the array and fill in the values based on specific conditions.
• We will need to calculate and compare the values as we go.
• The problem can be solved in linear time by iterating once through the array and maintaining the maximum value.
Steps:
• Create an array nums of length n + 1.
• Initialize the first two elements nums[0] = 0 and nums[1] = 1.
• Iterate through the array and fill in the values according to the rules provided for nums[2 * i] and nums[2 * i + 1].
• Track the maximum value as the array is populated.
Empty Inputs:
• If n = 0, the array will only contain one element, which is 0.
Large Inputs:
• For the maximum value of n = 100, the solution should handle the array efficiently.
Special Values:
• When n = 1, the array will be [0, 1], and the maximum will be 1.
Constraints:
• Ensure that the array size does not exceed the constraints given (n <= 100).
class Solution {
public int getMaximumGenerated(int n) {
if (n == 0) return 0;
int[] nums = new int[n + 1];
nums[0] = 0;
nums[1] = 1;
int max = 1;
for(int i = 1; 2 * i + 1 < n + 1; i++) {
nums[2 * i] = nums[i];
max = Math.max(nums[2 * i], max);
nums[2 * i + 1] = nums[i] + nums[i + 1];
max = Math.max(nums[2 * i + 1], max);
}
return max;
}
1 : Class Definition
class Solution {
This defines a class named `Solution`, which contains the method to compute the maximum value generated by the sequence.
2 : Method Definition
public int getMaximumGenerated(int n) {
This line defines the method `getMaximumGenerated` that takes an integer `n` as input and returns the maximum value in the generated sequence.
3 : Base Case Check
if (n == 0) return 0;
If `n` is 0, the function returns 0 because the generated sequence has no elements.
4 : Array Initialization
int[] nums = new int[n + 1];
An array `nums` is initialized with `n+1` elements to store the generated sequence.
5 : Base Case Assignment
nums[0] = 0;
The first element of the sequence is set to 0, as per the problem definition.
6 : Base Case Assignment
nums[1] = 1;
The second element of the sequence is set to 1, as per the problem definition.
7 : Variable Initialization
int max = 1;
The variable `max` is initialized to 1, which will track the maximum value encountered in the sequence.
8 : Loop
for(int i = 1; 2 * i + 1 < n + 1; i++) {
A loop starts to iterate from index 1 to generate the sequence values until `2*i + 1` exceeds `n`.
9 : Sequence Generation
nums[2 * i] = nums[i];
The element at index `2*i` is assigned the value of `nums[i]`, following the pattern defined in the problem.
10 : Max Update
max = Math.max(nums[2 * i], max);
The maximum value is updated if the value at `nums[2*i]` is greater than the current `max`.
11 : Sequence Generation
nums[2 * i + 1] = nums[i] + nums[i + 1];
The element at index `2*i + 1` is assigned the sum of `nums[i]` and `nums[i + 1]`, as per the pattern.
12 : Max Update
max = Math.max(nums[2 * i + 1], max);
The maximum value is updated again if the value at `nums[2*i + 1]` is greater than the current `max`.
13 : Return Statement
return max;
The method returns the maximum value found in the sequence.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The solution iterates through the array once to generate the values and track the maximum.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) due to the storage of the nums array.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus